Introduction
While developing Media Assistant, I faced several issues, mostly with the performance. I have written another
article titled Replacing TreeView with ListBox, where I explained
how I simulated a listbox to look like a tree view to support virtualization. I had to do that because a tree view performs very, very slow if there are about 20,000 nodes.
I faced a similar issue when I added a Thumbnail View to display the movies in the repository. I used a ListBox
where I changed the ItemsPanel
to WrapPanel
. When I use the WrapPanel
, the ListBox
loses its virtualization ability and it takes a lot of time to display 5000 movies in my repository.
Because WrapPanel
doesn't support virtualization. In this article, I will explain how I virtualized the WrapPanel
.
Thumbnail View
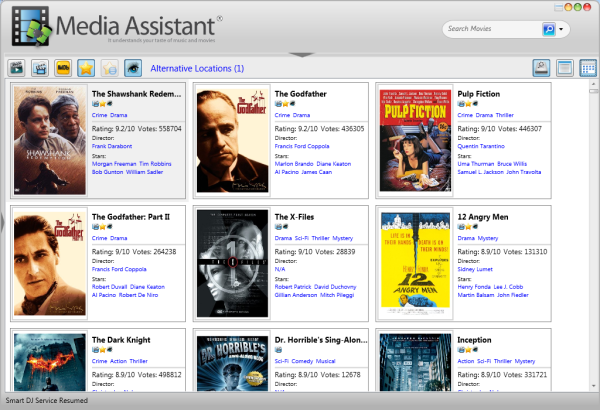
ListBox with WrapPanel
I changed the ItemsPanel
of ListBox
with WrapPanel
to show the thumbnail view.
<ListBox x:Name="thumbnailListBox" ItemsSource="{Binding DataSource.ResultMovies}"
ScrollViewer.HorizontalScrollBarVisibility="Disabled"
SelectedItem="{Binding DataSource.SelectedMovie}"
ScrollViewer.IsDeferredScrollingEnabled="True"
ItemTemplate="{StaticResource NormalThumbnailTemplate}"
ScrollViewer.ScrollChanged="HandleScrollChanged"
>
<ListBox.ContextMenu>
<ContextMenu>
<MenuItem Header="Play" Command="{Binding DataSource.PlayMovieCommand}"/>
<Separator/>
<MenuItem Header="Show in Windows Explorer"
Command="{Binding DataSource.ShowMovieInWindowsExplorerCommand}"/>
</ContextMenu>
</ListBox.ContextMenu>
<ListBox.ItemsPanel>
<ItemsPanelTemplate>
<WrapPanel/>
</ItemsPanelTemplate>
</ListBox.ItemsPanel>
</ListBox>
Once I used the WrapPanel
with ListBox
, it displays the movies in a thumbnail like view without virtualization. I could not find any solution to virtualize
the WrapPanel
. So, I investigated which part of my code was taking time. Then I found that individual thumbnail items take some time to render because I used
an image, there was some processing with each item, and I was using the converters, etc.
I tried with a simple border without anything in it with WrapPanel
and it displays very quickly. So, I tried to use the detail movie view with an image and other
information only for the items which are in the visible area, and all other items which are not in the visible area with a simple border. I kept changing the views while the user scrolled.
I subscribed to the ScrollChanged
event and used an ItemTemplate
which switches between a normal border and a detail movie view.
Thumbnail Template
<DataTemplate x:Key="NormalThumbnailTemplate">
<Border Width="300" Height="200" BorderThickness="1" Margin="5" BorderBrush="Gray">
<ContentControl x:Name="content" Content="{Binding}" ContentTemplate="{x:Null}"/>
</Border>
<DataTemplate.Triggers>
<DataTrigger Binding="{Binding IsVisible}" Value="True">
<Setter TargetName="content" Value="{StaticResource NormalThumbnail}" Property="ContentTemplate"/>
</DataTrigger>
</DataTemplate.Triggers>
</DataTemplate>
In the movie data, I used a property IsVisible
which is used in the DataTrigger
to switch to the thumbnail view. NormalThumbnail
is the DataTemplate
which displays the detail movie information with image and other data. So I'm not going to explain that part in this article.
How to identify which items are in the visible area of the ListBox
private void HandleScrollChanged(object sender, ScrollChangedEventArgs e)
{
ShowVisibleItems(sender);
}
private void ShowVisibleItems(object sender)
{
var scrollViewer = (FrameworkElement) sender;
var visibleAreaEntered = false;
var visibleAreaLeft = false;
var invisibleItemDisplayed = 0;
foreach (Movie item in thumbnailListBox.Items)
{
if (item.IsVisible) continue;
var listBoxItem =
(FrameworkElement) thumbnailListBox.ItemContainerGenerator.ContainerFromItem(item);
if (visibleAreaLeft==false &&
IsFullyOrPartiallyVisible(listBoxItem, scrollViewer))
{
visibleAreaEntered = true;
}
else if (visibleAreaEntered)
{
visibleAreaLeft = true;
}
if(visibleAreaEntered)
{
if(visibleAreaLeft && ++invisibleItemDisplayed>10)
break;
var job = new Job(MakeVisible);
job.Store.Add(item);
job.Start();
}
}
}
This code simply finds those items which are fully or partially visible and marks them as visible. I used a Job
class which marks the items to be visible
in a different thread. I did it just to delay the trigger. But it is not necessary in most cases. So, ignore that part.
protected bool IsFullyOrPartiallyVisible(FrameworkElement child, FrameworkElement scrollViewer)
{
var childTransform = child.TransformToAncestor(scrollViewer);
var childRectangle = childTransform.TransformBounds(
new Rect(new Point(0, 0), child.RenderSize));
var ownerRectangle = new Rect(new Point(0, 0), scrollViewer.RenderSize);
return ownerRectangle.IntersectsWith(childRectangle);
}
This code identifies if an item is in the visible area of the scroll viewer. Then I find the visible items rectangle and the view point's rectangle
in the same co-ordinate system and find the intersection. And I'm done.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.