Introduction
This application will show a different way of how to navigate through records in a data source with a TrackBar
(Slider) by using either the mouse or the direction keys (Left, Right, Up, Down). When you move the slider, the trackbar sends notification messages to indicate the change. If you reach the end or beginning of the trackbar by moving the slider, you will be informed with a MessageBox
.
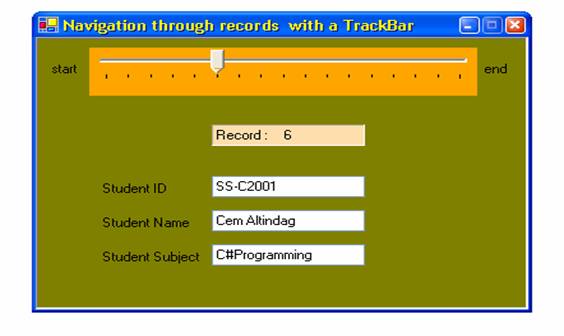
Code and How It Works
If the program is started, first the connection to the database and then the data binding for all the controls on the Form
will be established.
private void Form1_Load(object sender, System.EventArgs e)
{
fnGetDatabaseConnection();
fnGetDataBindings();
}
private void fnGetDatabaseConnection()
{
OleDbConnection con=new OleDbConnection(conString);
OleDbDataAdapter dadapter=new OleDbDataAdapter(selectString, con);
dataset=new DataSet();
dadapter.Fill(dataset, "studentTable");
}
Here is a brief introduction to DataBinding
and BindingContext
used in the method fnGetDataBindings()
.
Data Binding
Data binding is the ability to bind some elements of a data source with some graphical elements of an application. The data in Windows Forms is bound by calling DataBindings
. Windows Forms allow you to bind easily to almost any structure that contains data.
Windows Forms controls (i.e., TextBox
, etc.) support two types of data binding:
- Simple data binding
- Complex data binding
Simple data binding can be performed either at design time using DataBindings
property of a control or dynamically at run time. For example: a simple Windows Form displays data from a single table in a DataGrid
.
Complex data binding is useful if you bind a control to a list of values from a data source. The ComboBox
and ListBox
controls also support complex data binding.
For Example
textBox1.DataBindings.Add("Text", dataset, "studentTable.studentID");
The control textBox1
is bound to the studentID
column of a studentTable
table on the DataSet
(dataset
) through the BindingContext
object.
BindingContext
Every Windows Form has a BindingContext
object keeping track of all the CurrencyManager
objects on the Windows Form. CurrencyManager
keeps track of the position in the data source and keeps data-bound controls synchronized with each other. When you bind a data object to a control (i.e., TextBox
), a CurrencyManager
object is automatically assigned.
If you bind several controls to the same data source, they share the same CurrencyManager
.
For Example
- If you want to know how many records are in a data table, you simply query the
BindingContext
object's Count
property.
this.BindingContext[dataset,"studentTable"].Count - 1 ;
- If you want to get the current position from the
BindingContext
object:
this.BindingContext[dataset, "studentTable"].Position + 1;
Here we pass the table "studentTable
" to the BindingContext
as the bound control.
Now here is the method for data binding.
private void fnGetDataBindings()
{
this.label1.Text = "Record : " +
((this.BindingContext[dataset, "studentTable"].Position + 1).ToString());
this.textBox1.DataBindings.Add("Text", dataset,
"studentTable.studentID");
this.textBox2.DataBindings.Add("Text", dataset,
"studentTable.studentName");
this.textBox3.DataBindings.Add("Text", dataset,
"studentTable.studentSubject");
this.trackBar1.Maximum =
this.BindingContext[dataset,"studentTable"].Count - 1 ;
}
TrackBar(Slider)
There is only one point left to mention, namely the event handler for scrolling the TrackBar
(Slider) control. A TrackBar
is a window that has a thumb (slider) and optional tick marks. The thumb can be adjusted. Its position corresponds to the Value
property. When you move the slider, using either the mouse or the direction keys, the TrackBar
sends notification messages to indicate the change. TrackBar
can be aligned horizontally or vertically.
Scroll
event will occur when you move the slider either with a mouse or keyboard (Left, Right, Up, Down).
private void trackBar1_Scroll(object sender, System.EventArgs e)
{
int recordNr;
recordNr=this.BindingContext[dataset,"studentTable"].Position =
this.trackBar1.Value ;
++recordNr;
this.label1.Text = "Record : " + recordNr.ToString();
if ((this.BindingContext[dataset, "studentTable"].Position ) <
(this.BindingContext[dataset, "studentTable"].Count-1))
{
this.BindingContext[dataset,"studentTable"].Position +=1;
}
else
{
csShowMessageBox.fnShowMessageBoxWithParameters("You've " +
"reached the end of the records",
"LAST RECORD: "+recordNr.ToString(),
MessageBoxButtons.OK,
MessageBoxIcon.Information,0,0);
}
if (this.BindingContext[dataset, "studentTable"].Position ==1)
{
csShowMessageBox.fnShowMessageBoxWithParameters("You've reached" +
" the beginning of the records",
"FIRST RECORD: "+recordNr.ToString(),
MessageBoxButtons.OK,
MessageBoxIcon.Information,0,0);
}
}
In Conclusion
I designed this program to show a different way of how to navigate through records in a data table with a TrackBar
(Slider). I think and hope that I've showed some interesting tips which could help you maybe in some cases. Good coding.
History
- 8th July, 2005: Initial version
License
This article has no explicit license attached to it, but may contain usage terms in the article text or the download files themselves. If in doubt, please contact the author via the discussion board below. A list of licenses authors might use can be found here.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.