Introduction
Recently, I was looking for a way in which to use Dependency Injection Container in WCF service.
For my MVC projects, I usually use Unity, but I wasn’t able to make it work for WCF service, so I was looking for an alternative and I found it is very easy to use Ninject.
This article should illustrate how to setup WCF service and make it work with Ninject DIC.
WCF Service
Start by creating the WCF service.
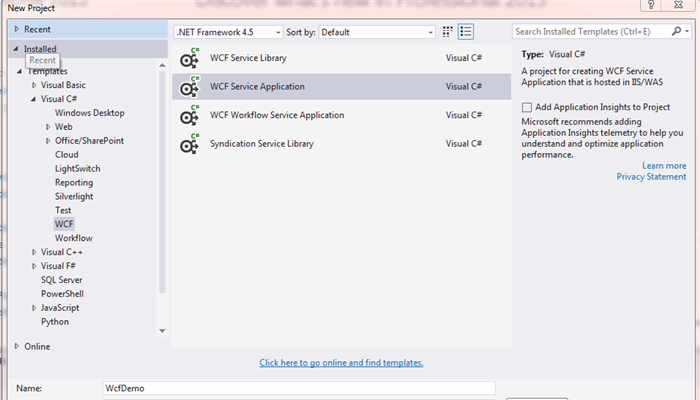
Setup Ninject
Next step is to install Ninject NuGet packages:


At this point, the structure of your project should look like this:

IDataReader
is my custom object that I call from Service1
and it is actually the interface that we need to inject into Service1
constructor. DataReader
is implementation of this interface.
Service1
[ServiceContract]
public interface IService1
{
[OperationContract]
string GetData(int value);
}
public class Service1 : IService1
{
IDataReader _reader;
public Service1(IDataReader reader)
{
_reader = reader;
}
public string GetData(int value)
{
return _reader.ReadData(value);
}
}
IDataReader and DataReader
public interface IDataReader
{
string ReadData(int value);
}
public class DataReader : IDataReader
{
public string ReadData(int value)
{
return string.Format("Data for value: {0} is {1}", value, new Guid());
}
}
In order to inject the interface into constructor, you need to register it in Ninject container like this:
private static void RegisterServices(IKernel kernel)
{
kernel.Bind<IDataReader>().To<DataReader>();
}
Now, if you would run the application, you would get this error:

The problem is that you’ve just set up the container, but you didn’t wire it into the application.
Wire the Ninject into the Application
You need to change the Service settings.
You can do this by markup:

and you need to change the code accordingly:
<%@ ServiceHost Language="C#" Debug="true"
Service="WcfDemo.Service1" CodeBehind="Service1.svc.cs" %>
<%@ ServiceHost Language="C#"
Debug="true" Service="WcfDemo.Service1"
CodeBehind="Service1.svc.cs"
Factory="Ninject.Extensions.Wcf.NinjectServiceHostFactory" %>
Summary
I think it is very easy to set up Ninject DIC into WCF service and it can bring you a lot of benefits to have DIC in your application.
You can download the entire working project here.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.