Introduction To 2nd Edition
Draw A Pattern, Generate A Strong Password
What if you could draw one pattern on screen and generate a strong password (SHA256-based) for each of the sites you log into?
With the newest version of DragPass you can do exactly that.
If You've Read the First Edition of This Article
Not A Great Video, But It's Only Two Minutes Long
Here's a link to a youtube video showing it in action and an overview explanation how it works.
dragpass : An alternate (and more secure way) to generate your passwords - YouTube[^]
Below is a snapshot of the new UI.
Much More To Discuss
If you've already read the first version of the article, there have been a lot of changes and you can skip directly to the the new additions to the article by clicking the following link: Revision 2 Starts Here
If you haven't read the article, start the Introduction to the original article below and progress through. This article builds upon an idea and in the end, it is more about the idea of destroying passwords than possibly even the implementation. Passwords must change.
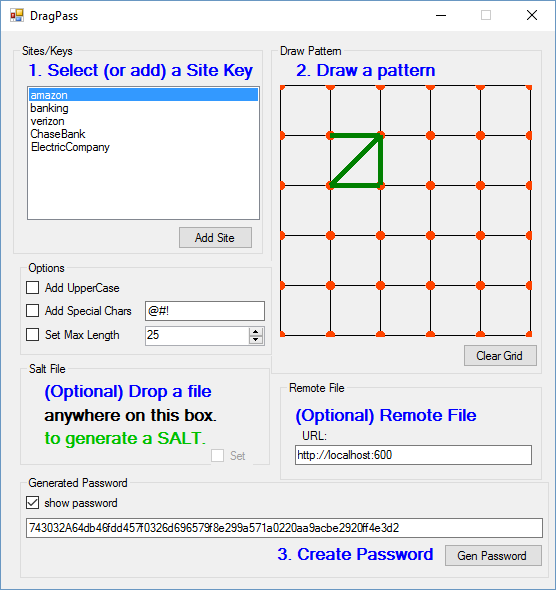
Introduction To First Edition
What Is This Article Really About?
This article is an attempt to start a conversation related to the following subjects:
1. passwords are annoying and cause vulnerabilities for numerous reasons.
2. Why do we require users to memorize and create passwords when they are obviously incapable of creating strong passwords?
Main Focus of This Article
I show you complete C# code which creates a method for creating strong passwords which the user never knows about, has to memorize or even sees. I know that someone else somwhere has probably thought about this already, but I haven't seen it discussed so I hope this article will help to start that discussion.
I'm also hoping to gather input, because there may be (are?) some flaws in my idea about the way that I am generating the passwords. What vulnerabilities do you see in my methods?
Background: What Got Me Thinking About Passwords
Consider Non-Technical Users (99.9% Of People On Internet)
First of all, you have to consider all the non-technical users of out there. To them, passwords are an annoyance and most of them either write them down or simply use one password for all their web sites. Either option creates a vulnerability.
Then, recently while checking a file on VirusTotal.com a thought struck me:
Quote:
Any file on your computer could be a unique password.
What? Let me explain how this idea came about. (I'm writing this as a walk-thru of my ideas so please stick with me as the idea progresses even if you notice that the first ideas may seem wrong.)
On Friday, I was thinking about the SHA-256 hash algorithm which VirusTotal uses to identify files.
If you go to VirusTotal - Free Online Virus, Malware and URL Scanner[^] and upload a file you can see it in action.
I uploaded the Bluestream.bmp from my Toshiba laptop and VirusTotal already knew this file because someone else had previously uploaded it.

One-Way Hash Is Unique to Every Set of Bytes
The SHA-256 hash that is uniquely generated for the set of bytes in the Bluestream.bmp file is:
6f6045a6e3449df05def5542c0a79127d2688a49672ad820a42ef659a81409f4
If you change one byte in that file it will generate an entirely different hash. Let me show you how simple the code is to generate the the hash from some bytes.
SHA256 mySHA256 = SHA256Managed.Create();
byte [] allBytes = Encoding.UTF8.GetBytes("super simple");
byte [] hashBytes = mySHA256.ComputeHash(allBytes);
StringBuilder outstring = new StringBuilder();
foreach (byte b in hashBytes ) { outstring.AppendFormat("{0:x2}", b);}
Console.WriteLine(outstring.ToString());
The first line creates an object which is set up to run the SHA-256 hashing algorithm for you.
The second line simply creates a byte array from the string "super simple"
The third line passes the byte array to the ComputeHash()
function of the SHA256 object.
ComputeHash()
returns an array of bytes which represent the unique hash value. However, those bytes may contain unprintable characters so we have to convert them to insure they are displayable. To do that, I iterate through each byte and call the StringBuilder
method AppendFormat()
. The format specifier {0:x2} formats the output to a two character hex value. This format will match the format used by VirusTotal.
Finally, I print the value out using Console.WriteLine().
The output on your computer will be the exact same as mine for those bytes and it will be:
3ea0100a11a0e118e2e8eabe2e5d723b6836151b39843347f84ea361a3757fa4
Think Differently About Passwords
One of the biggest problems with passwords is:
User's Create Weak Passwords
User's create bad passwords, based upon common words they can remember.
Creating bad passwords based upon words found in natural language allows hackers to do dictionary attacks against passwords. In other words, hackers can keep on guessing words or word-pairs until they've guessed the user's password.
The other main problem with passwords is:
Requiring the User To Know Their Passwords Creates Vulnerability
Because user's have to memorize their passwords, they end up creating weak passwords (so they can remember them). Or, they end up writing them down. Saving them in a file or use other methods which create additional vulnerabilities.
How VirusTotal Hashed A File
The difference between the code you just saw and the code at VirusTotal is that VirusTotal generated a hash on a file and not just a few bytes of a string. That just means that VirusTotal read the bytes from the file and then generated the hash on the bytes contained in the file that was uploaded.
It's very easy to change our code so that it will read bytes from a file. It's only a one line code change (see bold line in the following code sample:
SHA256 mySHA256 = SHA256Managed.Create();
byte [] allBytes = File.ReadAllBytes(@"c:\temp\supersimple.txt");
byte [] hashBytes = mySHA256.ComputeHash(allBytes);
StringBuilder outstring = new StringBuilder();
foreach (byte b in hashBytes ) { outstring.AppendFormat("{0:x2}", b);}
Console.WriteLine(outstring.ToString());
I'm using the static class System.IO.File
to call the ReadAllBytes()
method which reads the bytes of a file into a byte array, It's very convenient.
supersimple.txt Genereates Exact Same Hash
I used Notepad++ to create a file which contained the exact same string : "super simple" and saved it to the file.
That means the exact same bytes are being hashed and so when we run this code you get the same hash value generated as before:
3ea0100a11a0e118e2e8eabe2e5d723b6836151b39843347f84ea361a3757fa4
You can download the supersimple.txt
(zip file at top of this article) and try it yourself. Just make sure you change the path to the file accordingly. Also, make sure you unzip the file first too. If you run the hash on the zipped file you'll get a different hash, because the bytes are different. The zip has a hash of:
32bdbe113b9d2565755596aa4afb420095a261d3f1caa623525e488b774b85c0
Uploaded supersimple.txt To VirusTotal.com
I uploaded the supersimple.txt file to VirusTotal.com. You can see in the snapshot below, it generated the same hash value.

VirusTotal Identifies A File By Its Unique Hash
If you upload supersimple.txt it will let you know that it has previously scanned the file. It can do that because it identifies the file based upon the unique hash generated from the file's bytes.
Leap of Imagination : Hoping For Simplicity
This means that if we could provide an easy way to a user to point at a file and generate a unique hash, we could create a very complex password for that user (the hash value). Imagine how simple that would be for a user. All she would have to do is remember a file that she uses as her password generator. She'd never have to memorize any complex password or write anything down.
But, could we create a simple program that does that for the user? Absolutely.
Download Working Program : Version 1
You can download the DragPass_v001.zip to get the code for this section.
What Does DragPass Do?
The program I call DragPass allows a user to drag any file and drop it on the form. When the user does so, the SHA256 hash value will be generated from the bytes in the file and then displayed in the textbox (if the checkbox is checked).
Copies has to clipboard : It will also copy the value to the clipboard so that even if the hash isn't displayed the user can simply paste it into his password box on a web site and be done.

You can see that I dragged and dropped the supersimple.txt file onto the form and it generated the same hash value.
Generate Hash For Any File On Your Computer
You can open up File Explorer and drag and drop any file that is on your computer and this program will generate the SHA256 hash for it. That's kinds of neat.
Drag and Drop Desktop Shortcuts
An additionally neat feature of this program is that you can drag and drop any desktop shortcut onto it and it will generate a hash code that way too. That could be a cool way to keep your passwords easily available. Just generate some shortcuts, assign an icon and drag and drop them onto the form and use the generated hash as your password.
Code Wrapped Up On A Form
I basically just wrapped up our previous code on a form and made it so that when a file is dropped on the form the code runs. There's not a whole lot of code we had to write to create the app so I'll let you take a look at it in the code download. The drag and drop stuff is the most interesting part of the code so we'll take a look at it.
Drag and Drop Is Easy
Basically you just register the form to receive the drag and drop events in the form constructor and then you handle those events.
Here's what the constructor looks like:
public MainForm()
{
InitializeComponent();
this.AllowDrop = true;
this.DragEnter += new DragEventHandler(MainForm_DragEnter);
this.DragDrop += new DragEventHandler(MainForm_DragDrop);
}
In that case the this variable is MainForm so we are registering it so that the allows the drop event.
After that we set up the EventHandlers for each event. Finally we write the code that runs for each of those events.
void MainForm_DragEnter(object sender, DragEventArgs e)
{
if (e.Data.GetDataPresent(DataFormats.FileDrop)) e.Effect = DragDropEffects.Copy;
}
void MainForm_DragDrop(object sender, DragEventArgs e)
{
string[] files = (string[])e.Data.GetData(DataFormats.FileDrop);
foreach (string file in files)
{
var m256 = SHA256Managed.Create();
byte[] allBytes = File.ReadAllBytes(file);
byte [] hashBytes = m256.ComputeHash(allBytes);
StringBuilder pwd = new StringBuilder();
foreach (byte b in hashBytes) { pwd.AppendFormat("{0:x2}", b); }
if (showPasswordCheckBox.Checked)
{
passwordTextBox.Text = pwd.ToString();
}
Clipboard.SetText(pwd.ToString());
}
}
The DragEnter EventHandler changes the cursor so the user knows that something is happening.
Of course, you can see the the DragDrop EventHandler runs the code for opening the file, getting the bytes and generating the SHA256 hash. Finally, we copy the hash to the clipboard for easy using as a password.
What Are the Weaknesses of Such An Approach?
However, you may be thinking, "Well, surely that doesn't seem safe, since a hacker could gain access to my computer and generate hashes on all my files."
Yes, that is true, however, they'd have to then try every one of those hashes against your user account to see if it worked also. That's probably not likely, but let's add another layer of security to this and we'll learn a bit more about hashing also.
Adding A Salt For More Security
Download
You can see the completed code by downloading DragPass_v002.zip file from the top of this article.
Okay, so since a hacker could generate hashes for each of your files let's add another layer of security by salting the hash. What is salting the hash? It's a simple way of adding another factor of complexity into the one-way hash algorithm by allowing the user to insert new bytes into the string value which is hashed.
Two Factors To Hash
Basically, you allow the user to add some bytes so that the final input to the hash is different and this will obviously cause the final hash to be different. This requires the hacker to not only be able to hash every file on your computer to obtain the final hash, because now the hash is not only based upon the file, but it is also based upon some additional bytes.
What Will We Allow the User To Salt With?
I suggest we simply have the user choose two files to create the hash. The huge number of files on a computer would make it virtually impossible for a hacker to guess which two files were chosen for to generate the password. First, let's take a look at how the program works from the user's perspective and then we can discuss the simple code modifications.
Drop A File With No Salt : For Comparison
Here's what the program looks like in version 2 when you drop the same supersimple.txt file on it (but do not generate a salt value.

Since I am now allowing the user to drop two files I have to provide seperate areas for her to do that. I've created two groupboxes and now there are seperate Drag and Drop events for each groupbox. In this example I dropped the supersimple.txt on the right groupbox and a hash was created with no salt. That's why the hash value is the same as in the previous example.
Now, I'll drop a shortcut on the left groupbox to set the salt value (which the user will never see) and then I'll drop the same supersimple.txt file on the right and you'll see that the final hash is completely different since it's been salted.

You can see that the hash value for the supersimple.txt has changed to :
0ab7d9aad8606c99fd4a918fd638a1282df5cf4e519eb5c5a71da556b14ded3d
You would not be able to recreate that value since you don't know which file I used for the salt so I have provided it in the code download. The salt file I used is named salt.txt
.
Generate New Hash Using New File
Each time you drag and drop a new file onto the right side, a new hash will be generated using the same salt file.
That makes it easier to set the salt one time and then generate your hashes from numerous files if you like.
Change The Salt File
If you want to change the salt file you can right-click on the left side and a menu item will appear allowing you to clear the salt file so you can either:
- create hashes without the salt
- add a new salt to be used in subsequent hash cretions.

Less For The User To Remember and More Secure Password
Think about the benfits.
- With this method, the user can more easily generate a more secure password (not based upon natural language).
- The user is only required to remember two files to generate a password. He is not forced to remember some long and complex password.
Imagine A Complete Scenario For Multiple Devices
Suppose users are directed to pick one file as a salt. A user could take a picture with his phone use it for his salt.
Provide A Salt From Google Drive (Dropbox, OneDrive, etc)
After that, he could transfer the file to his Google Drive and we could create a way for the user to select that file from his Android device, laptop, or whatever device he is working on. The same way he chose the salt from his local machine. However, this remote location would provide another layer of security since, even if the hacker got on his computer the hacker wouldn't necessarily have access to the remote drive or file.
Same Files, Generate Same Hash (Password)
Since the same files generate the same hash, which we use as the password, as long as the user has those files available she can generate her password from anywhere.
SHA256 Is A Standard Algorithm
Since SHA256 is a standard algorithm I can write the hash creation code on any platform so that the user can easily select two files from her iPhone, Android Phone, Windows laptop or any device and generate her password at any time so she can sign on to any of her web sites from any of her devices and she is more secure than ever.
What Are The Possible Dangers?
If the user attempts to remember his password and salt files by naming them indiscretely such as myPwdFile.jpg and saltfile.png then a hacker may be able to find the two files easily.
Can you think of other security exposures related to this? I hope you'll reply if you do.
What Are the Challenges?
User education is the biggest challenge. You have to explain to them that the password which is created never needs to be stored anywhere. That's because it is generated each time the user will sign in. She will simply drag and drop the same two files onto the interface, the password will be generated and copied to the clipboard for pasting into the password field on login form.
Getting People To Use It
Getting people to use it (marketing) is always the most difficult thing. Most non-technical users just won't know this may create a stronger password than anything they are creating now. It probably does.
Password Requirements
Also, some sites may have password requirements of using special characters or some nonsense. I suppose we could add a feature to add one special character to the end of the hash and then the app would just use that same character every time to fulfill the site's requirement.
Feedback : Request For Comments
I hope this has stimulated your imagination on how to make better passwords that are more secure. I hope that if I've missed any huge gap you'll respond and start a conversation. I think this is a fascinating topic and the challenge of changing this problematic yet core element of modern computing is an interesting challenge.
Revision 2 Starts Here
Now Supports the Following Requested Features
The biggest change is that now you can simply draw a pattern (which you duplicate each time) and that is part of what generates your hash (much more explanation further in the article).
- Generate different passwords for multiple sites based on your hidden token info (file, drawn pattern, etc)
- Add uppercase chars to password
- Add special chars to password
- Ability to use remote file as salt to hash via URL
- Of course, your passwords are not saved anywhere, instead they are generated each time (much more in the article)
The Positive Response Has Been Amazing
This article has been a lot of fun because so many great people have chimed in and added great ideas to the discussion.
That's why I added all of these new features.
You Can Create & Recreate Your Passwords Without Ever Memorizing Characters
Instead, now you can just memorize a pattern you draw on the grid.
The General Idea How New DragPass Works
The new DragPass should probably be renamed to DrawPass, because now you can basically draw a unique pattern and generate a hash-based password.
Two Things Required To Generate Password
There are only two things required to generate a password:
- Site key - any text which will allow you to remember the site you will be logging into
- A drawn pattern
What Is the Site Key and What Does It Do?
The site key is any text (string) that will help you remember what the password is for.
You enter a list of these in the ListBox at the top left:

This Is Where Many People's Brains Will Melt
When you add each site / key the item will be added to a file named dragpass.json
in the same directory where you started DragPass from.
What Is DragPass.json and What Are The Implications?
The project implements the NewtonSoft JSON library, obtained via Nuget (package manager).
This is just an easy way to allow the user to add her list of sites so she can easily generate passwords for them.
Oh No! What About Cracker Getting This File (List of Sites)?
Let the brain-melting begin!
Quite a few people are worried about crackers (they're really not hackers) getting on the user's machine and reading their files. I understand. It really does happen. Trojan viruses are everywhere. However, if the cracker is on your machine, then he has probably installed a keylogger and that is going to be way more effective at grabbing passwords.
Even If The Cracker Gets Your JSON File
Keep in mind that the data in the JSON file is a part of the salt that goes into creating the password, but it is only used so the user can create many passwords off of one pattern.
Keep in mind that there are two required values to generate the basic password so even if they had your json site entries they'd also need to generate all the values for the possible (almost infinite) graphic patterns.
Sigh...but alas, I expect a healhty amount of argument about this (and other things we will read about in the rest of the article).
Benefits of Working Together
It's all good though, because we are all working together to create a better system for users and destroy bad passwords forever.
Continuing our discussion, once you have the list items you will simply click the appropriate one to generate the password.
If you click the [Gen Password]
button at this point, it will warn you that you need to draw a pattern.
The text you enter into that list box will be converted to bytes and used to generate the hash.
Draw A Pattern
Now, you need to draw a pattern in the top right grid. All you have to do is click any of the grid posts in succession and line segments will begin to be drawn. As soon as you have at least one line segment, you can click the [Gen Password]
button and a unique and strong password will be generated.
How Does Drawing On The Grid Generate A Hash Value?
This is an interesting part of the code so we need to talk about how the final hash is generated if the user does only the following -- with no other options:
- selects a site
- draws a pattern
Domain Classes For Organization
I have created a few classes to help me keep everything organized. These are classes which came from analysis of the problem domain. They are very simple, but allow people new to the code to dissect the code more easily to see what it is doing. I want transparency in the code as much as possible since this is a nascent idea.
class LineSegment
{
public Point Start { get; set; }
public Point End { get; set; }
public LineSegment(Point start, Point end)
{
Start = start;
End = end;
}
}
I created a LineSegment class to simply to wrap up a couple of Point objects which will create the lines on the screen.
I've also created a plural of that class (LineSegments
- notice the "s") which has some additional functionality (related to generating a value which will go into creating the hash).
class LineSegments : List<LineSegment>
{
public String PostPoints { get; set; }
public int PostValue { get; set; }
public void AddOn(int pointValue)
{
PostValue += pointValue;
PostPoints += String.Format("{0},", pointValue);
}
}
This class is a List of the first class. This is how we keep track of all the lines that the user draws to create her pattern.
You can see that there is also a couple of properties (PostPoints
and PostValue
). Originally I was using PostPoints for debugging so I could test no matter how the user drew the shape I would be able to generate the same value every time.
PostValue
The PostValue
is the property we are interested in. It's not named very well. It was supposed to be related to the red dots which I call posts which are on the grid.
Drawing On A Windows Form
There's quite a bit of code just to allow the user to draw on the Windows form but I won't go into all of that. You can examine it for yourself. On the MainForm you'll find the important method (which should probably be in the domain class) named CalculateGeometricSaltValue()
private void CalculateGeometricSaltValue()
{
LineSegments.PostPoints = String.Empty;
LineSegments.PostValue = 0;
foreach (LineSegment l in LineSegments)
{
for (int x = 0; x < allPosts.Count; x++)
{
if (l.Start.X == allPosts[x].X && l.Start.Y == allPosts[x].Y)
{
System.Diagnostics.Debug.Print(string.Format("START x : {0}", x));
LineSegments.AddOn(x);
}
if (l.End.X == allPosts[x].X && l.End.Y == allPosts[x].Y)
{
System.Diagnostics.Debug.Print(string.Format("END x : {0}", x));
LineSegments.AddOn(x);
}
}
}
System.Diagnostics.Debug.Print(String.Format("Value : {0}",LineSegments.PostValue));
System.Diagnostics.Debug.Print(LineSegments.PostPoints);
}
It is in this method where I do the following:
- Insure that a LineSegment is only added once -- even if a user attempts to draw over it numerous times.
- Calculate the PostValue for the graphic shape the user has drawn.
Once that point value is generated we can use it later as a Salt when creating the hash.
Optional Items
Since many sites inforce specific requirements on their passwords I've added the ability to :
- add uppercase letter
- add 1 or more special characters
- set a max length -- it's odd to me that sites would do this, because it limits things.

Here's a password generated without the extra options turned on and then the same password with the the extra options (look closely, you'll see an uppercase letter and the rest).

After selecting all options:

You can see the overview of the work to create the hash in the MainForm method where the [Gen Password] button is clicked.
private void GenPasswordButton_Click(object sender, EventArgs e)
{
CalculateGeometricSaltValue();
if (SiteListBox.SelectedIndex < 0)
{
MessageBox.Show("You must select a Site/Key item to generate a password.");
return;
}
if (LineSegments.PostValue == 0)
{
MessageBox.Show("You must create a pattern to generate a password.");
return;
}
ComputeHashBytes();
if (addUpperCaseCheckBox.Checked)
{
AddUpperCaseLetter(pwdBuilder);
}
String pwd = pwdBuilder.ToString();
if (AddSpecialCharsCheckBox.Checked)
{
pwd = AddSpecialChars(pwd);
}
if (SetMaxLengthCheckBox.Checked)
{
pwd = pwd.Substring(0, Math.Min((int)MaxLengthUpDown.Value,pwd.Length));
}
if (showPasswordCheckBox.Checked)
{
passwordTextBox.Text = pwd.ToString();
}
Clipboard.SetText(pwd.ToString());
}
The basic steps are:
- Calculate the Geomtric Salt Value -- if the user hasn't drawn one he is warned that he must
- ComputeHashBytes -- use all items which user has chosen to create the hash salted with each required and optional item.
- Add in any special characters, uppercase
- limit the length as necessary
Now, let's take a look at how the Hash is actually generated and we'll look at the other optional items (using a remote file and/or local file).
ComputeHashBytes
This is where the hash is actually generated. Of course there are various amounts of salt sprinkled on, depending upon whether or not the user has chosen certain optional items such as using a remote file and/or a local file.
private void ComputeHashBytes()
{
var m256 = SHA256Managed.Create();
var patternBytes = System.Text.Encoding.UTF8.GetBytes(LineSegments.PostValue.ToString());
var selItemText = SiteListBox.SelectedItem.ToString();
var siteBytes = System.Text.Encoding.UTF8.GetBytes(selItemText);
byte[] allBytes = null;
if (SaltIsSetCheckBox.Checked)
{
allBytes = new byte[patternBytes.Length + siteBytes.Length + saltBytes.Length];
patternBytes.CopyTo(allBytes, 0);
siteBytes.CopyTo(allBytes, patternBytes.Length);
saltBytes.CopyTo(allBytes, patternBytes.Length + siteBytes.Length);
}
else {
allBytes = new byte[patternBytes.Length + siteBytes.Length];
patternBytes.CopyTo(allBytes, 0);
siteBytes.CopyTo(allBytes, patternBytes.Length);
}
if (remoteFileUrlTextBox.Text != string.Empty && remoteFileData.Length > 0)
{
if (remoteFileData.Length > 0)
{
var remoteBytes = System.Text.Encoding.UTF8.GetBytes(remoteFileData.ToString());
byte [] tempBuffer = new byte[allBytes.Length + remoteBytes.Length];
allBytes.CopyTo(tempBuffer, 0);
remoteBytes.CopyTo(tempBuffer, allBytes.Length);
allBytes = tempBuffer;
}
}
byte[] hashBytes = m256.ComputeHash(allBytes);
pwdBuilder = new StringBuilder();
foreach (byte b in hashBytes) { pwdBuilder.AppendFormat("{0:x2}", b); }
}
Remote File Bytes: What Is That?
I have added a feature which allows you to point at a remote file to add an additional salt to the hash.
You can grab a URL from your browser's navigation bar and drag it to the text box and it will be pasted in.
Here's what it looked like when I dragged in a folder from my google drive:

I'm Scared! Please Hold Me
The first thing someone is going to think is, "That's crazy! You are pulling bytes across the line and a cracker could sniff that data and have your file." Well, yes!
Use HTTPS : Nice Solution
Except that if you use a URL which is HTTPS, then a cracker cannot sniff the file bytes and get them. And if she can, then the entire Internet is OVER!! :)
The next thing you may say, is, "But that file may change and then you cannot recreate your password hash."
The File May Change
You are correct again. Howeve,r imagine if you drop a file in your dropbox, Google Drive, Live Drive, etc. that only you have a link too (and includes an HTTPS URL) then you can do this safely to further salt your hash.
File corruption could happen to any file at any time so that is always a concern. Protect yourself as you need. However, how many times is a file you get from a remote location on the Internet corrupt? Mostly rarely. And, you should be changing your password often anyways, even with this fantastic new way of generating passwords.
Local File As Salt
You can also still use a Local File as a salt. Just drag it and drop it like you did before.
Multiple Factors of Salt Make It Quite Strong
Now, if you really want to you can use all four factors to salt your final hash:
- site key - required
- geometric pattern - required
- local file
- remote file
The Fact That There Are Four Makes It More Difficult For Crackers
You see, now the crackers would have to try such an infinite number of variations that it wouldn't be worth the time to attempt to crack your password.
Real Cryptography : What Real Cryptographers Know
That's the thing that real cryptographers know. A method of encryption only has to be strong enough for a certain amount of time. That's why cryptographic methods are based on units of time and processing power.
Information Has An Expiration Date
The information you have has an expiration date. That means if it takes the crackers 6 months to generate your password, then you should be changing it every 5 months and you are safe. Even modern methods of cryptography are coming under scrutiny because of processing power.
Probably Strong Enough, Probably Stronger Than What You Use
This method probably creates a stronger method than what you currently use to create your passwords (or at least 90% of the users out there).
It is highly improbable that a cracker would take the time to crack a password that involved this much work. (Oh, that is going to send people into a case of the apoplectic vapors!!) :)
Possible Weaknesses
Geometric Value Generation
It may be possible that a cracker could generate a huge number of values that represent various geometric values by studying the LineSegment creation and PointValues
. Unlikely, but we know them crackers are bored people.
JSON Site File
We can encrypt that file in the future so it can only be read on the system it was created. It's a possible exposure and there are some other ideas to secure it also.
Conclusion
I'm hoping that you see passwords in an entirely new light.
I'm hoping that you actually read the article and think about how it might be right and how it might be wrong. I absolutely love the discussion going on about this. So many fantastic people out there have had kind comments.
I want us all to think about better methods for passwords because users (in general) are not creating secure passwords and use the same passwords everywhere.
I hope you'll try the software and join the discussion.
A Few Items of Confusion / Concern
I'm adding these as quick notes to show some things that have come up and some ideas behind them. Please feel free to discuss any of them.
Copying To Clipboard
A few people thought this was dangerous. However, the clipboard is very protected and also, of course, if someone is on your machine and able to read your clipboard, then you are toast anyways. :)
However, if you don't like the part where it copies to the clipboard, then you can just remove that code.
Password Generated From File Bytes Concerned A Few
This one was interesting, because in the first revision I showed you how you could simply select two files (one as a salt and the other as the hash) to generate a password.
A few people absolutely disagreed, because they thought someone could :
- get all of your files
- simply go through every one and pair it with every other one and generate your hash.
The Problems With That Theory
- The hacker is already on your machine (if she has access to all of your files). If this is true you are toast since the hacker would've already installed a keylogger and gotten your passwords. :)
- It would take a long, long time to generate all the possible hashes by pairing every two files (in both orders too)
- Even if the hacker did generate the astronomical number of hashes that would have to be generated, she cannot tell which one is correct until she attempts to log in to your account with each of them. She would most surely get locked out by the time she tried the several million hashes. :)
Why Would These Hash-based Passwords Be Less Safe?
I'm not sure why a password based upon a SHA256 hash (which is considered cryptographically safe at this time and is used by the entire IT industry) would be any more unsafe than someone creating a password from their mind.
History
Article 2nd Edition : 2015-06-03 - code release DragPass_v003.zip (at top of article)
Article and code first release : 2015-05-16