This demo focuses on using ASP.NET Scaffolding to generate the controllers and views.
STEP 1 - Create a New Project on Visual Studio 2015
- Open Visual Studio
- Create a new ASP.NET Web Application Project
- Give a name to the project (in this case, I call him MVC5)
- Select Template MVC
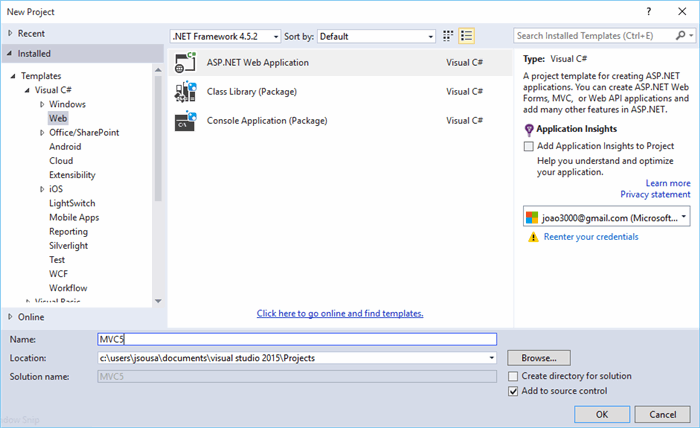
STEP 2 - Create class Movies
Add a new class to the project (this class represents a table, and the properties the columns of that table).
Give a name to that class (in my sample, I call him MoviesModel
).
On the class, create the following code:
using System;
using System.Collections.Generic;
using System.Data.Entity;
using System.Dynamic;
using System.Linq;
using System.Web;
namespace MVC5.Models
{
public class Email
{
public int ID { get; set; }
public string To { get; set; }
public string Subject { get; set; }
public string Message { get; set; }
}
public class EmailDBContext : DbContext
{
public DbSet<email> Emails { get; set; }
}
}
Put the class inside the Models folders, just to organize your code.

STEP 3 - Create a controller with views using Entity Framework
Add new Scaffolded to the project (new item existent on MVC5):

Choose option MVC5 Controller with views using Entity Framework:

Click Add
If you receive an error, it may be because you did not build the project in the previous section. If so, try building the project, and then add the scaffolded item again.

After the code generation process is complete, you will see a new controller and views in your project.
STEP 4 - Controller and Views Generation
a. The Controller was automatically created with CRUD operations
using System;
using System.Collections.Generic;
using System.Data;
using System.Data.Entity;
using System.Linq;
using System.Threading.Tasks;
using System.Net;
using System.Web;
using System.Web.Mvc;
namespace MVC5.Models
{
public class MoviesController : Controller
{
private MovieDBContext db = new MovieDBContext();
public async Task<actionresult> Index()
{
return View(await db.Movies.ToListAsync());
}
public async Task<actionresult> Details(int? id)
{
if (id == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
Movie movie = await db.Movies.FindAsync(id);
if (movie == null)
{
return HttpNotFound();
}
return View(movie);
}
public ActionResult Create()
{
return View();
}
[HttpPost]
[ValidateAntiForgeryToken]
public async Task<actionresult> Create([Bind(Include =
"ID,Title,ReleaseDate,Genre,Price")] Movie movie)
{
if (ModelState.IsValid)
{
db.Movies.Add(movie);
await db.SaveChangesAsync();
return RedirectToAction("Index");
}
return View(movie);
}
public async Task<actionresult> Edit(int? id)
{
if (id == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
Movie movie = await db.Movies.FindAsync(id);
if (movie == null)
{
return HttpNotFound();
}
return View(movie);
}
[HttpPost]
[ValidateAntiForgeryToken]
public async Task<actionresult>
Edit([Bind(Include = "ID,Title,ReleaseDate,Genre,Price")] Movie movie)
{
if (ModelState.IsValid)
{
db.Entry(movie).State = EntityState.Modified;
await db.SaveChangesAsync();
return RedirectToAction("Index");
}
return View(movie);
}
public async Task<actionresult> Delete(int? id)
{
if (id == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
Movie movie = await db.Movies.FindAsync(id);
if (movie == null)
{
return HttpNotFound();
}
return View(movie);
}
[HttpPost, ActionName("Delete")]
[ValidateAntiForgeryToken]
public async Task<actionresult> DeleteConfirmed(int id)
{
Movie movie = await db.Movies.FindAsync(id);
db.Movies.Remove(movie);
await db.SaveChangesAsync();
return RedirectToAction("Index");
}
protected override void Dispose(bool disposing)
{
if (disposing)
{
db.Dispose();
}
base.Dispose(disposing);
}
}
}
b. The Views were automatically created

Run the application:


MVC5 Resources
Some good online resources about MVC5:
ASP.NET MVC5: The official Microsoft .NET WebSite http://www.asp.net/mvc/tutorials/mvc-5 
For more examples, follow my blog at http://joaoeduardosousa.wordpress.com/ 
Check My Other Source Code
I am João Sousa, and since i finish my degree I’m working in software development using Microsoft technologies.
I was awarded
Microsoft Most Valuable Professional (MVP) 2015 – .Net
My profissional profile:
Azure Developer
.NET Developer
My Certifications:
MCTS - .NET Framework - Application Development Foundation
MCTS - .NET Framework 2.0 - Windows-based Client Development
MCTS - .NET Framework 3.5 ADO.NET Applications
MCTS - .NET Framework 3.5 ASP.NET Applications
MCSD - Programming in HTML5 with JavaScript and CSS3
MCSD - Developing ASP.NET MVC 4 Web Applications
MCSD - Developing Windows Azure and Web Services
MCSA Office 365 - Managing Office 365 Identities and Requirements
MCSA Office 365 - Enabling Office 365 Services
MCSD - Implementing Microsoft Azure Infrastructure Solutions