Introduction
Sometimes we need to restrict some values on the client side for entering only text
and digit based on requirements. And also we would see how to disable options
like cut, copy, paste, and drag values from textboxes.
Background
Before we restrict the values we need to create text-boxes. In the code, we can disable the input with different values in the textboxes,
and restrict the end user by using cut, copy, and paste using JavaScript and jQuery.
Using the Code
jQuery
<script type="text/javascript"
src="http://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<script type="text/javascript">
$(function () {
$("#tbxNumber").bind("keypress", function (e) {
var keyCode = e.which ? e.which : e.keyCode
var result = (keyCode >= 48 && keyCode <= 57);
$("#lblMsgNumbers").css("display",
result ? "none" : "inline");
return result;
});
$(".tbxClass").bind("paste", function (e) {
return false;
});
$("#tbxClass").bind("cut", function (e) {
return false;
});
$("#tbxClass").bind("copy", function (e) {
return false;
});
$("#tbxClass").bind("drop", function (e) {
return false;
});
$("#tbxText").bind("keypress", function (e) {
var keyCode = e.which ? e.which : e.keyCode
var result = ((keyCode >= 65 && keyCode <= 90) ||
(keyCode >= 97 && keyCode <= 122));
$("#lblMsgText").css("display",
result ? "none" : "inline");
return result;
});
});
</script>
In the above example using jQuery - on the load event handler I created event handlers to handle events like, keypress, paste, cut, copy paste, and drop on the textboxes.
For keypress event, first I determined the ASCII code with keyboard keys and then verified that its ASCII code exists within the range. And for each textbox,
give a maximum
size of 10 values which can be entered. On cut, copy, paste, and drop events I return false so that the functionality of the textbox would be disabled.
In the below example using JavaScript, the function determines the ASCII code with keyboard keys and then verifies
whether the ASCII code exists within the given range. And each textbox has a size of 10. For cut, copy, paste, and drop events, return false so that the functionality of the textbox would be disabled.
JavaScript
<script type="text/javascript">
function IsDigit(e) {
var keyCode = e.which ? e.which : e.keyCode
var result = (keyCode >= 48 && keyCode <= 57);
document.getElementById("lblMsgNumberJS").style.display =
result ? "none" : "inline";
return result;
}
function IsText(e) {
var keyCode = e.which ? e.which : e.keyCode
var result = ((keyCode >= 65 && keyCode <= 90) ||
(keyCode >= 97 && keyCode <= 122));
document.getElementById("lblMsgTextJS").style.display =
result ? "none" : "inline";
return result;
}
</script>
ASP Code
<h1>
Using JQuery
</h1>
<h3>
Restricting Numbers
</h3>
<p>
<asp:TextBox ID="tbxNumber" runat="server" MaxLength="10" />
<asp:Label ID="lblMsgNumbers" runat="server"
ForeColor="#CC0000" Text="Input Digits [0 - 9] Only" />
</p>
<h3>
Restricting Text
</h3>
<p>
<asp:TextBox ID="tbxText" runat="server" MaxLength="10" />
<asp:Label ID="lblMsgText" runat="server"
ForeColor="#CC0000" Text="Input Text [a - z] or [A - Z] Only" />
</p>
<h1>
Using Javascript
</h1>
<h3>
Restricting Numbers
</h3>
<p>
<asp:TextBox ID="tbxNumberJS" runat="server"
MaxLength="10" onkeypress="return IsDigit(event);"
ondrop="return false;" onpaste="return false;"
oncut="return false;" oncopy="return false;" />
<asp:Label ID="lblMsgNumberJS" runat="server"
ForeColor="#CC0000" Text="Input Digits [0 - 9] Only" />
</p>
<h3>
Restricting Text
</h3>
<p>
<asp:TextBox ID="tbxTextJS" runat="server"
MaxLength="10" onkeypress="return IsText(event);"
ondrop="return false;" onpaste="return false;"
oncut="return false;" oncopy="return false;" />
<asp:Label ID="lblMsgTextJS" runat="server"
ForeColor="#CC0000" Text="Input Text [a - z] or [A - Z] Only" />
</p>
Default output:
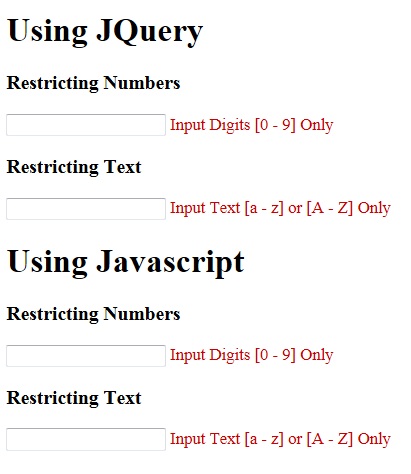
If you try to enter any other values then it would prompt a message.

Note: If you try to cut, copy, paste, or drag, values won't be accepted into textboxes.
I am a 29 year old software Web Developer from Hyderabad, India. I have been working since approximately age 25. Where as in IT Development industry since 27. I am Microsoft Certified Technology Specialist.
I have taught myself in development, beginning with Microsoft's technologies ASP.NET, Approximately 3 years ago, I was given an opportunity to work as a freelance in the tech field. Now I am working as a web developer where my roles make me purely in web based technology solutions which manage and control access to applications and patient information stored in legacy systems, client-server applications.
I too had an opportunity to train some IT professionals with technical skills in development area. Which became my passion.
I have worked on various .NET framework versions(2.0 , 3.5, 4.0) and have been learning every new technology being introduced. Currently, I am looking forward to working in R & D in .Net to create distributed, reusable applications.