LEADTOOLS Recognition Imaging SDK - I had the pleasure of taking the SDK for a spin, and let me tell you it was well worth it. LEADTOOLS has already established itself as the leader in Imaging SDKs, but for me it goes much further than just that. The technical support is stellar! This is what differentiates a good, functional product from a stunning product.
LEADTOOLS Recognition Imaging – Technical Support
Before I delve into the code, I have to highlight the LEADTOOLS technical support team. I had an issue I couldn’t resolve myself. I emailed LEAD's technical support and received a reply from them a few hours later. The support agent assigned to my case gave me several different solutions that I could try. This single email resolved my issue on the spot. This is where the watershed exists for many products. Great tools without great support is meaningless. LEADTOOLS surpassed my expectations and treated my support request with a complete and technically sound reply. I felt as if I had purchased the product (remember, I only have the trial version here).
LEADTOOLS Recogntion Imaging – Setting Up Your Code
I am using Visual Studio 2013 with a basic Windows Forms application. With LEADTOOLS, you can of course create web applications that harness the power of their Imaging SDK too. To start off, you will need to add the following using statements to your application.
using System;
using System.Diagnostics.Contracts;
using System.IO;
using System.Windows.Forms;
using Leadtools;
using Leadtools.Codecs;
using Leadtools.Forms;
using Leadtools.Forms.DocumentWriters;
using Leadtools.Forms.Ocr;
using Leadtools.Twain;
using Leadtools.Documents;
using Leadtools.Documents.UI;
Then in the constructor you need to set up the licensing for your SDK. To do this you need to define a path to your license file as well as define your developer key. You then call the LeadTools.RasterSupport
class which provides the methods for you to set your LEADTOOLS runtime license. This also unlocks support for optional LEADTOOLS features such as LEADTOOLS Document/Medical capabilities.
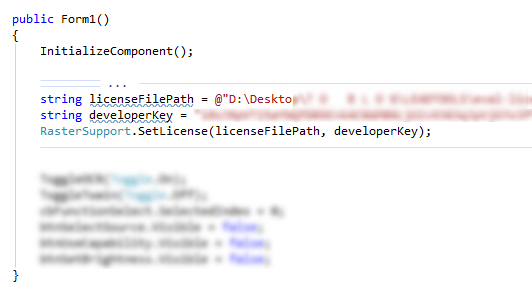
Converting a TIFF to a Searchable PDF Document
To see how easy it is to convert a TIFF to a text-searchable PDF document, I created the following app. All it does is read a TIFF file and processes that through the IOcrEngine. Clicking on the ‘Tiffs to PDF’ button will start to process the TIFF file.
private void LoadImage(string fileName)
{
Contract.Requires<FileNotFoundException>(File.Exists(fileName),
"The file does not exist at the given file location.");
try
{
using (RasterCodecs codec = new RasterCodecs())
{
rasterImageViewer1.Image = codec.Load(fileName);
}
}
catch (Exception ex)
{
MessageBoox.Show(ex.Message);
}
}
The TIFF file is then converted into a PDF document. I used the LEADTOOLS Document Viewer to display the created PDF document. You will notice that the Document Viewer also displays thumbnails of the generated PDF document.

When I open the newly created PDF document in Acrobat Reader, you can see that the PDF document is a fully valid and searchable PDF document.

The code to accomplish this is just as simple. In the MultiTiff()
method, I create an instance of the IOcrEngine. The engine is then started with default options by passing null as a parameter. Be sure to add a reference to the ‘Leadtools.Forms.Ocr.Advantage
’ DLL in your project. Then run the AutoRecognizeManager
passing it the path to the TIFF file and the file name of the PDF document you want to create.
private void MultiTiff()
{
using (IOcrEngine ocrEngine = OcrEngineManager.CreateEngine
(OcrEngineType.Advantage, false)
)
{
ocrEngine.Startup(null, null, null, null);
ocrEngine.AutoRecognizeManager.Run(
@"C:\temp\LEADTOOLS\tiffs\nda.tif",
@"C:\temp\LEADTOOLS\tiffs\TiffOutputt\nda.pdf",
DocumentFormat.Pdf,
null,
null);
}
}
In the button click event ‘Tiffs to PDF’, the following code is used to create a split container that will display the generated PDF document as well as the thumbnail container. I then loaded the created PDF document into my DocumentFactory
object. The result is a beautifully generated and displayed PDF document with minimal code.
private void btnProcessTiffs_Click(object sender, EventArgs e)
{
MultiTiff();
var splitContainer = new SplitContainer
{
Dock = DockStyle.Fill
};
this.Controls.Add(splitContainer);
var createOptions = new DocumentViewerCreateOptions()
{
ViewContainer = splitContainer.Panel2,
ThumbnailsContainer = splitContainer.Panel1
};
var documentViewer =
DocumentViewerFactory.CreateDocumentViewer
(createOptions);
var document =
DocumentFactory.LoadFromFile
(@"C:\temp\LEADTOOLS\tiffs\TiffOutput\nda.pdf",
new LoadDocumentsOptions { UseCache = false });
documentViewer.SetDocument(document);
documentViewer.View.PreferredItemType =
DocumentViewerItemType.Svg;
documentViewer.Commands.Run
(DocumentViewerCommands.InteractivePanZoom);
}
Consider the following application: reading multiple TIFF files and converting all these into PDF Documents. The use of this code logic is very powerful.
If you run into the following exception ‘Raster PDF Engine is needed to use this feature,’ you need to locate the Leadtools.PdfEngine.dll in the LEADTOOLS installation directory (usually located at ‘C:\LEADTOOLS 19\Bin’ in one of the Dotnet subfolders). Copy this DLL and paste it into the bin folder of your application, or add it to the project and set its Build Action to "Content" and Copy to Output Directory to "Copy Always." You can also copy the Leadtools.PdfEngine.dll to a common directory and use the InitialPath method to tell LEADTOOLS to locate and load the PDF Engine from the DLL at the given path. You can read more at the following support article ‘InitialPath Property.‘

Zonal OCR
You can also specify that LEADTOOLS only recognize a specific zone from a loaded TIFF file. I am using the Advantage OCR Engine here. The application also uses a split container with a LEADTOOLS RasterImageViewer control in the top panel, and a plain old text box in the bottom panel.

Clicking on the ‘Load Image’ button will load the TIFF file (the same TIFF I used as example above) and display it in the RasterImageViewer control. This is where you can now use your mouse and select a zone of text for the OCR engine to recognize. When you click on the ‘Read Zone’ button, the text is output to the text box in the bottom panel.

Here is a closer view of the selected zone and the text it recognized which was output to the text box. As you can see, this is quite accurate.

The code to accomplish this is also a walk in the park. In the ‘Load Image’ button logic all I am doing is setting the RasterImageViewer to the image loaded with the TIFF codec at the given path. My button click just called the method ‘LoadImage
’ and passed it the path to the TIFF file.
private void LoadImage(string fileName)
{
Contract.Requires<FileNotFoundException>(File.Exists(fileName),
"The file does not exist at the given file location.");
try
{
using (RasterCodecs codec = new RasterCodecs())
{
rasterImageViewer1.Image = codec.Load(fileName);
}
}
catch (Exception ex)
{
Message.Show(ex.Message);
}
}
The ‘ReadFileZone
’ method first checks that a zone is selected in the loaded TIFF file and then proceeds to create the Advantage OCR Engine. You will see that the engine starts up and a page is then created from the image in the RasterImageViewer. After that, the zone I selected with my mouse is then identified and recognized. The recognized text is then output to the text box in the split container. Easy peasy lemon squeezy.
private void ReadFileZone()
{
Contract.Requires<FormatException>(rasterImageViewer1.Image.HasRegion,
"Using your mouse, please select a zone in the viewer.");
try
{
using (IOcrEngine engine = OcrEngineManager.CreateEngine
(OcrEngineType.Advantage, false))
{
engine.Startup(null, null, null, null);
using (IOcrDocument doc =
engine.DocumentManager.CreateDocument())
{
IOcrPage page = doc.Pages.AddPage
(rasterImageViewer1.Image, null);
OcrZone zone = new OcrZone()
{
Bounds = new LogicalRectangle
(
rasterImageViewer1.Image.GetRegionBounds(null)),
ZoneType = OcrZoneType.Text
};
page.Zones.Add(zone);
page.Recognize(null);
txtEdit.Text = page.GetText(0);
}
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
Consider the following application: Recognizing text on a standard document by pre-selecting zones automatically and saving these zones to a data store such as a SQL Server database. You can then provide a digital copy of multiple forms that are searchable from the database in a snap.
Conclusion
The LEADTOOLS Recognition Imaging SDK is a very powerful set of tools for your .NET application requirements. I have only scratched the surface of what is possible with the SDK as it includes many additional features for document, medical and multimedia imaging, and also includes development interfaces for CDLL, C++ Class Libraries, HTML5/JavaScript, iOS/OS X, Android and more. I encourage you to have a look at their product line overview and download the trial to play with.
Disclosure of Material Connection: I received one or more of the products or services mentioned above for free in the hope that I would mention it on my blog. Regardless, I only recommend products or services I use personally and believe my readers will enjoy. I am disclosing this in accordance with the Federal Trade Commission’s 16 CFR, Part 255: “Guides Concerning the Use of Endorsements and Testimonials in Advertising.
With over 17 years of experience programming with C# and Visual Studio, I have worked for various companies throughout my career. I have been privileged to work with and learn from some of the most brilliant developers in the industry. I have authored several books on topics ranging from Visual Studio and C# to ASP.NET Core. I am passionate about writing code and love learning new tech and imparting what I know to others.