Introduction
Consider that there is requirement to drag some object e.g image and drop it over other object from one place to another.This document explains how to achieve this using javascript.<o:p>
For any object to participate in drag drop functionality requires to support events which enable drag drop.If object is not supporting these events directly then we should have container object which supports these events.<o:p>
<o:p>
Container which supports drag drop events is required .we are using DIV.<o:p>
<o:p>
<div id='dMain' <o:p>
ondragstart='fnGetSource();' ondragenter='cancelevent();' ondragover='cancelevent();' ondrop='fnGetDestination();'><o:p>
<o:p>
<img id='Image1' ></img> //Source Image to be dragged<o:p>
<o:p>
<img id='Image2' ></img> //target image on which source image will be dropped<o:p>
<o:p>
</div><o:p>
<o:p>
If we drag Image1 and drop over image2 then Event ondragstart will get fired on source object . function fnGetSource will be executed.we will get id of source object.<o:p>
<o:p>
<o:p>
fnGetSource()<o:p>
{<o:p>
var srcElementId = event.srcElement.id ;<o:p>
alert(srcElementId);<o:p>
}<o:p>
<o:p>
<o:p>
When we release mouse over target object ,ondrop event gets fired on target object.This will execute function fnGetDestination.we will get id of destination object<o:p>
<o:p>
fnGetDestination()<o:p>
{<o:p>
var destElementId = event.srcElement.id ;<o:p>
alert(destElementId);<o:p>
}<o:p>
<o:p>
We need to handle 2 more events ondragenter and ondragover. These events are automatically fired on target object when we start dragging(ondragenter) and drags over valid drop target(ondragover).<o:p>
<o:p>
function cancelevent ()<o:p>
{<o:p>
window.event.returnValue = false;<o:p>
}<o:p>
<o:p>
Consider sample application where we need to drag image from one area and drop it to another as shown in following screens 1 and 2.<o:p>
<v:shapetype id="_x0000_t75" coordsize="21600,21600" o:spt="75" o:preferrelative="t" path="m@4@5l@4@11@9@11@9@5xe" filled="f" stroked="f"><v:stroke joinstyle="miter"><v:formulas><v:f eqn="if lineDrawn pixelLineWidth 0"><v:f eqn="sum @0 1 0"><v:f eqn="sum 0 0 @1"><v:f eqn="prod @2 1 2"><v:f eqn="prod @3 21600 pixelWidth"><v:f eqn="prod @3 21600 pixelHeight"><v:f eqn="sum @0 0 1"><v:f eqn="prod @6 1 2"><v:f eqn="prod @7 21600 pixelWidth"><v:f eqn="sum @8 21600 0"><v:f eqn="prod @7 21600 pixelHeight"><v:f eqn="sum @10 21600 0"><v:path o:extrusionok="f" gradientshapeok="t" o:connecttype="rect"><o:lock v:ext="edit" aspectratio="t"><o:p>
<o:p> 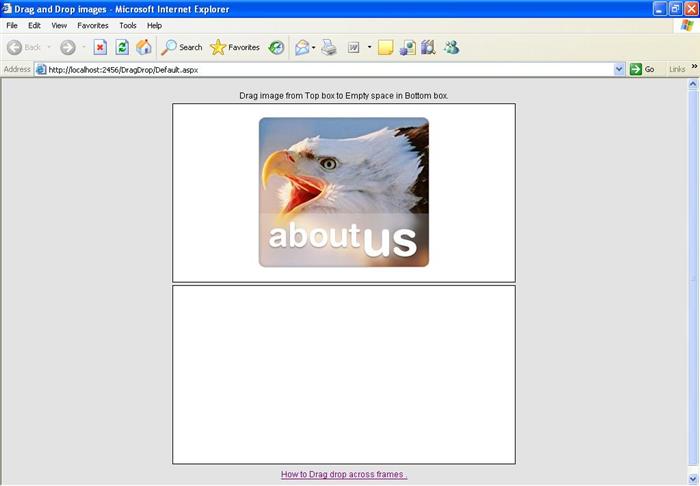
<o:p>

<o:p>
<o:p>
sample code for testing.<o:p>
<%@ Page Language="VB" AutoEventWireup="false" CodeFile="test1.aspx.vb" Inherits="test1" %><o:p>
<o:p>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"><o:p>
<html xmlns="http://www.w3.org/1999/xhtml"><o:p>
<head runat="server"><o:p>
<title>Drag and Drop images</title><o:p>
<o:p>
<script language="JavaScript1.2"><o:p>
var srcElementId; //Id of dragged object<o:p>
var destElementId; //Id of valid drop target object <o:p>
<o:p>
function fnGetSource()<o:p>
{<o:p>
srcElementId = event.srcElement.id ;<o:p>
} <o:p>
function fnGetDestination()<o:p>
{<o:p>
destElementId = event.srcElement.id;<o:p>
var dest = document.getElementById(destElementId).innerHTML ;<o:p>
document.getElementById(destElementId).innerHTML = dest + "<br><img src='images/small_0.jpg' />" <o:p>
document.getElementById("Top").innerHTML = "";<o:p>
} <o:p>
function cancelevent()<o:p>
{<o:p>
window.event.returnValue = false;<o:p>
}<o:p>
</script><o:p>
<o:p>
<style><o:p>
.cssNormalText<o:p>
{<o:p>
font-family: Arial, Helvetica, sans-serif;<o:p>
font-size: 12px;<o:p>
}<o:p>
</style><o:p>
</head><o:p>
<body style="background-color: #E3E3E3;"><o:p>
<form id="form1" runat="server"><o:p>
<table border="0" width="100%"><o:p>
<tr><o:p>
<td align="center"><o:p>
<div class="cssNormalText"><o:p>
Drag image from Top box to Empty space in Bottom box.<o:p>
</div><o:p>
</td><o:p>
</tr><o:p>
<tr><o:p>
<td align="center" valign="middle"><o:p>
<div id="Top" style="border: solid 1px black; height: 260px; width: 500px; overflow: auto;<o:p>
background-color: White;" ondragstart='fnGetSource();' ondragover='cancelevent();'><o:p>
<br /><o:p>
<img src="images/small_0.jpg" /><o:p>
</div><o:p>
</td><o:p>
</tr><o:p>
<tr><o:p>
<td align="center" valign="middle"><o:p>
<div id="bottom" style="border: solid 1px black; height: 260px; width: 500px; background-color: White;"<o:p>
ondragenter='cancelevent();' ondragover='cancelevent();' ondrop='fnGetDestination();'><o:p>
</div><o:p>
</td><o:p>
</tr><o:p>
</table><o:p>
</form><o:p>
</body><o:p>
</html><o:p>
<o:p> |
<o:p>
<o:p>
1.ondragenter Event<o:p>
Fires on the target element when the user drags the object to a valid drop target.<o:p>
Syntax<o:p>
Inline HTML<o:p> |
<ELEMENT ondragenter = "handler" ... > <o:p> |
All platforms<o:p> |
Event property<o:p> |
object.ondragenter = handler<o:p> |
JScript only<o:p> |
object.ondragenter = GetRef("handler")<o:p> |
Visual Basic Scripting Edition (VBScript) 5.0 or later only<o:p> |
Named script<o:p> |
<SCRIPT FOR = object EVENT = ondragenter> <o:p> |
Internet Explorer only<o:p> |
Event Information<o:p>
Bubbles<o:p> |
Yes<o:p> |
Cancels<o:p> |
Yes<o:p> |
To invoke<o:p> |
- Drag the selection over a valid drop target within the browser. <o:p>
- Drag the selection to a valid drop target within another browser window. <o:p>
|
Default action<o:p> |
Calls the associated event handler.<o:p> |
Event Object Properties<o:p>
Although event handlers in the DHTML Object Model do not receive parameters directly, a handler can query an event object for data.<o:p>
Remarks<o:p>
You can handle the ondragenter event on the source or on the target object. Of the target events, it is the first to fire during a drag operation. Target events use the getData method to stipulate which data and data formats to retrieve. The list of drag-and-drop target events includes: <o:p>
When scripting custom functionality, use the returnValue property to disable the default action.<o:p>
<o:p>
2.ondragover Event<o:p>
Fires on the target element continuously while the user drags the object over a valid drop target.<o:p>
Syntax<o:p>
Inline HTML<o:p> |
<ELEMENT ondragover = "handler" ... > <o:p> |
All platforms<o:p> |
Event property<o:p> |
object.ondragover = handler<o:p> |
JScript only<o:p> |
object.ondragover = GetRef("handler")<o:p> |
Visual Basic Scripting Edition (VBScript) 5.0 or later only<o:p> |
Named script<o:p> |
<SCRIPT FOR = object EVENT = ondragover> <o:p> |
Internet Explorer only<o:p> |
Event Information<o:p>
Bubbles<o:p> |
Yes<o:p> |
Cancels<o:p> |
Yes<o:p> |
To invoke<o:p> |
- Drag the selection over a valid drop target within the browser. <o:p>
- Drag the selection to a valid drop target within another browser window. <o:p>
|
Default action<o:p> |
Calls the associated event handler. <o:p> |
Event Object Properties<o:p>
Although event handlers in the DHTML Object Model do not receive parameters directly, a handler can query an event object for data.<o:p>
Remarks<o:p>
The ondragover event fires on the target object after the ondragenter event has fired.<o:p>
When scripting custom functionality, use the returnValue property to disable the default action.<o:p>
3.ondragstart Event<o:p>
Fires on the source object when the user starts to drag a text selection or selected object. <o:p>
Syntax<o:p>
Inline HTML<o:p> |
<ELEMENT ondragstart = "handler" ... > <o:p> |
All platforms<o:p> |
Event property<o:p> |
object.ondragstart = handler<o:p> |
JScript only<o:p> |
object.ondragstart = GetRef("handler")<o:p> |
Visual Basic Scripting Edition (VBScript) 5.0 or later only<o:p> |
Named script<o:p> |
<SCRIPT FOR = object EVENT = ondragstart> <o:p> |
Internet Explorer only<o:p> |
Event Information<o:p>
Bubbles<o:p> |
Yes<o:p> |
Cancels<o:p> |
Yes<o:p> |
To invoke<o:p> |
Drag the selected text or object.<o:p> |
Default action<o:p> |
Calls the associated event handler. <o:p> |
Event Object Properties<o:p>
Although event handlers in the DHTML Object Model do not receive parameters directly, a handler can query an event object for data.<o:p>
Remarks<o:p>
The ondragstart event is the first to fire when the user starts to drag the mouse. It is essential to every drag operation, yet is just one of several source events in the data transfer object model. Source events use the setData method of the dataTransfer object to provide information about data being transferred. Source events include ondragstart, ondrag, and ondragend. <o:p>
When dragging anything other than an img object, some text to be dragged must be selected.<o:p>
<o:p>
4.ondrop Event<o:p>
Fires on the target object when the mouse button is released during a drag-and-drop operation.<o:p>
Syntax<o:p>
Inline HTML<o:p> |
<ELEMENT ondrop = "handler" ... > <o:p> |
All platforms<o:p> |
Event property<o:p> |
object.ondrop = handler<o:p> |
JScript only<o:p> |
object.ondrop = GetRef("handler")<o:p> |
Visual Basic Scripting Edition (VBScript) 5.0 or later only<o:p> |
Named script<o:p> |
<SCRIPT FOR = object EVENT = ondrop> <o:p> |
Internet Explorer only<o:p> |
<o:p> Event Information<o:p>
Bubbles<o:p> |
Yes<o:p> |
Cancels<o:p> |
Yes<o:p> |
To invoke<o:p> |
Drag the selection over a valid drop target and release the mouse.<o:p> |
Default action<o:p> |
Calls the associated event handler.<o:p> |
Event Object Properties<o:p>
Although event handlers in the DHTML Object Model do not receive parameters directly, a handler can query an event object for data.<o:p>
Remarks<o:p>
The ondrop event fires before the ondragleave and ondragend events.<o:p>
When scripting custom functionality, use the returnValue property to disable the default action.<o:p>
As of Microsoft Internet Explorer 5, drag-and-drop events can be used to carry out drag-and-drop activities, not only with input type=text elements, but also with block and inline tags. For example, text can be selected, dragged, then dropped on a div target. This causes several target events to fire, including ondragenter, ondragover, and ondrop. Because drag-and-drop actions are not directly supported on block and inline tags, you must use extra scripting to carry out the move or copy to the target using innerText, for example.<o:p>
You must cancel the default action for ondragenter and ondragover in order for ondrop to fire. In the case of a div, the default action is not to drop. This can be contrasted with the case of an input type=text element, where the default action is to drop. In order to allow a drag-and-drop action on a div, you must cancel the default action by specifying window.event.returnValue=false in both the ondragenter and ondragover event handlers. Only then will ondrop fire.<o:p>
<o:p>
5.ondrag Event<o:p>
Fires on the source object continuously during a drag operation.<o:p>
6.ondragleave Event<o:p>
Fires on the target object when the user moves the mouse out of a valid drop target during a drag operation.<o:p>
7. Ondragend Event<o:p>
Fires on the source object when the user releases the mouse at the close of a drag operation.<o:p>
Sequence of event firing<o:p>
1)Ondragstart :source(ondrag, Ondragend)<o:p>
2)Ondrag :source (continuously)<o:p>
3)Ondragenter:target<o:p>
4)Ondragover:target(continuously)<o:p>
5)ondrop : target<o:p>
6)Ondragleave:target<o:p>
7)Ondragend :source
Technology used
Sample code is developed using Microsoft dot net technology C# .