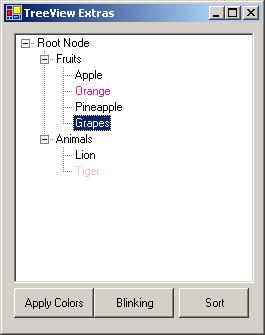
Introduction
This is my first article posting in this site. The code snippets illustrated here will be help to customize your TreeView
control in VB.NET. Here, I have tried out two things that can be done when selecting a node - first to blink the node with the help of a Timer
control, and second, sort the node with its subtree nodes.
Concept
While we are working with most VB.NET controls, it is very easy to provide a good and easy-to-use presentation. But if we are working on a control like TreeView
, we may face some difficulties. To overcome those issues, these code snippets will be helpful in a big way. Here, I like to focus on two things, one is how a Timer control is used and is used separately in another class for more flexibility, and how an individual node can be blinked.
Using the code
Create a new form and add a TreeView
control. Apply these initial settings for the TreeView
control and the Button
controls in the form:
Me.TreeView_C.ImageIndex = -1
Me.TreeView_C.Location = New System.Drawing.Point(10, 10)
Me.TreeView_C.Name = "TreeView_C"
Me.TreeView_C.Nodes.AddRange(New System.Windows.Forms.TreeNode() _
{New System.Windows.Forms.TreeNode("Root Node", _
New System.Windows.Forms.TreeNode() _
{New System.Windows.Forms.TreeNode("Fruits", _
New System.Windows.Forms.TreeNode() _
{New System.Windows.Forms.TreeNode("Apple"), _
New System.Windows.Forms.TreeNode("Orange"), _
New System.Windows.Forms.TreeNode("Pineapple"), _
New System.Windows.Forms.TreeNode("Grapes")}), _
New System.Windows.Forms.TreeNode("Animals", _
New System.Windows.Forms.TreeNode() _
{New System.Windows.Forms.TreeNode("Lion"), _
New System.Windows.Forms.TreeNode("Tiger")})})})
Me.TreeView_C.SelectedImageIndex = -1
Me.TreeView_C.Size = New System.Drawing.Size(240, 250)
Me.TreeView_C.TabIndex = 0
Me.btnApplyColors.Location = New System.Drawing.Point(10, 265)
Me.btnApplyColors.Name = "btnApplyColors"
Me.btnApplyColors.Size = New System.Drawing.Size(80, 30)
Me.btnApplyColors.TabIndex = 1
Me.btnApplyColors.Text = "Apply Colors"
Me.btnSort.Location = New System.Drawing.Point(175, 265)
Me.btnSort.Name = "btnSort"
Me.btnSort.Size = New System.Drawing.Size(70, 30)
Me.btnSort.TabIndex = 2
Me.btnSort.Text = "Sort"
Me.btnBlinking.Location = New System.Drawing.Point(90, 265)
Me.btnBlinking.Name = "btnBlinking"
Me.btnBlinking.Size = New System.Drawing.Size(80, 30)
Me.btnBlinking.TabIndex = 3
Me.btnBlinking.Text = "Blinking"
The code below is used to control events:
Dim sortedAsc As Boolean = False
Dim BlinkingNodes As String = ""
Dim cnode As New TreeNode
Public Enum SortOrder
Ascending
Descending
End Enum
Private Sub btnApplyColors_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles btnApplyColors.Click
TreeView_C.SelectedNode.ForeColor = Color.Pink
End Sub
Private Sub btnSort_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles btnSort.Click
TreeView_C.SelectedNode.ForeColor = _
TreeView_C.DefaultForeColor
cnode = TreeView_C.SelectedNode
If sortedAsc = False Then
SortNode(SortOrder.Ascending)
sortedAsc = True
Else
SortNode(SortOrder.Descending)
sortedAsc = False
End If
End Sub
Private Sub btnBlinking_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles btnBlinking.Click
If BlinkingNodes.IndexOf(TreeView_C.SelectedNode.Text) < 0 Then
Dim obj As New BlinkTimer
obj.cnode = TreeView_C.SelectedNode
obj.cnode.ForeColor = TreeView_C.DefaultForeColor
obj.timer_c.Start()
BlinkingNodes = BlinkingNodes & ", _
" & TreeView_C.SelectedNode.Text
End If
End Sub
The sample function below sorts its subtree nodes on the selected base nodes:
Public Function SortNode(ByVal so As SortOrder)
Dim retTreenode As New TreeNode
Dim sSortString As String()
Dim iLoop, jLoop As Integer
ReDim sSortString(cnode.GetNodeCount(False) - 1)
retTreenode= cnode.Clone
For iLoop= 0 To sSortString.Length - 1
sSortString(iLoop) = cnode.Nodes(iLoop).Text
Next
If so = SortOrder.Ascending Then
sSortString.Sort(sSortString)
Else
sSortString.Reverse(sSortString)
End If
For iLoop= cnode.GetNodeCount(False) - 1 To 0 Step -1
cnode.Nodes(iLoop).Remove()
Next
For jLoop= 0 To sSortString.Length - 1
For iLoop= 0 To retTreenode.GetNodeCount(False) - 1
If sSortString(jLoop).Trim = _
retTreenode.Nodes(iLoop).Text Then
retTreenode.Nodes(iLoop).ForeColor = _
TreeView_C.DefaultForeColor
cnode.Nodes.Add(retTreenode.Nodes(iLoop))
End If
Next
Next
End Function
The sample class below is used internally to blink a selected node:
Public Class BlinkTimer
Public cnode As TreeNode
Public timer_c As New timer
Dim BlinkColor As Color = Color.DeepPink
Public Sub New()
AddHandler timer_c.Tick, AddressOf BlinkOn
End Sub
Public Sub BlinkOn(ByVal sender As System.Object, _
ByVal e As System.EventArgs)
If cnode.ForeColor.ToString <> BlinkColor.ToString _
And cnode.ForeColor.ToString <> _
Color.Transparent.ToString Then
cnode.ForeColor = Color.Transparent
End If
If cnode.ForeColor.ToString = Color.Transparent.ToString _
Or cnode.ForeColor.ToString = _
Color.Empty.ToString Then
cnode.ForeColor = BlinkColor
ElseIf cnode.ForeColor.ToString = _
BlinkColor.ToString Then
cnode.ForeColor = Color.Transparent
End If
End Sub
End Class
Points of Interest
On working with the Timer
control, it is better to assign a Timer
control in a separate class and access it, instead of assigning it to a control, since the Timer
control can be accessed with a single instance and used multiple times.