Visual Studio Code (VS Code) has been my favorite editor for a few years. With the activity enhancement made by Microsoft and the community, VS Code has been evolved to a whole level. With the release of remote develop extension, now I can use VS Code for my daily work and my open source projects on all kinds of environments. This article is a brief tutorial of the VS Code setup for Python developments – the setup I use daily. If someone happens to want to use the VS Code for Python development, hopefully, this article could save the person’s time. VS Code has tremendous documents. Visit this link for more details.
This tutorial uses a simple Python project to demonstrates the VS Code setup for Python development on Windows 10, Windows Subsystem for Linux, remote Linux, and Raspbian on Raspberry Pi.
The Python project has the following layout:
project
|-- mathlib
| |-- __init__.py
| |-- compute.py
|-- test_compute.py
And the contents of compute.py and test_compute.py are:
compute.py
def inc(value: int) -> int
return value + 1
def dec(value: int) -> int
return value - 1
test_compute.py
from mathlib import compute
def test_int():
assert compute.inc(value=5) == 6
def test_dec():
assert compute.dec(value=5) == 4
Windows-Centralized Setup
Although VS Code can run on different platforms, e.g., Linux and MacOS, this tutorial uses Windows 10 as the main place to run VS Code.
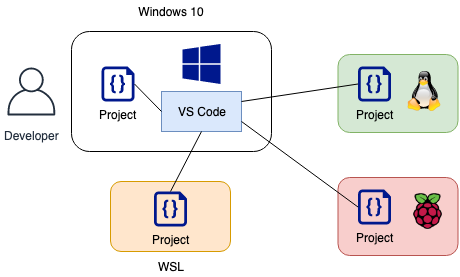
Assumptions and Requirements
The software used in this tutorial are:
- Visual Studio Code 1.37 with extensions
- Python 2019.8.30787
- Remote Development 0.16.0
- Python 3.7
- Windows 10 1903
- Ubuntu 18.04 (for WSL and remote Linux)
- Raspbian on Raspberry Pi 3 B+
A typical Python development includes the virtual environment, linting, test, and debug. This tutorial uses:
All of the linters are supported by Python extension of VS Code. More details for linting can be found here.
Installation on Windows
Since Windows 10 is the host VS Code runs in this tutorial, we need to install VS Code on the Windows host.
Install VS Code
- Download the VS Code from here. When the tutorial is written, the latest version is 1.37.
- Double click the downloaded binary, VSCodeUserSetup-x64-1.37.1.exe, to install.
Install VS Code Extensions
VS Code has thousands of thousand extensions; the Python and Remote Development extensions are the minima for Python development on Windows and remote platforms.
- Launch VS Code.
- Click the extension icon
or type Ctrl+Shift+X
to open the extension windows. - On the search bar, type Python and install it.
- On the search bar, type Remote Development and install it. Remote Development extension also installs Remote – Containers, Remote – SSH, Remote – SSH: Editing Configuration Files, Remote – SSH: Explore, and Remote – WSL.
After the installation, VS Code should look like:

Setup Python Development Environment on Windows
To run Python code on Windows, we need to install Python.
Install Python
Python can be installed by 1. Microsoft Store, or 2. Python official website. Follow the instructions to install it. The latest stable version is 3.7.4 when this article is written.
Create a Virtual Environment
Assume we use C:\Workspace as the workspace for this tutorial and working_environment
is our virtual environment.
cd C:\Workspace
C:\Workspace>python -m venv working_environment
Now we are ready to create the project with VS Code.
Step 1: Launch the VS Code
Step 2: File -> Open Folder
Step 3: Create a new folder called project
under C:\Workspace\
Step 4: Add folder and files shown at Plot, so it looks like:

Once we add a Python file, VS Code automatically detects the Python environment; by default, it picks the system one.

It may also detect something is not installed, e.g., pylint
and pytest
.

Ignore them for now, since we do not want to install these to the system Python interpreter.
Step 5: We want to use the virtual environment , working_environment
, we just created for this project. To do so, we need to tell VS Code the path to the virtual environment.
- File -> Preferences -> Settings
- Type
venv path
at the top search bar - Add C:\Workspace

Step 6: Click the Python Interpreter icon to select the working_environment
. working_environment
should be available now.
a. Click the icon:

b. Choose working_environment
:

Note: If it does not show up, restart VS Code may be necessary.
More details about Python virtual environments can be found here.
By default, Pylint
is enabled for Python in VS Code, but VS Code supports many other linters. This section demonstrates some of the linting setups that I use daily.
- File -> Preferences -> Settings and type linting at the top search bar. Many linter options will show up.

Enable and Install pydocstyle
- Check the box of
pydocstyle
option.

- As soon as we check the box, an install option pops up. Click it to install
pydocstyle
.

- If we want to add non-default options for
pydocstyle
, click the Add Item button.

Add the argument we want. For example, if we want to use numpy style, add --convention=numpy
.

- And click OK button. It will look like:

Note: The complete arguments of pydocstyle
can be found at http://www.pydocstyle.org/en/4.0.0/usage.html#command-line-interface.
Enable and Install mypy
- Check the box of
mypy
option.

- Install
mypy
.

Enable and Install pep8
Do the same thing as the previous step to enable and install pep8
.
- Check the box of
pep8
option.

- Install
pep8
.

Note: All the linters are installed on the working_environment
.
More details of VS Code with Linting can be found here.
Testing and Debugging
For the setups of testing and debugging, VS Code has very comprehensive documents:
Now, we should have a working environment for our Python projects with VS Code.

The VS Code Remote – SSH extension allows us to open a remote folder on a remote machine with a running SSH server and take full advantage of VS Code such as auto-completion, debugging, and linting.

Assumption: This section assumes that we have a remote Linux (Ubuntu 18.04) with SSH enabled.
Step 1: Install OpenSSH Client on the Windows 10 Host
To install OpenSSH, do the following:
Settings -> Apps -> Apps & features -> Optional features -> Optional features -> Add a feature (if OpenSSH client is not already installed). Find OpenSSH client and click ‘’Install’’. After the installation completes, it should look like:

More options for OpenSSH can be found here.
Step 2: Setup the SSH Key
On the Windows 10 host, launch Command Prompt and do the following steps:
- Generate an SSH key pair. And accept all the default value.
C:\Users\user> ssh-keygen -t rsa -b 4096
- Add the contents of the local public key (the id_rsa.pub) to the appropriate authorized_keys file on the SSH host, i.e., the remote Linux).
C:\Users\user> SET REMOTEHOST=your-user-name-on-host@host-fqdn-or-ip-goes-here
- Copy the local public key to the remote Linux.
C:\Users\user> scp %USERPROFILE%\.ssh\id_rsa.pub
%REMOTEHOST%:~/tmp.pubssh %REMOTEHOST% "mkdir -p ~/.ssh &&
chmod 700 ~/.ssh && cat ~/tmp.pub >> ~/.ssh/authorized_keys &&
chmod 600 ~/.ssh/authorized_keys && rm -f ~/tmp.pub"
Step 3: Connect to the Remote Linux Host
- Launch VS Code on Windows 10
- Click the remote development icon
and click the Configure an SSH host. Or type Ctrl+Shift+P to start Command Palette and type Remote-SSH: Connect to Host… and select Configure SSH Hosts…

- Choose the appropriate config file. It may look like C:\Users\user\.ssh\config.
- Edit the config file by adding the Linux host information. The config may look like:

- Click the name of the remote machine (in this example, its name is
remote-ubuntu
) to connect to the remote machine.

- A new window of VS Code will open and ask the user to enter the password.

- Once the connection is established. The icon
will be shown. - Install Python extension on the remote Linux. The Python extension has been installed on the Windows host, but in order to provide all Python features that VS Code provides, we need to install the Python extension on the remote Linux.
- Click the extension icon
. It should show something like the picture below:

The bottom section indicates the extensions installed on the local machine, i.e., Windows 10 in this example. The top section indicates the extensions installed on the remote machine, i.e., Ubuntu 18.04 in this case. Now the remote machine does not have any extension installed.
- To install the Python extension, click the install button
. - Once the installation completes, the extension window becomes:

Step 4: Setup the Project
On the Remote Linux
Now, we can create a virtual environment and a Python project on remote Linux.
On the Windows Host
- If we try to open a folder by File -> Open Folder. It will show the filesystem on the remote Linux. Select the project folder created above.

- Repeat the steps mentioned in the section: Create the Project and Setup VS Code.
Note: The settings we changed for Windows are per user. Here, we should change the settings for Remote.

Now, VS Code should be ready for Python development on the remote Linux machine.

More details of remote development with VS Code can be found here.
Setup Python Development Environment on Raspberry Pi via SSH
The remote development setup on Raspberry Pi is the same as regular Linux such as Ubuntu. However, the current remote development of VS Code does not support ARM architecture. Raspberry Pi 3 is ARM architecture. Therefore, to do the remote develop via SSH on Raspberry Pi, we need to use Visual Studio Code – Insiders.
Download and Install Visual Studio Code – Insiders
- Download VS Code – Insiders from https://code.visualstudio.com/insiders/
- Follow the instructions to install VS Code – Insiders
Once the VS Code – Insiders is installed. Follow the steps mentioned at Setup Python Development Environment on Linux via SSH. The steps are the same. The only difference is the remote Linux machine becomes Raspbian on Raspberry Pi.
The user experience for VS Code – Insiders with Raspberry Pi should be exactly the same as VS Code with remote Linux.

Setup Python Development Environment with WSL
The Windows Subsystem for Linux brings developers the Linux environment experience directly on Windows without using a virtual machine. With the Remote Development extension of VS Code, we can enjoy the full features of VS Code, and develop, run and debug Linux-based applications on Windows.

Step 1: Enable and Install WSL
Follow the instructions to enable and install WSL: https://docs.microsoft.com/en-us/windows/wsl/install-win10
Step 2: Create a Virtual Environment and the Project
From the WSL command line, issue the following commands:
Step 3: Connect to WSL from VS Code
- Launch VS Code.
- Type Ctrl+Shift+P to start Command Palette and type Remote-WSL: New Window.

- After select Remote-WSL: New Window, a new VS Code window will show up and connect to the WSL. On the left bottom, this icon
indicates VS Code has connected to the WSL. - Install Python extension on the WSL. Same as Remote SSH, we need to install the Python extension on the WSL. Click the button to install.

Step 4: Setup the Project
Once the VS Code connects to the WSL, we should be able to setup the Python project as we setup the project for Windows and remote Linux.
- Open the project folder.

- Repeat the steps mentioned in the section: Create the Project and Setup VS Code.
Note: same as Remote SSH, for WSL, we should change the settings under Remote [WSL: Ubuntu-18.04].

Now, VS Code should be ready for Python development with the WSL.

More details of remote develop with WSL can be found here.
Conclusion
VS Code is highly extensible and configurable through extensions and settings, so developers can add features via extensions and configure VS Code to enhance our workflow. In additional to Python and Remote Development extensions, here is the list of extensions I think useful and use daily.
Although this tutorial uses Windows 10 as the host for VS Code, VS Code can be installed and run on Linux and Mac as well. The setup described in the article can be applied to Linux and Mac. Hopefully, you found this article useful.
(This post appeared first on Shun's Vineyard.)
History
- 8th September, 2019: Initial version
My name is Shun. I am a software engineer and a Christian. I currently work at a startup company.
My Website: https://formosa1544.com
Email: shun@formosa1544.com