Introduction
An Azure Function is an entirely serverless way of having common functionality available to your applications via the cloud without the overhead of managing virtual machines or configurations, etc. You only pay for the actual execution time spent and all of the scaling is taken care of for you.
Background
This example is a piece of functionality that is often used in financial systems which validates a CUSIP which is a special 9-digit identifier used to uniquely identify a financial security traded in the North American markets. The last digit of this number is a check sum digit used to check if the CUSIP is valid or not.
Since this validation is idempotent and requires no external storage, it is an ideal candidate for an Azure function.
Creating a New Azure Function
You create new functions in the Azure portal in the "App Services" section:
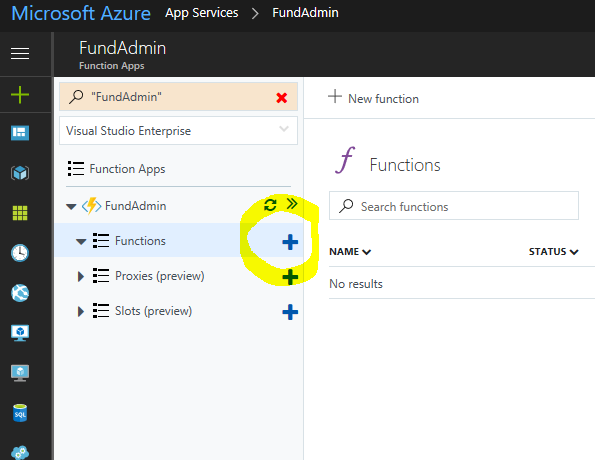
When you select this button, a quick-start screen allows you to select a language and starter template.

For this example, an API written in C# is selected.
The Function Wrapper (run.csx)
The template creates a wrapper that takes care of the connection and you then have to write the code that takes the inputs and returns an appropriate HTTP response.
#load "cusip.csx"
using System.Net;
public static async Task<HttpResponseMessage> Run(HttpRequestMessage req, TraceWriter log)
{
log.Info("C# HTTP CUSIP validation function");
string cusip = req.GetQueryNameValuePairs()
.FirstOrDefault(q => string.Compare(q.Key, "cusip", true) == 0)
.Value;
dynamic data = await req.Content.ReadAsAsync<object>();
cusip = cusip ?? data?.cusip;
if (IsValidCusip(cusip))
{
log.Info("Valid CUSIP : " + cusip);
return req.CreateResponse(HttpStatusCode.OK, "true");
}
else
{
log.Info("Invalid CUSIP : " + cusip);
return req.CreateResponse(HttpStatusCode.OK, "false");
}
}
In this case, we just want to return "true
" if the CUSIP is valid and "false
" if it is not.
Custom (Shared) Functions
If you have functionality that you want to share between different Azure functions, you can have it in a separate code file that you can then reference in your Azure function. You need to use the #load
command to reference this in your function wrapper.
public static bool IsValidCusip(string cusip)
{
if (string.IsNullOrWhiteSpace(cusip))
{
return false;
}
if (cusip.Length == 9)
{
int checkDigit = getExpectedCheckDigit(cusip);
int expectedCheckDigit = (int)Char.GetNumericValue(cusip[8]);
return (checkDigit == expectedCheckDigit);
}
return false;
}
Triggering the Azure Function
To run the Azure function, you use the URL in the form https://{function app name}.azurewebsites.net/api/{function name} with any parameters passed in either in the query string or in the request body - for example, you could use https://fundadmin.azurewebsites.net/api/CUSIPValidation?cusip=00846U101.
History
- 17th August, 2017 - First version