Today, I helped a client to solve a problem regarding EF4.1 Code First. 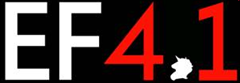
The issue that they banged their heads with was an inheritance tree mapping in their model which is spread across two different assemblies. When they run the application, they always got the same exception – “mapping and metadata information could not be found for entitytype ‘entity name’”. So they sent me a sample solution and I checked it and gave them the solution.
The Client Provided Model
In one class library, there are the following two classes:
namespace CodeFirstTest
{
public class Base
{
public int Id { get; set; }
}
public class DeriveB : Base
{
}
}
In another console application, there is another derived class for the Base
class:
namespace CodeFirstTest
{
public class DeriveA : Base
{
}
}
Also, in the same console application, they have created the context:
namespace CodeFirstTest
{
public class TheContext : DbContext
{
public TheContext()
{
}
public DbSet<Base> MyModel { get; set; }
}
}
Running the following code will produce the “mapping and metadata information could not be found for entitytype
‘entity name’” exception:
namespace CodeFirstTest
{
class Program
{
static void Main(string[] args)
{
Database.SetInitializer<TheContext>(
new DropCreateDatabaseIfModelChanges<TheContext>());
using (TheContext ctx = new TheContext())
{
var obj1 = new DeriveA();
ctx.MyModel.Add(obj1);
var obj2 = new DeriveB();
ctx.MyModel.Add(obj2);
obj2 = new DeriveB();
ctx.MyModel.Add(obj2);
ctx.SaveChanges();
}
using (TheContext ctx = new TheContext())
{
var res = ctx.MyModel.Where(b => b.Id == 2).SingleOrDefault();
}
}
}
}
The Solution
First, I reproduced the exception and then tried to figure out what might be the mapping error that they get. Since the problem is in the entity type mapping, it must be caused by things like:
- Type mismatches
- Misspelled properties
- Properties that are missing
- More
The solution to the problem is to add mapping to all the entities in the context. In order to do that, you will have to override the OnModelCreating
method and map all the entities:
protected override void OnModelCreating(DbModelBuilder modelBuilder)
{
base.OnModelCreating(modelBuilder);
modelBuilder.Entity<Base>();
modelBuilder.Entity<DeriveA>();
modelBuilder.Entity<DeriveB>();
}
This solves the problem and produces the relevant database when the console application is running.
Summary
It took me a short period of time in order to find the mapping problem that my client had. The solution for the problem is simple but I would expect that Code First will supply the solution within. Maybe in vNext…
Gil Fink is a web development expert and ASP.Net/IIS Microsoft MVP. He is the founder and owner of sparXys. He is currently consulting for various enterprises and companies, where he helps to develop Web and RIA-based solutions. He conducts lectures and workshops for individuals and enterprises who want to specialize in infrastructure and web development. He is also co-author of several Microsoft Official Courses (MOCs) and training kits, co-author of "Pro Single Page Application Development" book (Apress) and the founder of Front-End.IL Meetup. You can read his publications at his website: http://www.gilfink.net