Introduction
There are a lot of color picker controls for Windows form app. This tip describes how to use an in-line color picker control that allows you to more easily select a color in MenuStrip
or ContextMenuStrip
. This control is called ToolStripInlineColorPicker
control. The ToolStripInlineColorPicker
class provides a color picker of color tile style like below:
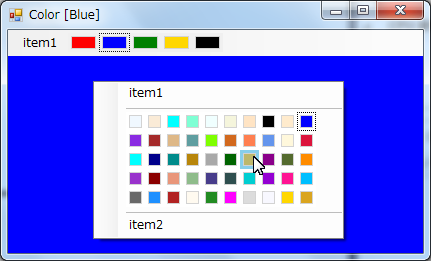
Reference of ToolStripInlineColorPicker Class
The ToolStripInlineColorPicker
class has inherited the ToolStripControlHost
class that hosts the color tile panel control (ColorTilePanel
class).
Constructors
Name | Description |
ToolStripInlineColorPicker() | Initializes a new instance of the ToolStripInlineColorPicker class. |
Properties
Name | Description |
CellHeight | Gets or sets the height in pixels of a color cell |
CellPadding | Gets or sets the internal spacing, in pixels, between the color cells and its edges |
CellWidth | Gets or sets the width in pixels of a color cell |
ColorList | Gets or sets the list of colors that is displayed on the color tile panel |
ColorPanel | Gets the color tile panel (ColorTilePanel class) in ToolStripInlineColorPicker class |
Columns | Gets or sets the number of columns in the color tile panel |
CurrentColor | Gets or sets the current color |
Name | Gets or sets the name of the item (Inherited from ToolStripControlHost ) |
Padding | Gets or sets the internal spacing, in pixels, between the item's contents and its edges. (Inherited from ToolStripControlHost .) |
Rows | Gets or sets the number of rows in the color tile panel. |
Events
Name | Description |
ColorSelected | Occurs when the mouse pointer is over the color cell and a mouse left button is pressed. (Change CurrentColor property) |
How to Use the Class
Case 1. Use in MenuStrip
Use the Designer. Adding the MenuStrip control to your form. then, add InlineColorPicker
to the menu item.

Using the properties window, set the properties and event handlers. Event handler that must be implemented is ColorSelected
event handler only.
void colorPicker_ColorSelected(object sender, ColorSelectedEventArgs e)
{
currentColor = e.SelectedColor;
contextMenuStrip1.Close();
var item = menuStrip1.Items.Find("toolStripInlineColorPicker1", false);
(item[0] as ToolStripInlineColorPicker).CurrentColor = currentColor;
Text = currentColor.ToString();
Invalidate();
}
Case 2. Use in ContextMenuStrip
First, create the ContextMenuStrip
control. Adding the ToolStripInlineColorPicker
class. Then, set the properties and event handlers in code.
private void CreateContextMenuStrip()
{
contextMenuStrip1.Items.Add(new ToolStripMenuItem() { Text = "item1" });
contextMenuStrip1.Items.Add(new ToolStripSeparator());
var colorPicker = new ToolStripInlineColorPicker()
{
Name = "colorPicker",
BackColor = Color.White,
ColorList = colorList,
CurrentColor = currentColor,
Columns = 10,
Rows = 5
};
colorPicker.ColorSelected += colorPicker_ColorSelected;
contextMenuStrip1.Items.Add(colorPicker);
contextMenuStrip1.Items.Add(new ToolStripSeparator());
contextMenuStrip1.Items.Add(new ToolStripMenuItem() { Text = "item2" });
}
Next, implement event handlers.
private void contextMenuStrip1_Opening(object sender, CancelEventArgs e)
{
var item = contextMenuStrip1.Items.Find("colorPicker", false);
(item[0] as ToolStripInlineColorPicker).CurrentColor = currentColor;
}
void colorPicker_ColorSelected(object sender, ColorSelectedEventArgs e)
{
currentColor = e.SelectedColor;
contextMenuStrip1.Close();
var item = menuStrip1.Items.Find("toolStripInlineColorPicker1", false);
(item[0] as ToolStripInlineColorPicker).CurrentColor = currentColor;
Text = currentColor.ToString();
Invalidate();
}
That's all. It's very easy to use.
Known Issues of the Class
In the case of the ContextMenuStrip
control, you can't access properties and events of the ToolStripInlineColorPicker
class in designer.
The demo project uses VS 2015.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.