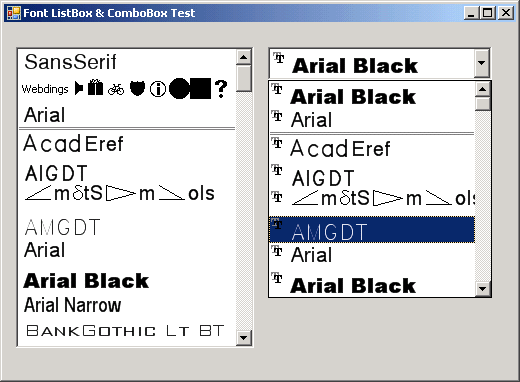
Introduction
This control replicates the Office Font drop down box, with the feature of having your top five recently selected fonts.
Background
I started this control a while back as a ListBox control and after overcoming several problems I converted it to a ComboBox by copying most of the code in one go in a few minutes. Two controls for the price of one!
Using the code
There is only one property that really needs setting and that's the Image
setting. This image is the TT, TrueType image included in the package - this is actually a fudge because I didn't have the time (read as: couldn't be bothered) to get the Resource Manager to work nicely in VS 2003, but in VS 2005 it's simple. You've also got the MaximumFavourites
property which sets how many fonts you are going to show in the Favourite section, set at a default of 5. And a NonReadableFonts
which is just a list of fonts found on my PC that don't display readable text, add yours as you go along.
fontListBox1.MaximumFavourites = 5;
fontListBox1.NonReadableFonts = new string[] {
"Webdings",
"Wingdings",
"Wingdings 2",
"Wingdings 3"};
Points of Interest
Damn this code was tricky, trying to get the favourite feature to work nicely, but when the control was finished I looked back at it and it seems pretty simple, just a case of understanding the logic.
I have a collection which has a list of the favourites which is updated in the OnSelectedIndexChanged
event. It sees if the newly selected font is in the Favourites, if it is then moves it to the top of the list, if it isn't then adds it, removing other favourite fonts if the count is over the maximum limit.
protected override void OnSelectedIndexChanged(EventArgs e)
{
base.OnSelectedIndexChanged(e);
string fontName = this.Text;
if (fontName == "") { return; }
int indexOf = favourites.IndexOf(fontName);
if (indexOf == -1)
{
if (maxFavourites > favourites.Count)
{
favourites.Insert(0, fontName);
this.Items.Insert(0, fontName);
}
else
{
favourites.RemoveAt(maxFavourites - 1);
favourites.Insert(0, fontName);
this.Items.RemoveAt(maxFavourites - 1);
this.Items.Insert(0, fontName);
}
}
else
{
if (favourites.Count > 1)
{
favourites.RemoveAt(indexOf);
favourites.Insert(0, fontName);
this.Items.RemoveAt(indexOf);
this.Items.Insert(0, fontName);
}
}
this.EndUpdate();
}
If the font is unreadable then I use Tahoma as a default font to write the name and then try and write the name of the font in it's actual font type, usually displaying images.
The ItemHeight
in the ListBox or ComboBox has a 3 added to the value to allow for drawing the Favourite separator, otherwise the text would be clipped at the bottom.
Known Issues
Due to the designer always getting the fonts at design time, the Items
collection would grow and grow and grow! So I put in a hack to get the Design Mode to work in this instance (as I am not using ComponentModel
, the design mode won't work) and not generate the font list until the control is actually running in runtime.
Selecting a font that is added to the Favourite section means that the selection frame isn't quite right. Also selecting a font will not return you to the top of the list.
History
- Uploaded Visual Studio 2005 Beta 2 - 17 June 2005.
- Uploaded Visual Studio 2003 - 17 June 2005.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.