Introduction
This little guide helps users to properly configure Hibernate Ant Tools with no requirement of the Eclipse plugin.
Background
You need to download all the Hibernate tools jar provided in the official site.
Using the Code
Create a Java project and call it as you like (in this example, we'll call it HibernateAntGenerator
).
Assign as a source folders this path as described in the window below:
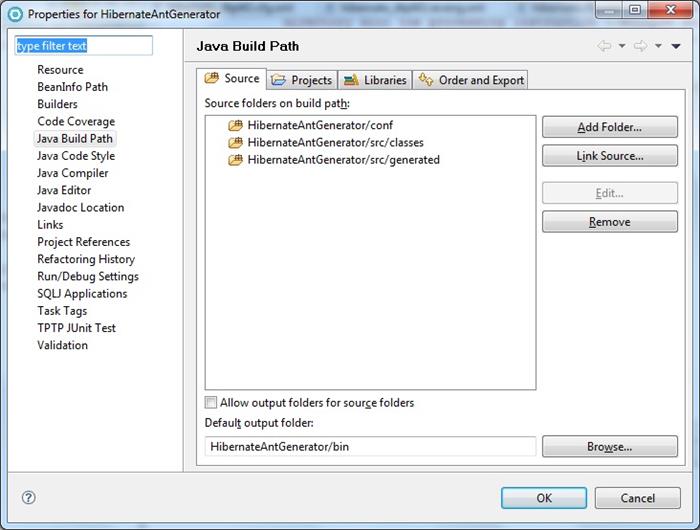
Also, create a folder named lib and put all the Hibernate Tools jar inside, then add these jars in Libraries section.

Once the project is created, create a log4j.properties
inside conf folder and add the following lines:
log4j.rootLogger = ALL, Console
log4j.appender.Console=org.apache.log4j.ConsoleAppender
log4j.appender.Console.layout=org.apache.log4j.PatternLayout
log4j.appender.Console.layout.conversionPattern=%m%n
Now, still in this folder, create a file called hibernate.cfg.xml.
Here, we'll put the database connection configuration required for generating all the objects requested from the tables. Here's an example in DB2:
="1.0"="UTF-8"
<!DOCTYPE hibernate-configuration PUBLIC
"-//Hibernate/Hibernate Configuration DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<property name="hibernate.connection.driver_class">com.ibm.db2.jcc.DB2Driver</property>
<property name="hibernate.connection.password">pws</property>
<property name="hibernate.connection.url">jdbc:db2://host:port/dbname</property>
<property name="hibernate.connection.username">user</property>
<property name="hibernate.default_schema">schema</property>
<property name="hibernate.dialect">org.hibernate.dialect.DB2Dialect</property>
</session-factory>
</hibernate-configuration>
In the same folder, create a file called hibernate.revenge.xml, where the table in which we'll apply the reverse engineering operation are mapped:
="1.0"="UTF-8"
<!DOCTYPE hibernate-reverse-engineering PUBLIC
"-//Hibernate/Hibernate Reverse Engineering DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-reverse-engineering-3.0.dtd" >
<hibernate-reverse-engineering
<table-filter match-name="MY_TABLE" match-schema="MY_SCHEMA"></table-filter>
</hibernate-reverse-engineering>
Now, in the project's root, export an ant build file, which will be created with all the jar's classpath you have included into the project.
Clean all the targets automatically generated and write something like this:
="1.0"="utf-8"
<!DOCTYPE project>
<project basedir="." name="HibernateAntGenerator">
<property environment="env" />
<property name="ECLIPSE_HOME" value="C:/Program Files (x86)/IBM/SDP" />
<property name="debuglevel" value="source,lines,vars" />
<property name="target" value="1.6" />
<property name="source" value="1.6" />
<property name="conf.dir" value="conf" />
<property name="build.dir" value="src" />
<path id="toolslib">
<pathelement location="bin" />
<pathelement location="lib/antlr-2.7.6.jar" />
<pathelement location="lib/asm-attrs.jar" />
<pathelement location="lib/asm.jar" />
<pathelement location="lib/c3p0-0.9.1.jar" />
<pathelement location="lib/cglib-2.2.jar" />
<pathelement location="lib/commons-collections-3.1.jar" />
<pathelement location="lib/commons-logging-1.0.4.jar" />
<pathelement location="lib/concurrent-1.3.2.jar" />
<pathelement location="lib/connector.jar" />
<pathelement location="lib/dom4j-1.6.1.jar" />
<pathelement location="lib/ehcache-1.2.3.jar" />
<pathelement location="lib/hibernate3.jar" />
<pathelement location="lib/jaas.jar" />
<pathelement location="lib/javassist.jar" />
<pathelement location="lib/jboss-cache.jar" />
<pathelement location="lib/jboss-common.jar" />
<pathelement location="lib/jboss-jmx.jar" />
<pathelement location="lib/jboss-system.jar" />
<pathelement location="lib/jdbc2_0-stdext.jar" />
<pathelement location="lib/jgroups-2.2.8.jar" />
<pathelement location="lib/jta.jar" />
<pathelement location="lib/log4j-1.2.15.jar" />
<pathelement location="lib/oscache-2.1.jar" />
<pathelement location="lib/proxool-0.8.3.jar" />
<pathelement location="lib/slf4j-api-1.5.8.jar" />
<pathelement location="lib/slf4j-log4j12-1.5.8.jar" />
<pathelement location="lib/jtidy-r938.jar" />
<pathelement location="lib/swarmcache-1.0rc2.jar" />
<pathelement location="lib/freemarker.jar" />
<pathelement location="lib/hibernate-tools-3.4.0.CR2.jar" />
<pathelement location="lib/db2jcc_license_cisuz.jar" />
<pathelement location="lib/db2jcc_license_cu.jar" />
<pathelement location="lib/db2jcc.jar" />
</path>
<taskdef name="hibernatetool"
classname="org.hibernate.tool.ant.HibernateToolTask"
classpathref="toolslib" />
<hibernatetool destdir="${build.dir}/generated">
<classpath>
<path location="${build.dir}/classes" />
</classpath>
<jdbcconfiguration packagename="test"
configurationfile="${conf.dir}/hibernate.cfg.xml"
revengfile="${conf.dir}/hibernate.reveng.xml"
detectmanytomany="true" />
<hbm2java />
<hbm2dao />
<hbm2cfgxml />
<hbm2hbmxml />
</hibernatetool>
</project>
With these directives...
<hbm2java />
<hbm2dao />
<hbm2cfgxml />
<hbm2hbmxml />
...the builder will generate respectively the Java entities, the DAO (also called Home classes), the hibernate config file with the database and schema to import and finally the mapping for each entity table requested in the generated source folder.
Points of Interest
While the Eclipse plugin is intuitive and easy to use as well, the Hibernate Ant Tools allows the user to work with any IDE Java compatible.