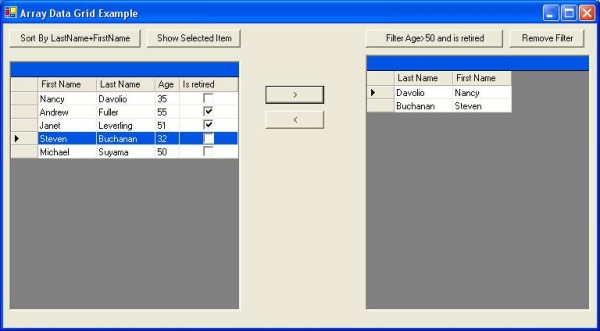
Introduction
System.Windows.Forms.DataGrid
is a great control with so many features, but I think that it's a little bit hard to use it. So I wrote a user control that uses a data grid, but it's a little bit easier to use. Here are its features:
- It gets an array of objects as its data source
- It returns its selected item as an object
- It returns its selected items as an array of objects
- You can format it very easily
- You can add an object to its datasource
- You can remove an object from its datasource
- You can filter its datasource
- You can sort its datasource
How it works
Setting the datasource
You can set its datasource like this:
myGrid.DataSource=users;
Formatting
You can format the grid using a GridFormatter
object.
A grid formatter is an object that has some column formatter that will tell the grid how to format itself. Each column formatter contains a DataGridColumnStyle
type.
GridFormatter format1=new GridFormatter() ;
format1.AddColumnFormatter("FirstName","FirstName",
typeof(DataGridTextBoxColumn));
If you want to hide a column, just don't add a column formatter to your grid formatter.
When the grid formatter is ready, we set our data grid formatter to let it format itself.
myGrid.GridFormatter=format1;
The good news is that you can change your formatter anytime you like.
Hint: In order to format an empty grid, you can pass an empty array of your object to its datasource and then format it.
Hint: Since
GridFormatter
uses a
DataGridColumnStyle
object to format the grid, you can easily show almost every thing in your grid. (
Here is an article about
DataGridColumnStyle
by
Declan Brennan that I found very interesting).
Adding and removing records
There are times that you want to add or remove records at run time.
You can do it using the AddRow(object obj)
and AddRows(object[] objects)
methods.
myGrid.AddRow(new User("FName","LName"));
And if you want to remove some records, use Remove(object obj)
or Remove(object[] objects)
.
Getting the selected items
You can get the current row (as an object) using the SelectedItem
property.
User selectedUser=(User)myGrid.SelectedItem;
You can get the selected items (as an array of object
s) using the SelectedItems
property.
usersGrid.AddRows((User[])myGrid.SelectedItems);
Filtering and sorting
You can apply a filter to your grid using ApplyFilter(ExpressionBuilder expression)
. Applying the filter takes an Expression Builder to build its filter expression. An Expression Builder is an object that builds a string. For example, if you like to say "FirstName='Fname' And Age>'15'", you will write:
ExpressionBuilder expBuilder =
new AndExpression(new EqExpression("FirstName","FName"),
new GtExpression("Age",15));
It is very similar to the Hibernate/NHibernate expression object model.
There are different expression builders that work together using a Composite pattern, therefore you can combine them to make a complex expression.
Now we have an Expression Builder we can use to filter our grid.
myGrid.ApplyFilter(expBuilder);
To remove the filter, simply use the RemoveFilter()
method.
Hint: If you filter a data grid and add an object that doesn't fit in the applied filter condition, it will be added, but it will be hidden until you remove the filter.
If you want to sort a DataGrid
, you can use an array of Order
objects (again, it's very similar to Hibernate/NHibernate).
An order object takes a column name and a bool value indicating to sort ascending (true
) or descending (false
).
Order[] order=new Order[]{new Order("FirstName",true),
new Order("LastName",false)};
myGrid.Sort(order);
Final word
This is my first article at CodeProject. I hope you find it useful, and please let me know your opinion and comments.
Let me know what other features this grid should have.
See also
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.