Introduction
Many of us now know the seamless power of AjaxControlToolkit features that aids to programmability and efficiency of our Applications. However in this article I have tried to exploit the AutoComplete extender a bit to create a generic WebMethod for retrieving auto completion lists. Here I will show how to make use of the context key attribute of the extender to create a single method that can be used with multiple AutoCompleteExtenders, differentiated only by the specific context keys that are supplied at runtime to each extender as and when needed.
Using the code
For this project to run you will need to add the reference to AjaxControlToolkit.dll to your project, there are numerous tutorials to guide you how to add AjaxControlToolkit to your project.
Assuming you have added the reference, let’s do the coding...
Step 1: Create a new Website and add a WebService file to it..
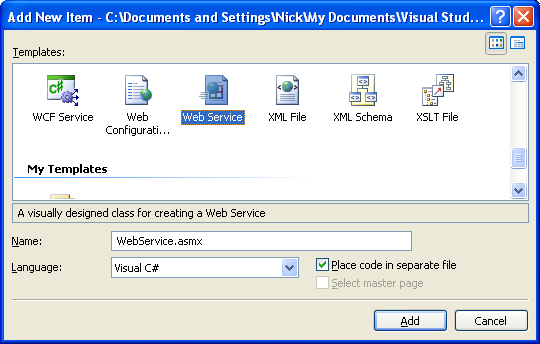
There’s no need to change anything in the asmx file, just go to webservice.cs file as well as step 2
Step 2: Find and uncomment the line
Somewhere and some-distance below it you will find a method (actually a WebMethod) as shown below
[WebMethod]
public string HelloWorld() {
return "Hello World";
}
Delete it clear(Or keep it if you are too keen to have it).. and create a new method with any name you fancy but keep the parameters absolutely the same as shown below including letter cases..
[WebMethod]
[System.Web.Script.Services.ScriptMethod()]
public string[] GetCompletionList(string prefixText, int count, string contextKey)
{
}
Step 3: Here comes a bit of imagination...
Imagine you have a string inside the GetCompletionList function that contains the information about from which table and from which one of its column you want to fetch the completion list in “tablename#columnname” format. Now suppose this string came from the contextKey parameter.This string can be used to write a parametrized sql query that fetches the data for us.
string query = "select " + contextKey.Split('#').ElementAt(1) + " from " +
contextKey.Split('#').ElementAt(0) + " where " +
contextKey.Split('#').ElementAt(1) + " like @prefixtext";
If for instance the contextKey value is “tbl_Contacts#ContactName” and
prefixText is “nick”, then the
query becomes
select ContactName from tbl_Contacts where ContactName like %nick% ;
and when executed will return all datavalues under ContactName column that contains the string nick
Fill a DataSet
using an SqlDataAdapter
and an SqlCommand
with its CommandText
= query
String cnString = System.Configuration.ConfigurationManager.ConnectionStrings["cnstring"].ConnectionString;
string query = "select " + contextKey.Split('#').ElementAt(1) + " from " + contextKey.Split('#').ElementAt(0) + " where " + contextKey.Split('#').ElementAt(1) + " like @prefixtext";
SqlConnection con = new SqlConnection(cnString);
SqlCommand cmd = new SqlCommand(query, con);
cmd.Parameters.Add("@prefixtext", SqlDbType.NVarChar).Value = "%" + prefixText + "%";
DataSet ds = new DataSet();
SqlDataAdapter da = new SqlDataAdapter(cmd);
try
{
da.Fill(ds, "completionListTable");
}
catch(Exception ex) { }
After the try block is executed without exception you are suppossed to get a table with single column containing all the strings containing the prefixText as rows.
Step 3: Query this table using linq or any other way you like, to retrieve a string array of suggested strings and return it..
DataTable CompletionListTable = ds.Tables["completionListTable"];
var list = from row in CompletionListTable.Select()
select row[0].ToString();
return list.ToArray();
So Our WebMethod is Complete now come over to the aspx page where the AutoCompleteExtender is to be used..
Step 4: Register the AjaxControlToolkit assembly..
Add a ToolkitScriptManager on the page
<asp:ToolkitScriptManager ID="scriptman" runat="server"></asp:ToolkitScriptManager>
Create two textboxes on the aspx page and two corresponding AutoCompleteExtenders..
<div>
<asp:ToolkitScriptManager ID="scriptman" runat="server"></asp:ToolkitScriptManager>
Categories:
<asp:TextBox ID="TextBox1" runat="server"></asp:TextBox>
<asp:AutoCompleteExtender ID="AutoCompleteExtender1" runat="server"
TargetControlID="TextBox1"
ServiceMethod="GetCompletionList"
ServicePath="~/WebService.asmx"
UseContextKey="true"
ContextKey=""
MinimumPrefixLength="1"></asp:AutoCompleteExtender>
Suppliers:
<asp:TextBox ID="TextBox2" runat="server"></asp:TextBox>
<asp:AutoCompleteExtender ID="AutoCompleteExtender2" runat="server"
TargetControlID="TextBox2"
ServiceMethod="GetCompletionList"
ServicePath="~/WebService.asmx"
UseContextKey="true"
ContextKey=""
MinimumPrefixLength="1"></asp:AutoCompleteExtender>
</div>
As you can see both are using same ServicePath
and ServiceMethod
but they will be assigned different ContextKeys
in the code-behind page as shown below…
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
AutoCompleteExtender1.ContextKey = "Categories#CategoryName";
AutoCompleteExtender2.ContextKey = "Suppliers#ContactName";
}
}
Note: I have used the Northwind databases’ Categories and Suppliers table..
Step 5: Run the Website
Below is the screen shots of the website when above codes are in action...


Like this you can use the same WebMethod in any number of AutoCompleteExtenders throughout your project without writing separate functions for each instance..