In this article, we are going to learn how to use Grunt.js to minify & uglify (JavaScript, cascading style sheets, Images and HTML) and to configure Task Runner Explorer in ASP.NET Core MVC.
What is Grunt.js?
Grunt.js is open source JavaScript toolkit for the automating repetitive tasks in the development process. It is similar to Gulp.js.
There are many tasks already available as Grunt Plugins. You just need to use it and auto your process.
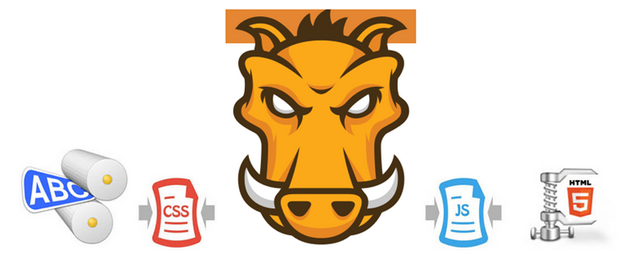
Let’s learn how to automate repetitive tasks using Grunt.js.
Topics
- Creating Application
- Adding package.json file to Project
- Downloading Dependencies of Grunt js
- Adding Gruntfile.js File to Project
- Writing Basic Task to Learn How to Start Using Grunt.js
- Installing "Task Runner Explorer" Extension in Visual Studio
- Writing Task to Minifying HTML File
- Writing Task to Concatenate File JavaScript Files
- Writing Task to Uglify and Minify JavaScript
- Writing Task to Minify Cascading Style Sheets
- Writing Task to Minify / Compress Images
- Writing Task to Copy Files From One Folder to Another Folder
- Running More than One Task in Sequence Using Grunt.js
Step 1: Creating Application
Now let’s create .NER Core Web Application. Open Visual Studio IDE from start page. Click on New Project link.

After clicking on New Project link, it will open a new dialog with Name "New Project". Inside that, from left panel, choose templates, inside that -> choose Visual C# -> inside that, choose .NET Core template, then in middle panel, you will see .NET Core project templates, from templates that choose "ASP.NET Core Web Application (.NET Core)" project templates.

After choosing a project template, next we are going to name the project "DemoGrunt
". Click on the OK button to create a project, and it will pop up another dialog with a name "New ASP.NET Core Web Application (.NET Core)".

Inside this dialog, we are going to choose the "Web Application" project template for create "ASP.NET Core Web Application" and click on the OK button for creating a project.
Below is the complete project view which is newly created:

After creating project, the next thing is to add package.json file to project.
Step 2: Adding package.json File in the Project
We are going to add package.json to the project for downloading packages from node package manager.
To add package.json, just right click on "DemoGrunt
" project, then select Add from menu list -> then under it, select New Item.

After selecting, a new dialog will pop up with a name "Add New Item" as shown below.
In this dialog, we are going to choose "npm Configuration File" and just click on Add button.

After clicking on Add button, you can see the package.json file is added to project as shown below:

Step 3: Downloading Grunt Packages
We are going to download packages:
grunt
grunt-contrib-concat
grunt-contrib-uglify
grunt-contrib-copy
grunt-minify-html
grunt-contrib-cssmin
grunt-tinyimg

Code Snippet to Add in package.json
Adding packages to the package.json file in the devDependencies
section for downloading packages.
{
"version": "1.0.0",
"name": "asp.net",
"private": true,
"devDependencies":
{
"grunt": "1.0.1",
"grunt-contrib-concat": "1.0.1",
"grunt-contrib-uglify": "2.0.0",
"grunt-contrib-copy": "1.0.0",
"grunt-minify-html": "2.1.0",
"grunt-replace": "1.0.1",
"grunt-contrib-cssmin": "1.0.2",
"grunt-tinyimg": "1.0.0"
}
}
Note
- folder/*.js - will match all the JavaScript files in folder
- folder/*.css - will match all the CSS files in folder
- folder/*.html - will match all the HTML files in folder
After adding Dependencies in the package.json file, just saving this file or the entire project, as you save, it will start downloading packages from Node Package Manager (NPM).

After downloading Dependencies next, we are going to add a Gruntfile.js file to project.
Step 4: Adding Gruntfile.js File in Project
In this step, we are going to add a Gruntfile.js file to project.
To add Gruntfile.js file, just right click on "DemoGrunt
" project, then select Add from menu list -> then under it, select New Item.

After selecting, a new dialog will pop up with name "Add New Item" as shown below.
In this dialog, we are going to choose "Grunt Configuration File" and just click on the Add button.

After clicking on Add button, it will add Gruntfile.js to project as shown below.
You can see some default code in Gruntfile.js files.

Step 5: Writing Basic Task to Learn How to Start using Grunt.js
- Now let’s write a simple message to display "Welcome to Grunt js in Visual Studio".
‘default
’ is the name of the task.
Below is the Complete Code Snippet of Gruntfile.js
module.exports = function (grunt)
{
grunt.registerTask('default', 'Task Description', function () {
grunt.log.write('Welcome to Grunt js in Visual Studio');
});
};
Now we have to run this task which is written in the Gruntfile.js. For doing that, we need to install extension "Task Runner Explorer".
Step 6: Installing "Task Runner Explorer" Extension in Visual Studio
For installing "Task Runner Explorer", just right-click on the Menu bar of Visual Studio in that, choose Tools Menu, inside that -> choose Extension and Updates.

After clicking on Extension and Updates, a new dialog will pop up as shown below in that you need to choose online tab from left, then in Search result, type "Task Runner Explorer"

Below is a snapshot after installing "Task Runner Explorer".
After installing, it will ask to restart Visual Studio.

After restarting Visual Studio next, we need to open Task Runner Explorer.
To open Task Runner Explorer, just click on Tools menu from Visual Studio and choose Task Runner Explorer Menu.

Snapshot of Task Runner Explorer
The Task Runner Explorer contains all tasks of Gruntfile.js and in Tasks, you will find the task which we have created "default" task.

Now we are going to run default task. To run it, just right-click on Task Name (default), then two options will appear from that, choose Run option (option with a play button in green color).

Below is the snapshot after running a task.

Wow, we have completed running the first task. It is easy, right?
After completing task next, we are going to learn about minifying HTML files using gruntfile.js.
Understanding Grunt Properties in Details

This information is taken from http://gruntjs.com/configuring-tasks.
Step 7: Writing Task to Minifying HTML File
In this step, we are going to write a task for minifying HTML files. To do that, first I am going to add a folder with name Htmlfiles in the wwwroot folder add them inside that folder, I am going to add an HTML file with name demo.html.
Folder Structure

And next, I am going to add another folder in a same wwwroot folder with name Compressedfiles. Here, we are going to store minified HTML files.
Folder Structure

After adding HomePage.html file, now let's add some text with space in this file.
Snapshot of HomePage.html File

After adding an HTML file with space, next we are going to write a task for minifying this HTML file and write it to other location (Compressedfiles) folder.
Below is the Complete Code Snippet of Gruntfile.js
module.exports = function (grunt) {
grunt.initConfig({
minifyHtml:
{
Html: {
src: ['wwwroot/Htmlfiles/**/*.html'],
dest: 'wwwroot/Compressedfiles/',
expand: true,
flatten: true
}
}
});
grunt.loadNpmTasks("grunt-minify-html");
};
In the above code snippet, we are using ["grunt-minify-html"]
plugin to minify HTML files along with this, we had also provided src (source) and dest (destination) folder path from where it is going to take HTML files (source) to minify and after minifying it, we are going to write it in a different folder in project (destination).
Understanding Code Snippet of Gruntfile.js
- The first step contains wrapper function and all Grunt related code that must be written inside this function.
module.exports = function (grunt) {
};
- In the second step, we are going to Initialize a configuration object:
module.exports = function (grunt) {
grunt.initConfig({
});
};
- In the third step, we are going to write a task "
minifyHtml
" inside the initConfig
method:
module.exports = function (grunt) {
grunt.initConfig({
minifyHtml:
{
}
});
};
- In the fourth step, we are going to write Target ("
Html
") inside Task "minifyHtml
"
module.exports = function (grunt) {
grunt.initConfig({
minifyHtml:
{
Html: {
}
}
});
};
Note: One Task can have many targets inside it.
- In the fifth step, we are going to configure properties source [src], destination [dest], expand, and flatten.
module.exports = function (grunt) {
grunt.initConfig({
minifyHtml:
{
Html: {
src: 'wwwroot/Htmlfiles/**/*.html',
dest: 'wwwroot/Compressedfiles/',
expand: true,
flatten: true
}
}
});
};
- Loading Grunt plugins and tasks "
grunt-minify-html
".
module.exports = function (grunt) {
grunt.initConfig({
minifyHtml:
{
Html: {
src: 'wwwroot/Htmlfiles/**/*.html',
dest: 'wwwroot/Compressedfiles/',
expand: true,
flatten: true
}
}
});
grunt.loadNpmTasks("grunt-minify-html");
};
Options
- Source [Src] = the path from where we are going to get all html files from Htmlfiles folder to minify
- Destination [Dest] = the path where we want to store all minified html files output in Compressedfiles folder
Finally, save Gruntfile.js and open Task Runner Explorer.
To run, just right click on "minifyHtml
" task and from the list of Tasks, choose Run.

After running task, let’s have look at Compressedfile folder where minified HTML file is stored.
Folder Structure

Output of Compressed File /Minified File

Step 8: Writing Task to Concatenate JavaScript Files
In this part, we are going to learn how to concatenate JavaScript files. For doing that, we are going to add four JavaScript files in a folder and then we are going to concat all JavaScript files into one single JavaScript file.
Folder Structure

In every JavaScript file, I am going to add a single JavaScript function in it such that we can understand how it concats files.

After writing all functions in JavaScript individual files, now let's write the task on Gruntfile.js to concat all these files to one single file.
One thing we have missed here is where we are going to store all concatenated JavaScript files for storing that file, I am going to create a new folder with name "ConcatenatedJsfiles" in which all JavaScript files which are concatenated will store in this folder.
Folder Structure

Below is the Complete Code Snippet of Gruntfile.js
module.exports = function (grunt) {
grunt.initConfig({
concat:
{
all:
{
src: ['wwwroot/js/**/*.js'],
dest: 'wwwroot/ConcatenatedJsfiles/combined.js'
}
}
});
grunt.loadNpmTasks("grunt-contrib-concat");
};
- Source [Src] = the path from where we are going to get all JavaScript files from JS folder to concat
- Destination [Dest] = the path where we want to store all Concatenated JavaScript files output in ConcatenatedJsfiles folder.
In the above code snippet, we are using ["grunt-contrib-concat"]
plugin to concat JavaScript files along with this, we had also provided src (source) and dest (destination) folder path from where it is going to take JavaScript files (source) to concat and after concating, it is going to write it at different folder in project (destination).
Finally, save Gruntfile.js and open Task Runner Explorer.
To run, just right click on "concat
" task and from the list of menu, choose Run menu.
Snapshot of Task Runner Explorer

After running task, let’s have look at "ConcatenatedJsfiles" folder where concatenated JavaScript file is stored.
Folder Structure

Output of Concatenated JavaScript Files

Step 9: Writing Task to Uglify and Minify JavaScript
In this step, we are going to write a task for uglifying and minifying JavaScript file for doing that first, I am going to add a folder with name MinifiedJavascriptfiles in wwwroot folder in this folder, all uglifying and minifying JavaScript files will be stored and we are going to get all JavaScript files from "Js" folder for uglifying and minify.
Folder Structure

JavaScript File to Minify

Below is the Complete Code Snippet of Gruntfile.js
module.exports = function (grunt)
{
grunt.initConfig({
uglify:
{
js:
{
src: ['wwwroot/js/**/*.js'],
dest: 'wwwroot/MinifiedJavascriptfiles/Calculation.js'
}
}
});
grunt.loadNpmTasks("grunt-contrib-uglify");
};
In the above code snippet, we are using ["grunt-contrib-uglify"]
plugin to uglify and minify JavaScript files along with this, we had also provided src (source) and dest (destination) folder path from where it is going to take JavaScript files (source) to uglify and minify and after uglifying and minifying, it is going to write it at different folder in project (destination).
Finally, save Gruntfile.js and open Task Runner Explorer.
To run, just right click on "uglify" task and from the list of menu, choose Run menu.
Snapshot of Task Runner Explorer

After running task, let’s have look at "MinifiedJavascriptfiles" folder where minified JavaScript file is stored.
Folder Structure

Output of Minified JavaScript Files

Step 10: Writing Task to Minify Cascading Style Sheets
In this step, we are going to write task for minifying cascading style sheets file. For doing that, first I am going to add a folder with name MinifiedCssfiles in wwwroot folder in this folder all minified cascading style sheets files will be stored and we are going to get all cascading style sheets files from "css" folder for minifying.

Cascading Style Sheets File to Minify

Below is the Complete Code Snippet of Gruntfile.js
module.exports = function (grunt) {
grunt.initConfig({
cssmin:
{
css:
{
src: ['wwwroot/css/**/*.css'],
dest: 'wwwroot/MinifiedCssfiles/site.min.css'
}
}
});
grunt.loadNpmTasks("grunt-contrib-cssmin");
};
In the above code snippet, we are using ["grunt-contrib-cssmin"]
plugin to minify CSS (Cascading Style Sheet) files along with this, we had also provided src (source) and dest (destination) folder path from where it is going to take CSS (Cascading Style Sheet) files (source) to minify and after minify, it is going to write it in a different folder in project (destination).
Finally, save Gruntfile.js and open Task Runner Explorer. To run, just right click on "Cssmin" and from the list of menu, choose Run menu.
Snapshot of Task Runner Explorer

After running task, we have to see "MinifiedCssfiles" folder where minified cascading style sheets file is stored.
Folder Structure

Output of Minified CSS Files

Step 11: Writing Task to Minify / Compress Images
In this step, we are going to Compress Images. To do this, I am going to use the "grunt-tinyimg
" plugin. Next, we are going to create a folder with name Compressimages in a wwwroot folder. In this folder, all Compress images will be stored and we are going to get all Images from the "images" folder for compressing.

Below is the Complete Code Snippet of Gruntfile.js
module.exports = function (grunt) {
grunt.initConfig({
tinyimg:
{
minifyimage:
{
src: ['wwwroot/images/**/*.{png,jpg,gif,svg}'],
dest: 'wwwroot/Compressimages/',
expand: true,
flatten: true
}
}
});
grunt.loadNpmTasks("grunt-tinyimg");
};
In the above code snippet, we are using ["grunt-tinyimg"]
plugin to minify Images files. Along with this, we had also provided src (source) and dest (destination) folder path from where it is going to take images (source) to minify images and after minifying, we are going to write it at different folder in project (destination).
Finally, save Gruntfile.js and open Task Runner Explorer.
To run, just right click on "tinyimg
" and from the list of menu, choose Run menu.
Snapshot of Task Runner Explorer

Snapshot of Images before Compressing

Snapshot of Images after Compressing

Step 12: Writing Task to Copy Files From One Folder to Another Folder
In this step, we are going to write a task for copying files from one folder to another folder.
For the demo, I am going to show how to copy HTML files from one folder to another folder in the project.
Package used "grunt-contrib-copy
".
I have added Htmlfiles folder inside the wwwroot folder where I need to move my HTML files from the TempHtmlFiles folder located outside of the wwwroot folder.

Below is Complete Code Snippet of Gruntfile.js
module.exports = function (grunt) {
grunt.initConfig({
copy: {
html:
{
src: 'TempHtmlFiles/**/*.html',
dest: 'wwwroot/Htmlfiles/',
expand: true,
flatten: true,
filter: 'isFile'
}
}
});
grunt.loadNpmTasks("grunt-contrib-copy");
};
In the above code snippet, we are using a ["grunt-contrib-copy"]
plugin to copy files along with this, we had also provided src (source) and dest (destination) folder path from where it is going to copy files (source) and after copying it is going to write it at different folder in project (destination).
Finally, save Gruntfile.js and open Task Runner Explorer.
To run, just right click on "copy" Task and from the Tasks list of menu and choose Run menu.
Snapshot of Task Runner Explorer

Snapshot of Copied Files in Htmlfiles Folder

Step 13: Running More Than One Task in Sequence Using Grunt.js
In this step, we are going to learn how to run more than on one task in particular sequence.
For doing that, we are going to use the registerTask()
method of Grunt.js and this method has three overloading.
Parameters
name
: The name of the task Callback
: A function that is run when the task is executed Message
: Log a message Array
: A string array of task names



Below Snapshot of Code Snippet Shows How to Run More Than One Task in Sequence Using Grunt.js

In the above code snippet, we are using two plugins ["grunt-contrib-copy, grunt-contrib-uglify"]
, one for copying files and another for uglifying JavaScript files.
We are going to run two tasks in sequence, first copying files task and another is uglifying JavaScript files task. In copying files task, we are going to copy files from one folder to another folder and in uglify task, we are going uglify and minify JavaScript files and then write it at a different folder in the project.
Finally, save Gruntfile.js and open Task Runner Explorer.
To run, just right click on aliased task "all" and from the list of menu, choose Run menu.
Snapshot of Task Runner Explorer

Snapshot of Copied Files in Htmlfiles folder

Finally, we have completed a short journey of learning using of Grunt js with ASP.NET Core MVC, I hope you have enjoyed it.
By using Grunt plugin, you can create different tools which are going to help in day to day process and reduce time to work on core process of your project.
History
- 25th January, 2017: Initial version