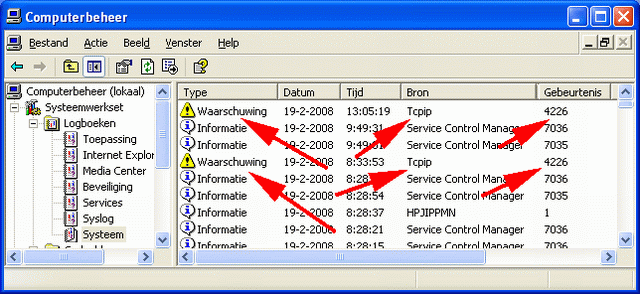
Introduction
When SP2 for Windows XP is installed, the event-log can show several (harmless) 4226 events generated by the TCPIP source. The symbolic name for this event is EVENT_TCPIP_TCP_CONNECT_LIMIT_REACHED
and the message in English is "TCP/IP has reached the security limit imposed on the number of concurrent (incomplete) TCP connect attempts." There is a lot of information on the Internet, but there are no programs to monitor the process responsible for generating this event. This article describes a simple program, which can be run in the background, to catch the 4226 event, and shows the information needed to discover the program, and TCPIP connections responsible for this event to occur.
Background
TCP/IP is connection oriented. To establish a connection between 2 hosts, your PC sends a SYN (synchronize) bit to the other computer. Your PC then waits to receive an ACK bit, or acknowledgement bit from the opposite end. Finally, your PC sends another bit to tell the other computer which sequence number to begin with. When all of this hand shaking is complete, data begins to flow.
The TCP/IP stack in Windows XP with Service Pack 2 (SP2) installed limits the number of concurrent, incomplete outbound TCP connection attempts (SYN_SENT
). When the limit (max. 10) is reached, subsequent connection attempts are put in a queue and resolved at a fixed rate so that there are only a limited number of connections in the incomplete state. During normal operation, when programs are connecting to available hosts at valid IP addresses, no limit is imposed on the number of connections in the incomplete state. When the number of incomplete connections exceeds the limit, for example, as a result of programs connecting to IP addresses that are not valid, connection-rate limitations are invoked, and this event is logged.

Establishing connection–rate limitations helps to limit the speed at which malicious programs, such as viruses and worms, spread to uninfected computers. Malicious programs often attempt to reach uninfected computers by opening simultaneous connections to random IP addresses. Most of these random addresses result in failed connections, so a burst of such activity on a computer is a signal that it may have been infected by a malicious program.
In my opinion, this limit is a good thing. The connection speed is not limited. Also the maximum 'successful' connections is not limited. Because most information on the Internet is wrong, a lot of people patch their TCPIP stack to overcome this limitation. There are only a few situations when this is useful, and those programs are better run on a Unix like machine. Trust me on this one, don't change it. (See Points of Interest section below.)
Using the Code
The program is a simple monitor program. When started, it shows only on output window. You have to iconify it to let it do its job. Because the 4226 event is only generated once in a while, you have to look at it to see if there is something logged.
The program has one generic class which does all the work. It is called LogMonitor
:
public partial class Form1 : Form
{
private LogMonitor lm;
public Form1()
{
InitializeComponent();
lm = new LogMonitor(this.textBox1);
}
}
All logs are written to a textbox
. The constructor of LogMonitor
sets up an eventhandler
which is called whenever a System
event occurs.
public class LogMonitor
{
private const string TCPIP = "Tcpip";
private const int FTTS = 4226;
private EventLog eventLog;
private TextBox textBox;
public LogMonitor(TextBox textBox)
{
this.textBox = textBox;
eventLog = new EventLog("System", ".");
eventLog.EntryWritten += new EntryWrittenEventHandler(OnEntryWritten);
eventLog.EnableRaisingEvents = true;
Log("Logging started.\r\n");
}
private delegate void LogDelegate(string s);
private void Log(string s)
{
if (this.textBox.InvokeRequired)
{
this.textBox.Invoke(new LogDelegate(Log), new object[] { s });
}
else
{
this.textBox.AppendText(s);
}
}
~LogMonitor()
{
eventLog.Close();
}
}
The OnEntryWritten
routine filters the event on Source
and Id
:
private void OnEntryWritten(Object source, EntryWrittenEventArgs e)
{
if (string.Compare(e.Entry.Source, TCPIP, false) != 0)
return;
int intId = (int)(e.Entry.InstanceId & 0xffff);
if (intId != FTTS)
return;
StringBuilder sb = new StringBuilder();
sb.AppendLine("Written: " + e.Entry.Message);
sb.AppendLine("Source: " + e.Entry.Source + " id: " + intId);
sb.Append(DecodeList(ProcessList(), Netstat()));
Log(sb.ToString());
}
When the event matches Tcpip
and 4226
, it gets the current processlist
(all running programs), and it looks for all the TCPIP connections and dumps the combined information.
Getting a list of all the running programs is easy. I store the names and process-ids into a dictionary
:
private Dictionary<string, string> ProcessList()
{
Dictionary<string, string> dictionary = new Dictionary<string, string>();
foreach (Process p in Process.GetProcesses())
{
string strKey = p.Id.ToString();
if(!dictionary.ContainsKey(strKey))
dictionary.Add(strKey, p.ProcessName);
}
return dictionary;
}
For getting a list of all connections, I use the good old DOS program called netstat
. It is executed and the standard output is redirected to use the information in the program.
private string Netstat()
{
Process p = new Process();
p.StartInfo.UseShellExecute = false;
p.StartInfo.RedirectStandardOutput = true;
p.StartInfo.CreateNoWindow = true;
p.StartInfo.FileName = "netstat";
p.StartInfo.Arguments = "-o";
p.Start();
string strNetstat = p.StandardOutput.ReadToEnd();
p.WaitForExit();
return strNetstat;
}
Combining the information of Netstat
and the processlist
, we can now see what has happened, causing the 4226 event.
private string DecodeList(Dictionary<string, string> dictionary, string strNetstat)
{
Regex regex = new Regex(
@"(?<proto>[^\s]*)\s+(?<local>[^\s]*)\s*
(?<remote>[^\s]*)\s+(?<status>[^\s]*)\s+(?<pid>[^\s]*)\s*",
RegexOptions.IgnorePatternWhitespace | RegexOptions.Compiled);
StringBuilder sb = new StringBuilder();
foreach (Match m in regex.Matches(strNetstat))
{
string strPid = m.Groups["pid"].Value;
string strName = strPid;
if (dictionary.ContainsKey(strPid))
strName = dictionary[strPid];
sb.AppendFormat("{0}\t{1}\t{2}\t{3}\t{4}\t({5})\r\n",
m.Groups["proto"].Value,
m.Groups["local"].Value,
m.Groups["remote"].Value,
m.Groups["status"].Value,
m.Groups["pid"].Value,
strName);
}
return sb.ToString();
}
After waiting a few hours, surfing the Internet, some badly configured Web sites tend to generate our event. Here is my output from a few hours of Web browsing:

As you can see, the event is generated by Internet Explorer (IExplore) navigating to a badly configured Web site. In this case, it was a popular Dutch social Web site (www.hyves.nl). No big deal. But we can write a letter to the system administrators, because they caused an event entry 4226 in our logfiles.
Points of Interest
Because there is a lot of fuzz on patching TCPIP.SYS for the 4226 event, I want to give some background information.
Before SP2 is installed on Windows XP, the maximum half open connections were practically unlimited. This gave writers of viruses and other malware the opportunity to write code which attacked others at a maximum possible rate, building up connections, even trying on non existing hosts. This is bad.
Does Patching Help?
Most modern p2p programs (uTorrent, Emule, etc.) have a built-in limit matching those of Windows SP2. Why? The answer is simple, the download speed depends NOT on the half-open connection limit. It depends solely on the upload speed of the peer host or hosts.
When uploading in a p2p network, your host (router or PC behind the router) gets numerous connection attempts. Most routers have security detection mechanisms, one of them is 'tcp_syn_flood
' which prevents a 'denial of service' of the router. In normal words, due to a lot of connection attempts, the router freezes, and your internet connection is lost. So normal (unpatched) tcpip.sys can help to make a better world, not freezing your friends routers.
Most people who patch tcpip.sys do increase the limit of their Windows OS. But they all forget to look at their router, which in most cases, also has a decent limit. Increasing the Windows limit can also cause freezes of your own router.
Unpatched tcpip.sys helps to make trackers do their job more efficiently. By not acknowledging a 'syn
', because the tracker is under heavy load, the p2p client can choose another to get its information from. Patched tcpip.sys however targets all possible trackers, the load on the servers increases when the information is not needed in the end.
And remember, all connections are put on a queue and resolved at a fixed rate, so they are not lost!!
The program presented in this article has been used for months and was written because there is so much mis-information on the Internet. I did not see any proof that a patched tcpip.sys does give faster downloads. Popular p2p programs like eMule and uTorrent do their job fantastically, without a patched tcpip.sys.
If there is any real theoretical background why the limit should be increased, please do let me know. Most of the information I found on the Internet, was incorrect.
History
- 19th February, 2008: Version 1.0.0.0
I'm Alphons van der Heijden, living in Lelystad, Netherlands, Europa, Earth. And currently I'm retiring from hard working ( ;- ), owning my own company. Because I'm full of energy, and a little to young to relax ...., I don't sit down, but create and recreate software solutions, that I like. Reinventing the wheel is my second nature. My interest is in the area of Internet technologies, .NET etc. I was there in 1992 when Mosaic came out, and from that point, my life changed dramatically, and so did the world, in fact. (Y)