Introduction
There are many different ways to count words in front-end webpage, and I would like to show you one of the methods using AngularJS which I find interesting and quite convenient.
More details about AngularJS:
- https://angularjs.org/
- https://docs.angularjs.org/tutorial
Background
Before this article, I have already posted a tip to count characters and set character-limit in Textarea by using AngularJS. Link: http://www.codeproject.com/Tips/897535/AngularJS-Counting-Characters-and-Set-Maximum-Leng
And in this article, before demonstrating how to count words, it is important to give a definition for a <word
>. Therefore, personally, a <word
> is defined as follows:
- It is a string which only comprises of human alphabets.
To standardize the definition, I will use English alphabets in this article, i.e. A-Z, a-z
Refer ASCII code here: http://www.asciitable.com/
Using the code
First of all, we need to create HTML file:
<!DOCTYPE html>
<html>
<title> Angular-102: Counting Words in Textarea </title>
<head></head>
<body>
<p id="sampleText"> Mein Name ist Kleinelefant. Hallo, wie geht's dir! </p>
<div>
<p>You have entered 0/100 characters</p>
<p> You have entered 0/10 words </p>
<Textarea name="TextField" rows=5 cols=50 maxlength="100"> </textarea>
</div>
</body>
</html>
In this HTML file, we have one Textarea which has 5
rows and 50
columns as default, and its maximum number of characters is 100
.
Next, we need to add library of AngularJS and define some AngularJS directives for a WordController
as follows:
<head>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.js"></script>
<script language="JavaScript">
angular.module('AppController', []).controller('WordController', function(){
var wordController = this;
wordController.CharacterLength = 0;
wordController.WORDS_MAXIMUM = 10;
wordController.WordsLength=0;
wordController.Text = "";
wordController.FontStyle={'color':'red'};
wordController.UpdateLengths = function($event)
{
}
});
</script>
</head>
So, in this WordController, we have already defined some attributes:
CharacterLength
: the number of characters in Textarea WORDS_MAXIMUM
: the word limit in Textarea WordsLength
: the number of words in Textarea Text
: the content of Textarea FontStyle
: the display style
And the method UpdateLengths()
which will be used to listen to any change in Textarea so that all related attributes can be updated immediately.
Also, it is very important to check if a character is an alphabet, so the method IsAlphabet()
is defined as:
wordController.IsAlphabet = function(character)
{
var numeric_char = character.charCodeAt(character);
if(numeric_char>64 && numeric_char<91)
{
return true;
}
if(numeric_char>96 && numeric_char<123)
{
return true;
}
return false;
}
Note: you can check ASCII table for more details
Now, we need to connect HTML tags with WordController defined in AngularJS:
1. For HTML tag:
<html ng-app="AppController">
2. For Textarea tag:
<div ng-controller="WordController as wordsController">
<p ng-model="wordsController.CharacterLength" >You have entered <font ng-style="wordsController.FontStyle">{{wordsController.CharacterLength}} </font>/100 characters</p>
<p ng-model="wordsController.WordsLength"> You have entered <font ng-style="wordsController.FontStyle">{{wordsController.WordsLength}}</font>/10 words </p>
<Textarea name="TextField" ng-model="wordsController.Text" ng-change="wordsController.UpdateLengths()" ng-trim="false" rows=5 cols=50 maxlength="100"> </textarea>
</div>
Next, we need to implement the method UpdateLengths() to update the counting numbers of characters and words:
wordController.UpdateLengths = function($event)
{
wordController.CharacterLength = wordController.Text.length;
wordController.WordsLength=0;
if(wordController.Text.length == 1 && wordController.Text[0]!=' ')
{
wordController.WordsLength = 1;
}
for( var i=1; i< wordController.Text.length; i++)
{
if( wordController.IsAlphabet(wordController.Text[i]) && !wordController.IsAlphabet(wordController.Text[i-1]))
{
wordController.WordsLength++;
if(wordController.WordsLength == WORDS_MAXIMUM + 1)
{
wordController.WordsLength--;
wordController.Text = wordController.Text.substring(0, i);
return;
}
}else if (wordController.IsAlphabet(wordController.Text[i]) && wordController.IsAlphabet(wordController.Text[i-1]) )
{
if(wordController.WordsLength==0)
{
wordController.WordsLength=1;
}
}else if(!wordController.IsAlphabet(wordController.Text[i]) && !wordController.IsAlphabet(wordController.Text[i-1]))
{
continue;
}else if(!wordController.IsAlphabet(wordController.Text[i]) && wordController.IsAlphabet(wordController.Text[i-1]))
{
continue;
}
}
}
Finally, the HTML would look like this:
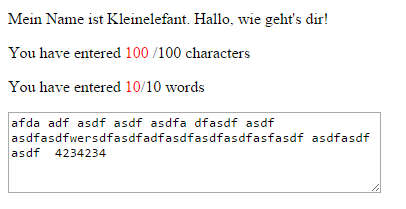
Points of Interest
Let's enjoy learning AngularJS! :)
History
- May 01st, 2015: Initial Release
My hobbies are to learn programming languages, including human languages, and to play sports, including E-sports.
My current expertises:
- Programming languages: C/C++/C#, Java, JavaScript, Ruby
- OS: Windows/Windows Server, Unix, Linux, Android
- Web framework: AngularJS, WCF, ASP.NET, Node.JS, Java Servlet
Note: Travelling is to enrich my life, so I travel very often