Introduction
I am going to start a series of posts building a simple app using the Chinook database as a back-end and putting a WPF client on the front-end. I am going to keep it loose as I don’t want to commit to blog posts that I may not get a chance to complete so I will keep every the post self-contained covering one detail of the implementation.
The technologies and tools I plan on using for this series are as follows:
As you can see, the idea is to use the latest technologies available to build a simple app to display the music details from the Chinook database. As I am not committing to a large series, I will say that my intentions for the series include:
- Setting up the model using EF4 Code First (this post)
- Creating a basic screen listing the artists and associated albums
- Creating an album details adorner to show the tracks of individual albums
Possible future posts will include:
- Adding the ability for customers to place an order (creating invoices)
- Adding employees to manage the customer invoices (this is a WPF app so the employee will be doing all of the work as this is not a customer facing app)
- Adding the ability to create custom playlists that can reviewed
Note that all of these items already exist in the Chinook database and I will be focusing on the data access and UI layers.
Building the Model using EF Code First
The first thing we need to do is create a new solution for our app. Being as we are creating the model first I am going to create a class library.
Once the solution and project are in place, we need to get the Code First bits into the project. I am going to use NuGet so you need to have this installed.
In the NuGet package manager window, we can find the required package by firing the command:
Get-Package –remote –filter CodeFirst
This will give us the following output:
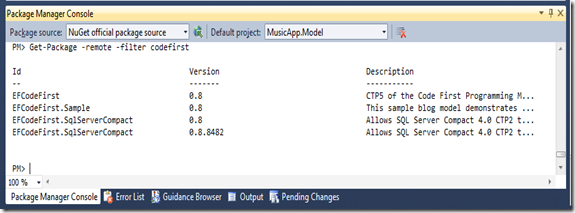
This shows us the package that we need to install is EFCodeFirst
so we issue:
Install-Package EFCodeFirst
This should add the EntityFramework
reference to your project but it didn’t do that on my class library so I had to add the reference manually ({SolutionFolder}\packages\EFCodeFirst.0.8\lib\EntityFramework.dll).
Now we have the reference we can start to create our POCO objects. I am going to create a model that initially uses the Artist
, Album
and Track
tables as they are going to be used in the first part of this app – I will add more as I go along.
EF Code First allows us to create standard POCOs to represent our model so for my initial model I have:
public class Artist
{
public int ArtistId { get; set; }
public string Name { get; set; }
public virtual ICollection<Album> Albums { get; set; }
}
public class Album
{
public int AlbumId { get; set; }
public string Title { get; set; }
public virtual Artist Artist { get; set; }
public virtual ICollection<Track> Tracks { get; set; }
}
public class Track
{
public int TrackId { get; set; }
public string Name { get; set; }
public virtual Album Album { get; set; }
public string Composer { get; set; }
}
Note the virtual association properties – this is to allow these properties to be lazy-loaded.
The next thing we need is the actual dbContext
which is defined as follows:
using System.Data.Entity;
using System.Data.Entity.ModelConfiguration.Conventions.Edm.Db;
.
.
.
public class Chinook : DbContext
{
protected override void OnModelCreating(
System.Data.Entity.ModelConfiguration.ModelBuilder modelBuilder)
{
modelBuilder.Conventions.Remove<PluralizingTableNameConvention>();
}
public DbSet<Artist> Artist { get; set; }
public DbSet<Album> Album { get; set; }
public DbSet<Track> Track { get; set; }
}
This is a class that includes one property per table in the database and handles the mapping to our objects. This is using default conventions with the exception of the one removed in the overridden OnModelCreating
method. This says that the database we have doesn’t have pluralised names as Code First expects and instead the names match the entities.
Next, we need to add an app.config file to the project and add the ConnectionString
for the database. I use SQLServer so adjust accordingly.
<connectionStrings>
<add name="Chinook" providerName="System.Data.SqlClient"
connectionString="Data Source=.;Initial Catalog=chinook;Integrated Security=true" />
</connectionStrings>
Notice that the name of the connectionString
must be the same as the class name when using the default conventions.
Testing That It Works
OK, we have the model built now and can run a crude test to see if it works. Add a console app to your solution and set it as the startup project. Add references to the Model
assembly and the Code First assembly and move the app.config to the new project. Now add the following code to the main
method.
static void Main(string[] args)
{
using (Chinook db = new Chinook())
{
foreach (var artist in db.Artist)
{
Console.WriteLine(artist.Name);
}
Console.ReadLine();
}
}
This will loop through all of the artists and print their name to the screen.
That’s all there is to creating a simple model with Entity Framework Code First CTP5. In the next instalment, I plan on writing a simple data provider class that will abstract the actual DbContext
calls away from the user and allow the user to simply call methods like provider.GetArtists()
.
I am a developer currently working for a financial company in the UK with a focus on back end work using NServiceBus. Personally I also enjoy working with ASP.NET (MVC), WPF, ruby and investigating best practice using methods like TDD and bettering the quality of code.