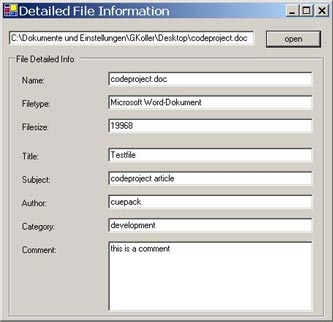
Introduction
This article shows, how one omitted the detail information of a file under the use of the Shell32.dll from the Windows system. Some detailed information are: Comment, Title, Subject, Category, Author, and so on.
Background
The Windows system could store additional information like author, comment, title, category... to a file, this information can be read with GetDetailsOf
method from the shell32.dll.
Using the code
First of all, add a new COM reference "Microsoft Shell Controls and Automation" (shell32.dll) to your Project.
After adding the reference, simple create an instance of the CFileInfo
class as in the demo project. To access the File Information, simply read the properties from the class.
try{
CFileInfo oDetailedFileInfo = new CFileInfo(sFileName);
txtName.Text = oDetailedFileInfo.FileName;
txtFiletype.Text = oDetailedFileInfo.FileType;
txtFileSize.Text = oDetailedFileInfo.FileSize.ToString();
txtAuthor.Text = oDetailedFileInfo.FileAuthor;
txtCategory.Text = oDetailedFileInfo.FileCategory;
txtComment.Text = oDetailedFileInfo.FileComment;
txtSubject.Text = oDetailedFileInfo.FileSubject;
txtTitle.Text = oDetailedFileInfo.FileTitle;
}catch(Exception ex){
MessageBox.Show("Could not read File information\r\n"
+ ex.Message, "Error while getting Info",
MessageBoxButtons.OK,MessageBoxIcon.Error);
}
Behind the Scenes
The GetDetailedFileInfo
method gets all available information of a file into an ArrayList
. The DetailedFileInfo
class is a helper class for holding the information in a simple object within the ArrayList
.
private ArrayList GetDetailedFileInfo(string sFile){
ArrayList aReturn = new ArrayList();
if(sFile.Length>0){
try{
ShellClass sh = new ShellClass();
Folder dir = sh.NameSpace( Path.GetDirectoryName( sFile ) );
FolderItem item = dir.ParseName( Path.GetFileName( sFile ) );
for( int i = 0; i < 30; i++ ) {
string det = dir.GetDetailsOf( item, i );
DetailedFileInfo oFileInfo = new DetailedFileInfo(i,det);
aReturn.Add(oFileInfo);
}
}catch(Exception){
}
}
return aReturn;
}
...
public class DetailedFileInfo{
int iID = 0;
string sValue ="";
public int ID{
get{return iID;}
set{iID=value;}
}
public string Value{
get{return sValue;}
set{sValue = value;}
}
public DetailedFileInfo(int ID, string Value){
iID = ID;
sValue = Value;
}
}
History
- First release - 15th August 2004.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.