A library that reliably parses AutoCad DXF files into JSON files without missing data. It is typesafe. Parse dxf at high speed. Lightweight library
DXF-JSON
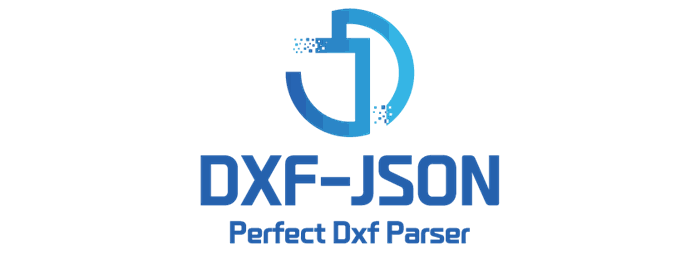







Perfect Dxf Parser
A library that reliably parses DXF files into JSON files without missing data.
- It is typesafe.
- Parse dxf at high speed.
- Lightweight library
Features
- Synchronous parsing, asynchronous parsing, and url fetch are possible.
- It was created for the purpose of parsing the AutoCad Dxf format. Dxf ref
- It is modularized and divided into Header, Classes, Tables, Blocks, Entities, and Objects sections.
- It's very simple to use.
- When I input dxf file it returns json data.
The goal of this library is to fully analyze dxf files from AutoCad and convert them to json files.
Coverage
Block:
- The
BLOCKS
section of the DXF file contains an entry for each block reference in the drawing.
Header:
- Most headers can be loaded. All that remains is to add types and add descriptions.
Entity:
arc
attdef
attribute
circle
dimension
ellipse
hatch
insert
leader
line
lwpolyline
mtext
point
polyline
section
solid
spline
text
vertex
viewport
Tables:
blockRecord
dimStyle
layer
ltype
style
vport
Objects:
dictionary
layout
plotSettings
Reference
I was able to get a lot of ideas from the dxf-parser library.
How Do I Use It?
npm
package:
npm i dxf-json
build
:
npm install
npm run build
test
:
npm install
npm run test:parser
parseSync
:
const parser = new DxfParser()
return parser.parseSync(buffer)
parseStream
:
import fs from 'fs'
const parser = new DxfParser();
const fileStream = fs.createReadStream("dxf file path", { encoding: 'utf8' });
return await parser.parseStream(fileStream);
parseUrl
:
const parser = new DxfParser();
return await parser.parseFromUrl(url, encoding, RequestInit);
Type
ParsedDxf
:
interface ParsedDxf {
header: DxfHeader;
blocks: Record<string, DxfBlock>;
entities: CommonDxfEntity[];
tables: {
BLOCK_RECORD?: DxfTable<BlockRecordTableEntry>;
DIMSTYLE?: DxfTable<DimStylesTableEntry>;
STYLE?: DxfTable<StyleTableEntry>;
LAYER?: DxfTable<LayerTableEntry>;
LTYPE?: DxfTable<LTypeTableEntry>;
VPORT?: DxfTable<VPortTableEntry>;
};
objects: {
byName: Record<string, CommonDXFObject[]>;
byTree?: DxfObject;
};
}
header
DxfHeader
:
type DxfHeaderVariable =
...
| 'DRAGVS'
| 'INTERFERECOLOR'
| 'INTERFEREOBJVS'
| 'INTERFEREVPVS'
| 'OBSLTYPE'
| 'SHADEDIF'
| 'MEASUREMENT';
export type DxfHeader = typeof DefaultDxfHeaderVariables & {
MEASUREMENT: Measurement;
} & Record<string, any>;
blocks
DxfBlock
:
interface DxfBlock {
type: number;
name: string;
name2: string;
handle: string;
ownerHandle: string;
layer: string;
position: Point3D;
paperSpace: boolean;
xrefPath: string;
entities?: CommonDxfEntity[];
}
entities
CommonDxfEntity
:
interface CommonDxfEntity {
type: string;
handle: string;
ownerBlockRecordSoftId?: string;
isInPaperSpace?: boolean;
layer: string;
lineType?: string;
materialObjectHardId?: string;
colorIndex?: ColorIndex;
lineweight?: number;
lineTypeScale?: number;
isVisible?: boolean;
proxyByte?: number;
proxyEntity?: string;
color?: ColorInstance;
colorName?: string;
transparency?: number;
plotStyleHardId?: string;
shadowMode?: ShadowMode;
xdata?: XData;
ownerdictionaryHardId?: string | number | boolean;
ownerDictionarySoftId?: string | number | boolean;
}
tables
tables: {
BLOCK_RECORD?: DxfTable<BlockRecordTableEntry>;
DIMSTYLE?: DxfTable<DimStylesTableEntry>;
STYLE?: DxfTable<StyleTableEntry>;
LAYER?: DxfTable<LayerTableEntry>;
LTYPE?: DxfTable<LTypeTableEntry>;
VPORT?: DxfTable<VPortTableEntry>;
};
DxfTable
interface DxfTable<T extends CommonDxfTableEntry> {
subclassMarker: 'AcDbSymbolTable';
name: string;
handle: string;
ownerDictionaryIds?: string[];
ownerObjectId: string;
maxNumberOfEntries: number;
entries: T[];
}
interface CommonDxfTableEntry {
name: string;
handle: string;
ownerObjectId: string;
}
BLOCK_RECORD
BlockRecordTableEntry
:
interface BlockRecordTableEntry extends CommonDxfTableEntry {
subclassMarker: 'AcDbBlockTableRecord';
name: string;
layoutObjects: string;
insertionUnits: number;
explodability: number;
scalability: number;
bmpPreview: string;
}
DIMSTYLE
type DimStyleVariable =
| 'DIMPOST'
| 'DIMAPOST'
| 'DIMBLK_OBSOLETE'
| 'DIMBLK1_OBSOLETE'
| 'DIMBLK2_OBSOLETE'
| 'DIMSCALE'
| 'DIMASZ'
| 'DIMEXO'
| 'DIMDLI'
| 'DIMEXE'
| 'DIMRND'
| 'DIMDLE'
| 'DIMTP'
| 'DIMTM'
| 'DIMTXT'
| 'DIMCEN'
| 'DIMTSZ'
| 'DIMALTF'
| 'DIMLFAC'
| 'DIMTVP'
| 'DIMTFAC'
| 'DIMGAP'
| 'DIMALTRND'
| 'DIMTOL'
| 'DIMLIM'
| 'DIMTIH'
| 'DIMTOH'
| 'DIMSE1'
| 'DIMSE2'
| 'DIMTAD'
| 'DIMZIN'
| 'DIMAZIN'
| 'DIMALT'
| 'DIMALTD'
| 'DIMTOFL'
| 'DIMSAH'
| 'DIMTIX'
| 'DIMSOXD'
| 'DIMCLRD'
| 'DIMCLRE'
| 'DIMCLRT'
| 'DIMADEC'
| 'DIMUNIT'
| 'DIMDEC'
| 'DIMTDEC'
| 'DIMALTU'
| 'DIMALTTD'
| 'DIMAUNIT'
| 'DIMFRAC'
| 'DIMLUNIT'
| 'DIMDSEP'
| 'DIMTMOVE'
| 'DIMJUST'
| 'DIMSD1'
| 'DIMSD2'
| 'DIMTOLJ'
| 'DIMTZIN'
| 'DIMALTZ'
| 'DIMALTTZ'
| 'DIMFIT'
| 'DIMUPT'
| 'DIMATFIT'
| 'DIMTXSTY'
| 'DIMLDRBLK'
| 'DIMBLK'
| 'DIMBLK1'
| 'DIMBLK2'
| 'DIMLWD'
| 'DIMLWE';
interface DimStyleVariableSchema {
name: string;
code: number;
defaultValue?: string | number;
defaultValueImperial?: string | number;
}
type DimStylesTableEntry = {
subclassMarker: 'AcDbDimStyleTableRecord';
styleName: string;
DIMPOST?: string;
DIMAPOST?: string;
DIMBLK_OBSOLETE?: string;
DIMBLK1_OBSOLETE?: string;
DIMBLK2_OBSOLETE?: string;
DIMSCALE: number;
DIMASZ: number;
DIMEXO: number;
DIMDLI: number;
DIMEXE: number;
DIMRND: number;
DIMDLE: number;
DIMTP: number;
DIMTM: number;
DIMTXT: number;
DIMCEN: number;
DIMTSZ: number;
DIMALTF: number;
DIMLFAC: number;
DIMTVP: number;
DIMTFAC: number;
DIMGAP: number;
DIMALTRND: number;
DIMTOL: number;
DIMLIM: number;
DIMTIH: number;
DIMTOH: number;
DIMSE1: 0 | 1;
DIMSE2: 0 | 1;
DIMTAD: DimensionTextVertical;
DIMZIN: DimensionZeroSuppression;
DIMAZIN: DimensionZeroSuppressionAngular;
DIMALT: 0 | 1;
DIMALTD: number;
DIMTOFL: 0 | 1;
DIMSAH: 0 | 1;
DIMTIX: 0 | 1;
DIMSOXD: 0 | 1;
DIMCLRD: number;
DIMCLRE: number;
DIMCLRT: number;
DIMADEC?: number;
DIMUNIT?: number;
DIMDEC: number;
DIMTDEC: number;
DIMALTU: number;
DIMALTTD: number;
DIMAUNIT: number;
DIMFRAC: number;
DIMLUNIT: number;
DIMDSEP: string;
DIMTMOVE: undefined;
DIMJUST: DimensionTextHorizontal;
DIMSD1: 0 | 1;
DIMSD2: 0 | 1;
DIMTOLJ: DimensionTextVertical;
DIMTZIN: DimensionZeroSuppression;
DIMALTZ: DimensionZeroSuppression;
DIMALTTZ: DimensionZeroSuppression;
DIMFIT?: number;
DIMUPT: number;
DIMATFIT: number;
DIMTXSTY?: string;
DIMLDRBLK?: string;
DIMBLK?: string;
DIMBLK1?: string;
DIMBLK2?: string;
DIMLWD: number;
DIMLWE: number;
} & CommonDxfTableEntry;
type StyleResolver = <Name extends DimStyleVariable>(
variableName: Name,
) => DimStylesTableEntry[Name];
STYLE
interface StyleTableEntry extends CommonDxfTableEntry {
subclassMarker: 'AcDbTextStyleTableRecord';
name: string;
standardFlag: number;
fixedTextHeight: number;
widthFactor: number;
obliqueAngle: number;
textGenerationFlag: number;
lastHeight: number;
font: string;
bigFont: string;
extendedFont?: string;
}
LAYER
interface LayerTableEntry extends CommonDxfTableEntry {
subclassMarker: 'AcDbLayerTableRecord';
name: string;
standardFlag: number;
colorIndex: ColorIndex;
lineType: string;
isPlotting: boolean;
lineweight: number;
plotStyleNameObjectId?: string;
materialObjectId?: string;
}
LTYPE
interface LTypeTableEntry extends CommonDxfTableEntry {
subclassMarker: 'AcDbLinetypeTableRecord';
name: string;
standardFlag: number;
description: string;
numberOfLineTypes: number;
totalPatternLength: number;
pattern?: LineTypeElement[];
}
interface LineTypeElement {
elementLength: number;
elementTypeFlag: number;
shapeNumber?: number;
styleObjectId?: string;
scale?: number;
rotation?: number;
offsetX?: number;
offsetY?: number;
text?: string;
}
VPORT
interface VPortTableEntry extends CommonDxfTableEntry {
subclassMarker: 'AcDbViewportTableRecord';
name: string;
standardFlag: number;
lowerLeftCorner: Point2D;
upperRightCorner: Point2D;
center: Point2D;
snapBasePoint: Point2D;
snapSpacing: Point2D;
gridSpacing: Point2D;
viewDirectionFromTarget: Point3D;
viewTarget: Point3D;
lensLength: number;
frontClippingPlane: number;
backClippingPlane: number;
viewHeight: number;
snapRotationAngle: number;
viewTwistAngle: number;
circleSides: number;
frozenLayers: string[];
styleSheet: string;
renderMode: RenderMode;
viewMode: number;
ucsIconSetting: number;
ucsOrigin: Point3D;
ucsXAxis: Point3D;
ucsYAxis: Point3D;
orthographicType: OrthographicType;
elevation: number;
shadePlotSetting: number;
majorGridLines: number;
backgroundObjectId?: string;
shadePlotObjectId?: string;
visualStyleObjectId?: string;
isDefaultLightingOn: boolean;
defaultLightingType: DefaultLightingType;
brightness: number;
contrast: number;
ambientColor?: number;
}
objects
objects: {
byName: Record<string, CommonDXFObject[]>;
byTree?: DxfObject;
};
byName
CommonDXFObject
:
interface CommonDXFObject {
ownerObjectId: string;
ownerDictionaryIdHard: string;
ownerDictionaryIdSoft: string;
handle: string;
}
DxfObject
interface DxfObject {
name: string;
handle: string;
ownerDictionaryIdSoft: string;
ownerDictionaryIdHard?: string;
ownerObjectId?: string;
}
Please refer to the dxf
reference for undefined elements and leave them as issues!
History
- 29th January, 2024: Initial version
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.