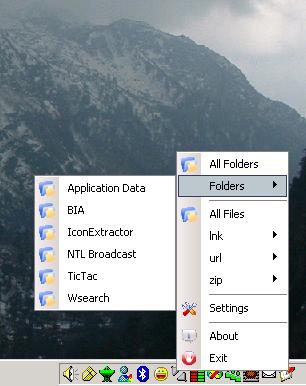
Introduction
The sole purpose of this application is to get rid of that messy desktop. All that this application does is cleans your desktop. It does this by arranging all files of the same type together, so that they are easily accessible from the system tray.
This app is my first experiment with Visual Basic 2005 Express edition... You need to have .NET 2.0 Framework installed in your system to run this app.
The working
Here goes the working... When the application executes, first it takes the backup of your desktop date-wise (in case you want to restore it later on). It then reads the files and folders on the desktop one by one and moves them to appropriate folders (according to there extensions). A menu is generated dynamically which lists the files and folders neatly arranged, in the system tray. You can now access any of the files or folders with ease.
Note: Make sure you run the application from a location other than the desktop... otherwise it will move itself.
The code
VB.NET 2005 made it so simple....With MY
, we can have access to so many things easily. I could access the desktop of my machine without even worrying about the version of Windows I am working on. Similarly moving, deleting, copying of files and directories were done by just one line of code. Check it out yourself:
Imports System.IO
Public Class Form1
Private Declare Function ShellExecute Lib "shell32.dll" _
Alias "ShellExecuteA"(ByVal hwnd As Integer, _
ByVal lpOperation As String, ByVal lpFile As String, _
ByVal lpParameters As String, ByVal lpDirectory As String, _
ByVal nShowCmd As Integer) As Integer
Dim desktoppath As String = _
My.Computer.FileSystem.SpecialDirectories.Desktop.ToString()
Dim destdir As String = _
My.Application.Info.DirectoryPath & "\DesktopCleaned\"
Dim backupdir As String = _
My.Application.Info.DirectoryPath & "\DesktopBackup_" & _
DateTime.Now.Day & DateTime.Now.Month & DateTime.Now.Year & "\"
Private Sub Form1_Load(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles MyBase.Load
Dim files As _
System.Collections.ObjectModel.ReadOnlyCollection(Of String)
Dim listdirs As _
System.Collections.ObjectModel.ReadOnlyCollection(Of String)
Dim i As Integer
Dim filext As String
Dim filename As String
Dim dirname As String
Dim lstindex As Integer = 0
My.Application.SaveMySettingsOnExit = True
On Error GoTo classhandler
classhandler:
If Err.Number = 57 Then
systray.BalloonTipIcon = ToolTipIcon.Warning
systray.BalloonTipTitle = "Warning"
systray.BalloonTipText = "Some files are in use. Please " & _
"close them and re-run the application"
systray.ShowBalloonTip(2)
Else
End If
Resume Next
files = My.Computer.FileSystem.GetFiles(desktoppath, True, "*.*")
listdirs = My.Computer.FileSystem.GetDirectories(desktoppath, _
FileIO.SearchOption.SearchTopLevelOnly, "*.*")
If Not My.Computer.FileSystem.DirectoryExists(destdir) Then
My.Computer.FileSystem.CreateDirectory(destdir)
End If
If Not My.Computer.FileSystem.DirectoryExists(destdir & _
"Folders") Then
My.Computer.FileSystem.CreateDirectory(destdir & "Folders")
End If
systray.BalloonTipIcon = ToolTipIcon.Info
systray.BalloonTipTitle = "Backup"
systray.BalloonTipText = "Backing up folders...Please wait"
systray.ShowBalloonTip(1)
For i = 0 To listdirs.Count - 1
dirname = listdirs(i)
lstindex = dirname.LastIndexOf("\")
dirname = dirname.Substring(lstindex + 1, _
dirname.Length - lstindex - 1)
If Not My.Computer.FileSystem.DirectoryExists(backupdir & _
dirname) Then
My.Computer.FileSystem.CopyDirectory(desktoppath & _
"\" & dirname, backupdir & dirname)
Else
My.Computer.FileSystem.CopyDirectory(desktoppath & _
"\" & dirname, backupdir & "Renamed_" & _
DateTime.Now.Hour.ToString() & _
DateTime.Now.Minute.ToString() & _
DateTime.Now.Second.ToString() & "_" & dirname)
End If
Next
systray.BalloonTipIcon = ToolTipIcon.Info
systray.BalloonTipTitle = "Backup"
systray.BalloonTipText = "Backing up files...Please wait"
systray.ShowBalloonTip(1)
For i = 0 To files.Count - 1
filename = files(i)
lstindex = filename.LastIndexOf("\")
filename = filename.Substring(lstindex + 1, _
filename.Length - lstindex - 1)
lstindex = filename.LastIndexOf(".")
If lstindex <> -1 Then
If My.Computer.FileSystem.FileExists(backupdir & _
filename) Then
My.Computer.FileSystem.CopyFile(desktoppath & _
"\" & filename, backupdir & "Renamed_" & _
DateTime.Now.Hour.ToString() & _
DateTime.Now.Minute.ToString() & _
DateTime.Now.Second.ToString() & _
"_" & filename)
Else
My.Computer.FileSystem.CopyFile(desktoppath & _
"\" & filename, _
backupdir & filename)
End If
End If
Next
For i = 0 To listdirs.Count - 1
dirname = listdirs(i)
lstindex = dirname.LastIndexOf("\")
dirname = dirname.Substring(lstindex + 1, _
dirname.Length - lstindex - 1)
If Not My.Computer.FileSystem.DirectoryExists(destdir & _
"\" & "\Folders\" & dirname) Then
My.Computer.FileSystem.MoveDirectory(desktoppath & _
"\" & dirname, destdir & "\Folders\" & dirname)
Else
My.Computer.FileSystem.MoveDirectory(desktoppath & _
"\" & dirname, destdir & "\Folders\" & _
"Renamed_" & DateTime.Now.Hour.ToString() & _
DateTime.Now.Minute.ToString() & _
DateTime.Now.Second.ToString() & _
"_" & dirname)
End If
Next
For i = 0 To files.Count - 1
filename = files(i)
lstindex = filename.LastIndexOf("\")
filename = filename.Substring(lstindex + 1, _
filename.Length - lstindex - 1)
lstindex = filename.LastIndexOf(".")
If lstindex <> -1 Then
filext = filename.Substring(lstindex + 1, _
filename.Length - lstindex - 1)
If Not My.Computer.FileSystem.DirectoryExists(destdir & _
"\" & filext) Then
My.Computer.FileSystem.CreateDirectory(destdir & _
"\" & filext)
End If
If My.Computer.FileSystem.FileExists(destdir & _
"\" & filext & "\" & filename) Then
My.Computer.FileSystem.MoveFile(desktoppath & _
"\" & filename, destdir & _
filext & "\" & _
"Renamed_" & DateTime.Now.Hour.ToString() & _
DateTime.Now.Minute.ToString() & _
DateTime.Now.Second.ToString() & _
"_" & filename)
Else
My.Computer.FileSystem.MoveFile(desktoppath & _
"\" & filename, destdir & filext & _
"\" & filename)
End If
Else
filext = ""
End If
Next
systray.BalloonTipIcon = ToolTipIcon.Info
systray.BalloonTipTitle = "Cleaning"
systray.BalloonTipText = "Cleaning Desktop...Done"
systray.ShowBalloonTip(1)
Call genmenu()
End Sub
Sub genmenu()
Dim i As Integer
Dim j As Integer
Dim dirname As String
Dim dirnameinside As String
Dim filename As String
Dim lstindex As Integer = 0
Dim getdirs As _
System.Collections.ObjectModel.ReadOnlyCollection(Of String)
Dim getdirsinside As _
System.Collections.ObjectModel.ReadOnlyCollection(Of String)
Dim getfiles As _
System.Collections.ObjectModel.ReadOnlyCollection(Of String)
Dim parentMenu As ToolStripMenuItem
Dim ChildMenu As ToolStripMenuItem
Dim IconExtractor As IconExtractor
Dim Icon As System.Drawing.Icon
Dim File As String
Dim sep As ToolStripSeparator
Dim defalutmenu As ToolStripItem
rmenu.Items.Clear()
IconExtractor = New IconExtractor
If My.Computer.FileSystem.DirectoryExists(destdir & _
"\Folders") Then
getdirsinside = _
My.Computer.FileSystem.GetDirectories(destdir & "\Folders")
If getdirsinside.Count > 0 Then
defalutmenu = New ToolStripMenuItem()
defalutmenu.Name = "Folders"
defalutmenu.Text = "All Folders"
defalutmenu.ForeColor = Color.Aqua
rmenu.Items.Add(defalutmenu.ToString(), P2.Image)
parentMenu = New ToolStripMenuItem()
parentMenu.Name = "folders"
parentMenu.Text = "Folders"
rmenu.Items.Add(parentMenu)
For j = 0 To getdirsinside.Count - 1
lstindex = getdirsinside(j).LastIndexOf("\")
dirnameinside = getdirsinside(j).Substring(lstindex + 1, _
getdirsinside(j).Length - lstindex - 1)
ChildMenu = parentMenu.DropDown.Items.Add(dirnameinside, _
P2.Image, AddressOf MenuItem_Click)
ChildMenu.Tag = getdirsinside(j)
Next
sep = New ToolStripSeparator
sep.Name = "sep2"
rmenu.Items.Add(sep)
End If
End If
defalutmenu = New ToolStripMenuItem()
defalutmenu.Name = "files"
defalutmenu.Text = "All Files"
defalutmenu.ForeColor = Color.Aqua
rmenu.Items.Add(defalutmenu.ToString(), P2.Image)
getdirs = My.Computer.FileSystem.GetDirectories(destdir)
For i = 0 To getdirs.Count - 1
dirname = getdirs(i)
lstindex = dirname.LastIndexOf("\")
dirname = dirname.Substring(lstindex + 1, _
dirname.Length - lstindex - 1)
parentMenu = New ToolStripMenuItem()
parentMenu.Name = dirname
parentMenu.Text = dirname
If dirname <> "Folders" Then
rmenu.Items.Add(parentMenu)
End If
getdirsinside = _
My.Computer.FileSystem.GetDirectories(destdir & _
"\" & dirname)
For j = 0 To getdirsinside.Count - 1
lstindex = getdirsinside(j).LastIndexOf("\")
dirnameinside = getdirsinside(j).Substring(lstindex + 1, _
getdirsinside(j).Length - lstindex - 1)
ChildMenu = parentMenu.DropDown.Items.Add(dirnameinside, _
P2.Image, AddressOf MenuItem_Click)
ChildMenu.Tag = getdirsinside(j)
Next
getfiles = My.Computer.FileSystem.GetFiles(destdir & _
"\" & dirname)
For j = 0 To getfiles.Count - 1
File = getfiles(j)
Icon = IconExtractor.Extract(File, IconSize.Small)
P1.Image = Icon.ToBitmap()
lstindex = getfiles(j).LastIndexOf("\")
filename = getfiles(j).Substring(lstindex + 1, _
getfiles(j).Length - lstindex - 1)
ChildMenu = parentMenu.DropDown.Items.Add(filename, _
P1.Image, AddressOf MenuItem_Click)
ChildMenu.Tag = getfiles(j)
Next
Next
sep = New ToolStripSeparator
sep.Name = "sep"
rmenu.Items.Add(sep)
defalutmenu = New ToolStripMenuItem()
defalutmenu.Name = "Settings"
defalutmenu.Text = "S&ettings"
rmenu.Items.Add(defalutmenu.ToString(), _
sets.Image, AddressOf settings_Click)
sep = New ToolStripSeparator
sep.Name = "sep2"
rmenu.Items.Add(sep)
defalutmenu = New ToolStripMenuItem()
defalutmenu.Name = "About"
defalutmenu.Text = "A&bout"
rmenu.Items.Add(defalutmenu.ToString(), _
abtp.Image, AddressOf about_Click)
defalutmenu = New ToolStripMenuItem()
defalutmenu.Name = "Exit"
defalutmenu.Text = "E&xit"
rmenu.Items.Add(defalutmenu.ToString(), _
exitp.Image, AddressOf exit_Click)
End Sub
Private Sub MenuItem_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs)
Dim tsmi As New ToolStripMenuItem
tsmi = CType(sender, ToolStripMenuItem)
ShellExecute(0, "open", tsmi.Tag.ToString(), "", "", 1)
End Sub
Private Sub settings_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs)
setting.Show()
End Sub
Private Sub about_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs)
about.show()
End Sub
Private Sub exit_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs)
systray.Visible = False
Me.Close()
End
End Sub
End Class
I love coding, gaming and .......... http://www.gauravcreations.com