Introduction
Last week at work, I had to work on a C++/CLI class that derived from a class written in C# which implemented IDisposable
. I got it wrong at first (yeah, same guy who wrote a book on the subject a couple of years ago), and my boss and I spent some time going through the generated code in Reflector before fixing it up. I came home that night and quickly put together a simple project so I could see the whole picture from a simple perspective. I thought it would benefit others to put together an article reflecting (no pun intended) the issue.
The C# base class
Here's a simple C# class that implements IDisposable
. It's assumed that the class handles both managed and native resources.
namespace DisposeDemo
{
[SuppressMessage("Microsoft.Naming",
"CA1709:IdentifiersShouldBeCasedCorrectly",
MessageId = "Cs",
Justification = "Personal preference")]
public class CsDisposableBase : IDisposable
{
#region IDisposable Members
public void Dispose()
{
Dispose(true);
GC.SuppressFinalize(this);
}
~CsDisposableBase()
{
Dispose(false);
}
protected virtual void Dispose(bool disposing)
{
if (disposing)
{
DisposeManagedResources();
}
FreeNativeResources();
}
#endregion
private void DisposeManagedResources()
{
this.WriteLine("Disposing managed resources in base class");
}
private void FreeNativeResources()
{
this.WriteLine("Freeing native resources in base class");
}
public CsDisposableBase(String name)
{
this.Name = name;
}
public String Name { get; private set; }
protected void WriteLine(String text)
{
Console.WriteLine("{0} : {1}", this.Name, text);
}
}
}
For any C# developer inheriting from this class, it's a no-brainer to override and implement void Dispose(bool)
as the class requirements dictate. But things are not so obvious from C++/CLI. C++/CLI does not permit any method named "Dispose
" in your class. While you've all probably implemented IDisposable
classes in C++/CLI before, you may not have yet derived from a C# IDisposable
base before. Anyway, the trick is to avoid over-thinking and complicating the issue - all you need to do is to handle it just as you'd do normally. Put the managed-disposal in the destructor and the native-disposal in the finalizer.
The C++/CLI derived class
Here's the derived class implementation in C++/CLI:
namespace CppDisposable
{
public ref class DisposableDerived : CsDisposableBase
{
private:
void DisposeManagedResourcesDerived()
{
WriteLine("Disposing managed resources in derived class");
}
void FreeNativeResourcesDerived()
{
WriteLine("Freeing native resources in derived class");
}
public:
~DisposableDerived()
{
DisposeManagedResourcesDerived();
this->!DisposableDerived();
}
!DisposableDerived()
{
FreeNativeResourcesDerived();
}
DisposableDerived(String^ name) : CsDisposableBase(name)
{
}
};
}
At first glance, the code seems wrong. How do we ensure that the base class disposal code (and the finalizer) are called as and when needed? Let's write some test code to see what happens.
int main(array<System::String ^> ^args)
{
CppDisposable::DisposableDerived object1(
"Object that gets disposed");
CppDisposable::DisposableDerived^ object2 =
gcnew CppDisposable::DisposableDerived("Object that gets GCd");
return 0;
}
Running that gives the following output :-
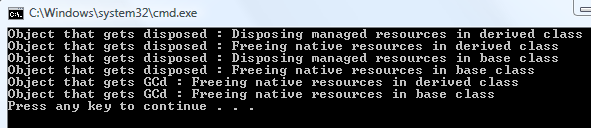
Wow. Everything worked fine. How did that happen? The answer lies in VC++ compiler magic. A look at the generated code using Reflector shows us that the compiler has generated the code that we may otherwise have had to write ourselves.
What Reflector shows
Here's the generated code (as viewed in Reflector):
protected override void Dispose([MarshalAs(UnmanagedType.U1)] bool flag1)
{
if (flag1)
{
try
{
this.~DisposableDerived();
}
finally
{
base.Dispose(true);
}
}
else
{
try
{
this.!DisposableDerived();
}
finally
{
base.Dispose(false);
}
}
}
So, during a Dispose
call, here's what will happen:
- The C# base class
Dispose
will call Dispose(true)
and suppress GC. - The C++/CLI implementation will call
~DisposableDerived
(which frees the managed resources in the derived class and then calls !DisposableDerived
which frees the native resources in the derived class). - Now,
base.Dispose(true)
is called (in the finally
-block) which frees the managed and native resources in the base class.
And this is what happens during a finalization:
- The C# base class finalizer calls
Dispose(false)
. - The C++/CLI implementation calls
!DisposableDerived
(which frees the native resources in the derived class). - And
base.Dispose(false)
is called (in the finally
-block) which frees the native resources in the base class.
Essentially, this code is exactly what we'd have needed to write - the compiler generates it for us.
Conclusion
I know this is a very simple article, but sometimes it's the simple things that we stumble over. I wish I had covered this topic in my book (where I talk about deterministic destruction). As always, please feel free to submit feedback (critical or otherwise).
History
- July 5, 2008 - Article published.
Nish Nishant is a technology enthusiast from Columbus, Ohio. He has over 20 years of software industry experience in various roles including Chief Technology Officer, Senior Solution Architect, Lead Software Architect, Principal Software Engineer, and Engineering/Architecture Team Leader. Nish is a 14-time recipient of the Microsoft Visual C++ MVP Award.
Nish authored C++/CLI in Action for Manning Publications in 2005, and co-authored Extending MFC Applications with the .NET Framework for Addison Wesley in 2003. In addition, he has over 140 published technology articles on CodeProject.com and another 250+ blog articles on his WordPress blog. Nish is experienced in technology leadership, solution architecture, software architecture, cloud development (AWS and Azure), REST services, software engineering best practices, CI/CD, mentoring, and directing all stages of software development.
Nish's Technology Blog :
voidnish.wordpress.com