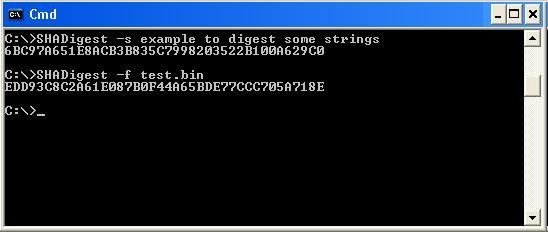

Contents
- Introduction
- Overview
- Using CSHADigest Class
- Using SHADigest Program
The CSHADigest
class has been designed to be fast and to be easily integrated in applications on independent platforms. This class respects the SHA1 digest 160 bits definitions.
Note: The demo project works on UNIX and Win platforms.
class CSHADigest
{
public:
CSHADigest();
virtual ~CSHADigest();
int ComputeInputFile(const char filePathName[]);
void Start();
int ComputeInputBuffer(const uByte* buffer, uInt32 bufferSize);
void Stop();
uByte* GetByteResult();
void GetByteResult(uByte* byteResult);
char* GetHexResult();
void GetHexResult(char* hexResult);
static uInt32 GetFileSize(const char filePathName[]);
static void ConvertByteResultToHexResult(const uByte* byteResult,
char* hexResult);
protected:
struct SSHAContext
{
uInt32 intermediateHash[5];
uInt32 lengthLow;
uInt32 lengthHigh;
uInt16 messageBlockIndex;
uByte messageBlock[64];
};
SSHAContext SHAContext;
void PadMessage();
void ProcessMessageBlock();
uByte byteResult[20];
char hexResult[41];
};
How to digest a string
void Start();
int ComputeInputBuffer(const uByte* buffer, uInt32 bufferSize);
void Stop();
Step 1: Create an instance of CSHADigest
class.
CSHADigest SHADigest;
Step 2: Use Start()
to initialise the digest processes.
Step 3: Call ComputeInputBuffer()
each times it's necessary. Return 0 if an error has been detected, else 1.
Step 4: When you have finished and you want to compute the results, call Stop(
).
The get digest result call:
char* GetHexResult()
(The SHA Digest is coded on 40 chars in Hex format.)
or
uByte* GetByteResult()
(The SHA Digest is coded on 20 bytes in Binary format.)
View in the SHADigest.cpp file the main function, to have a good example.
Warning: The SHA Digest result returned by GetByteResult()
(or GetHexResult()
) is valid only during the existence of the SHADigest
instance. Preferably use GetByteResult(uByte* byteResult)
(or GetHexResult(char* hexResult)
) in the other cases.
How to digest a file
int ComputeInputFile(const char filePathName[]);
Step 1: Create an instance of CSHADigest
class.
CSHADigest SHADigest;
Step 2: Just call ComputeInputFile()
with the valid filepath as argument. Return 0 if an error has been detected, else 1.
The get digest result call:
char* GetHexResult()
(The digest is coded on 40 chars in Hex format.)
or
uByte* GetByteResult()
(The digest is coded on 20 bytes in Binary format.)
View the SHADigest.cpp file to have a good example.
Warning: The SHA Digest result returned by GetByteResult()
(or GetHexResult()
) is valid only during the existence of the SHADigest
instance. Preferebly use GetByteResult(uByte* byteResult)
(or GetHexResult(char* hexResult)
) in the other cases.
On Windows platform

Compile and copy the SHADigest.exe in [root drive]\windows\System32\ folder.
Now, starts a new instance of the command interpreter (cmd.exe):
Execute SHADigest
with the following parameters :
To compute the SHADigest of a file:
SHADigest -f FilePathName (or just SHADigest FilePathName)
To compute the SHADigest of a string:
SHADigest -s string
To compute the SHADigest of some strings:
SHADigest -s string1 string2 [...] stringN
On Unix platform

To compile the program type make. To copy the SHADigest in /usr/bin/ folder, just type make install. (You must be logged in as root.)
Now, start a shell. Execute SHADigest with the following parameters:
To compute the SHADigest of a file:
SHADigest -f FilePathName (or just SHADigest FilePathName)
To compute the SHADigest of a string:
SHADigest -s string
To compute the SHADigest of some strings:
SHADigest -s string1 string2 [...] stringN
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.