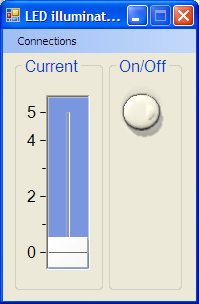
Figure 1. User interface

Figure 2. Photo of the hardware setup
(
Click for larger photo).
Introduction
In order to bring-up a small piece of hardware, I had to whip-up a quick utility for controlling an LED light source. My tools of choice for this type of task are a C#, National Instrument Measurement Studio, USB-6008 input/output card. At some point, I realized that a write-up like this one may be useful for those who are scratching the surface of Measurement Studio.
Hardware
The setup is built around National Instruments USB-6008 multipurpose I/O device. I was connected to the laptop via USB. Constant current LED driver and LED itself were on a separate board (more detailed schematic).
LED output is proportional to the current. The driver circuit is design so that the LED current is proportional to the control voltage V_SET_I
, which is generated by one of the analog outputs on USB-6008. Another digital control signal turns LED on and off.
Figure 3. Block diagram of the hardware setup.
(I've also uploaded the
schematic of the constant current LED driver.)
Software
The purpose of the software is to provide a simple GUI, which allows the user to control the LED intensity with a slider and to turn the LED on/off with a toggle button.
Class Diagram
Subclasses of DAQmx.Task
are responsible for accessing the hardware channels. They expose public
methods and properties while hiding the details such as physical designation of the channels involved. For our tasks, additional writer objects (AnalogSingleChannelWriter
and DigitalSingleChannelWriter
) are also created.

A tempting mistake is to try to create a task that contains more than one type of channel. For example, it could be analog output channel (AO) and digital output channel (DO).
this.AOChannels.CreateVoltageChannel
("Dev2/ao1", "LED brightness", 0, 5, AOVoltageUnits.Volts);
this.DOChannels.CreateChannel("Dev2/port0/line0", "LED on",
ChannelLineGrouping.OneChannelForEachLine);
Figure 5.
This is easy to address by making a separate subclass of DAQmx.Task
for each of the channel types you want to use (see Figure 4).
About the Source Code
The code for this project was hand-written. At the same time, MStudio has a wizard that can generate code for tasks. If you’d like to learn more about the wizard, have a look at [4]. The code for the project is written in a bare bones style: has hard coded parameters, no sanity checking, no error handling. That makes it easier to see the calls to MStudio.
Points of Interest
Let’s take a look at one of the DAQmx.Task
subclasses - the LEDOn
. Its purpose is to toggle a digital output. The bare bones code is small:
class LEDOn : NationalInstruments.DAQmx.Task
{
private DigitalSingleChannelWriter m_dscwLEDon;
public void Init()
{
this.DOChannels.CreateChannel("Dev2/port0/line0", "LED on",
ChannelLineGrouping.OneChannelForEachLine);
this.Control(TaskAction.Verify);
m_dscwLEDon = new DigitalSingleChannelWriter(this.Stream);
}
public bool On
{
set { m_dscwLEDon.WriteSingleSampleSingleLine(true, value); }
}
}
The form class instantiates the LEDOn
class (and other task classes), then it calls the initialization, then it can write to the channels.
Initialization
Before using a channel, a small initialization has to take place:
- A channel is created within the task.
- Task is verified.
- Appropriate writer is created and connected to the task’s stream.
All this is done in LEDOn.Init():

Figure 6. State transition diagram for a DAQmx.Task object
Writing to the Channel
Writing to the channel is done by calling the WriteSingleSampleSingleLine()
method of the channel writer object. Other types of channels have similar WriteX()
methods. Notice that autoStart
is set to true
. The task briefly goes to the Running
state, writes the sample, goes back to Committed
state. There is a useful article in the MStudio online help “Using the Start Task function/VI”, which details when to call Start()
and Stop()
, and how autoStart
works.
Closing Remarks
I’m planning to add a more elaborate version of this project that has sanity checking and error handling.
As always, bug notes, suggestions, insight, comments, requests, etc. are welcome!
References
[1] Official home page for National Instruments Measurement Studio
[2] .NET section of Measurement Studio forum
[3] USB-6008 multipurpose I/O device
[4] Walkthrough: Creating a MeasurementStudio NI-DAQmx Application
[5] Measurement Studio .NET class hierarchy chart
Acronyms and Abbreviations
NI | National Instruments |
MStudio | Measurement Studio made by NI |
DAQ | Data Acquisition |
History
- Initial draft. September 26, 2010