Introduction
Let’s first establish what the purpose of code is in the first place.
For this article, the purpose of code is how to handle exceptions in MVC, different ways to handle exceptions in MVC and which way is the most suitable in which condition.
** Simple Approach by using Try...Catch Block **
It is the most basic level of Exception handling. Is is also called Method level exception handling.
What is Try Catch Block ?
The try
block contains a block of code within which an exception might occur. Catch
block will catch the exception which will occur in try
block.
Syntax of Try
...Catch
:
try
{
}
catch(ExceptionType ObjectName)
{
}
public ActionResult EXMethodApproch()
{
try
{
object obj = null;
string myStr = obj.ToString();
return View();
}
catch(NullReferenceException)
{
return View("Your Custom Error Page");
}
catch(DivideByZeroException)
{
return View("Your Custom Error Page");
}
}
If you use some External Dll / External Controls and you are not sure for its functionality, then you can use Method level (try
...catch
) exception handling.
The main disadvantage of using this method is that error handling login can't be reused.
** Global Level Exception Handling / "HandleError" Attribute **
What is HandleError Attribute?
HandleErrorAttribute
is used to handle an exception which is thrown by ActionMethod
and display friendly error pages when there is an unhandled exception.
- Add the below Action Method in Home controller:
public ActionResult EXHandleErrorAttributeApproch()
{
throw new Exception("Error While Processing");
}
Run this page, we can find an exception as we did not handle exception in our code.
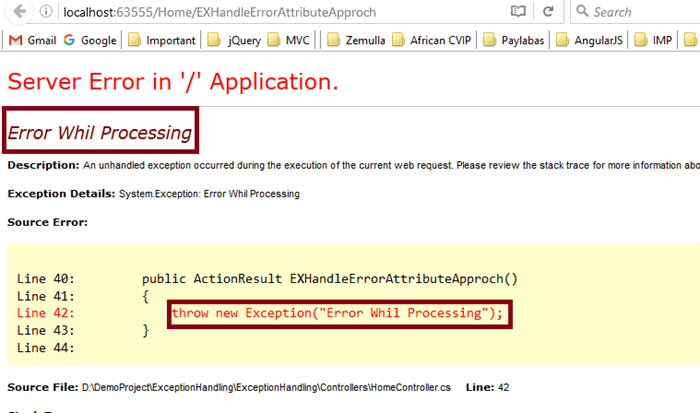
Now, we understand how to display friendly error pages.
- Set
[HandleError]
Attribute on top of HomeController
, for unhandled exceptions arising in any of its action methods are handled by it.

Note: HandleError Attribute required customErrors mode="On" in your web.config File.
- Add FilterConfig.cs class in "App_Start" folder and Add "
RegisterGlobalFilters
" method as per below code.
public static void RegisterGlobalFilters(GlobalFilterCollection filters)
{
filters.Add(new HandleErrorAttribute());
}

- Now, set
RegisterGlobalFilters
method in Global.asax.cs file. Paste the below statement in Global.asax.cs file.
FilterConfig.RegisterGlobalFilters(GlobalFilters.Filters);

- Enable Custom Error Mode in Web.config. Add the below code in your web.config:
<customErrors mode="On">
</customErrors>
After this, instead of silly yellow page, we just found two statement as below image:

- Run the project and enter a wrong URL. (Here, we enter wrong
ActionResult
name so 404 {The resource cannot be found} Exception Occurs)

- Add Error Page (for 404 Error Code):
public ActionResult ErrorPage404()
{
return View();
}
Now, reference this page to customErrors
in Web.config file.
<customErrors mode="On">
<error statusCode="404" redirect="~/Home/ErrorPage404"/>
</customErrors>
Run this page, and again go through step 6. Now we found the custom (friendly) error page.

** custom exception filter by extending HandleErrorAttribute class **
Add new class as "CustomErrorHandler
", and paste the below code in that class.
public class CustomErrorHandler : HandleErrorAttribute
{
public override void OnException(ExceptionContext exceptionContext)
{
if (!exceptionContext.ExceptionHandled)
{
string controllerName = (string)exceptionContext.RouteData.Values["controller"];
string actionName = (string)exceptionContext.RouteData.Values["action"];
var model = new HandleErrorInfo(exceptionContext.Exception, controllerName, actionName);
exceptionContext.Result = new ViewResult
{
ViewName = "ActionName of CustomError Page Name",
ViewData = new ViewDataDictionary<HandleErrorInfo>(model),
TempData = exceptionContext.Controller.TempData
};
}
}
}
Now, add CustomErrorHandler
to Filter Config:
filters.Add(new CustomErrorHandler());

So, we define our custom exception handler for handle exception.
Now just Add [CustomErrorHandler]
on top of your ActionResult
Method or controller (if you want to apply in all ActionResult
Method) .
[CustomErrorHandler]
public ActionResult TestCustomHandler()
{
return View();
}