Introduction
This article demonstrates an easy way to add a Combo box to a docking tool bar. I was needed to add a edit control to the toolbar for my dBase Explorer project (visit my web site for detail information: http://www.codearchive.com/~dbase/). Although, I found some of the articles on it in the Code Project site, most of them are difficult to implement, and you need to add a lot of code or even a new class. This article shows how you can add a combo box to a toolbar only by adding a few lines of code.
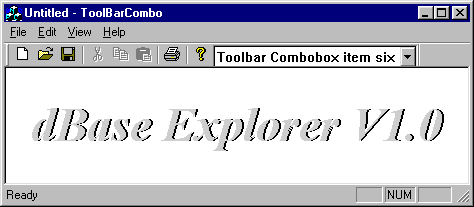
In order to add a combo box to a toolbar, you need to declare a member variable type CComboBox
to the CMainFrame
class as shown below:
class CMainFrame : public CFrameWnd
{
protected:
CMainFrame();
DECLARE_DYNCREATE(CMainFrame)
protected:
CStatusBar m_wndStatusBar;
CToolBar m_wndToolBar;
CComboBox m_comboBox;
...
};
You also need to create a place holder icon in the toolbar for the combo box. An Id should be assigned to the place holder icon by double clicking on it, for example, in my case ID_COMBO
was assigned to the place holder. Then by calling the Create
function, you create the combo box in the toolbar as shown below:
if(!m_comboBox.Create(CBS_DROPDOWNLIST | CBS_SORT | WS_VISIBLE |
WS_TABSTOP | WS_VSCROLL, rect, &m_wndToolBar, ID_COMBO))
{
TRACE(_T("Failed to create combo-box\n"));
return FALSE;
}
The complete listing of the OnCreate
function is given below:
int CMainFrame::OnCreate(LPCREATESTRUCT lpCreateStruct)
{
if (CFrameWnd::OnCreate(lpCreateStruct) == -1)
return -1;
if (!m_wndToolBar.CreateEx(this, TBSTYLE_FLAT, WS_CHILD | WS_VISIBLE | CBRS_TOP
| CBRS_GRIPPER | CBRS_TOOLTIPS | CBRS_FLYBY | CBRS_SIZE_DYNAMIC) ||
!m_wndToolBar.LoadToolBar(IDR_MAINFRAME))
{
TRACE0("Failed to create toolbar\n");
return -1;
}
if (!m_wndStatusBar.Create(this) ||
!m_wndStatusBar.SetIndicators(indicators,
sizeof(indicators)/sizeof(UINT)))
{
TRACE0("Failed to create status bar\n");
return -1;
}
CRect rect;
int nIndex = m_wndToolBar.GetToolBarCtrl().CommandToIndex(ID_COMBO);
m_wndToolBar.SetButtonInfo(nIndex, ID_COMBO, TBBS_SEPARATOR, 205);
m_wndToolBar.GetToolBarCtrl().GetItemRect(nIndex, &rect);
rect.top = 1;
rect.bottom = rect.top + 250 ;
if(!m_comboBox.Create(CBS_DROPDOWNLIST | CBS_SORT | WS_VISIBLE |
WS_TABSTOP | WS_VSCROLL, rect, &m_wndToolBar, ID_COMBO))
{
TRACE(_T("Failed to create combo-box\n"));
return FALSE;
}
m_comboBox.AddString("Toolbar Combobox item one");
m_comboBox.AddString("Toolbar Combobox item two");
m_comboBox.AddString("Toolbar Combobox item three");
m_comboBox.AddString("Toolbar Combobox item four");
m_comboBox.AddString("Toolbar Combobox item five");
m_comboBox.AddString("Toolbar Combobox item six");
m_wndToolBar.EnableDocking(CBRS_ALIGN_ANY);
EnableDocking(CBRS_ALIGN_ANY);
DockControlBar(&m_wndToolBar);
return 0;
}
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.