Integration testing is crucial in software development, ensuring components work in harmony. However, when using xUnit for these tests, developers often miss out on detailed logs, making debugging a challenge. This article introduces a solution that seamlessly reintroduces comprehensive logging, offering valuable insights into test behaviors and simplifying the troubleshooting process.
Introduction
Integration testing ensures that various components of an application work together seamlessly. For developers using xUnit for integration testing, a limitation arises where logs disappear during the tests. Without these logs, it's challenging to understand the behavior of a test. This article introduces the ConnectingApps.Xunit.TestLogger NuGet package, designed to bring back logging capabilities during xUnit integration tests, offering insights into the test's behavior.
Background
In the .NET world, the WebApplicationFactory
class is a fundamental tool for setting up integration tests for web applications. This class, part of the Microsoft.AspNetCore.Mvc.Testing package, allows developers to establish and configure a web application instance tailored for testing. A comprehensive guide on using the WebApplicationFactory
for integration testing can be found in Microsoft's official documentation.
Using the Code
To reintroduce logging capabilities with the ConnectingApps.Xunit.TestLogger NuGet package, a slight adjustment in the setup of your tests is needed. It supports .NET 6, .NET 7 and .NET 8 .
Previously, you'd set up the WebApplicationFactory
as shown:
public NoLoggingTest()
{
_factory = new WebApplicationFactory<Program>();
}
With the ConnectingApps.Xunit.TestLogger package, use this approach:
public ImprovedLoggingTest(ITestOutputHelper output)
{
_factory = new TestLoggerWebApplicationFactory<Program>(output);
}
To understand this better, let's look at a detailed controller method:
[HttpGet(Name = "GetWeatherForecast")]
public IEnumerable<weatherforecast> Get()
{
_logger.LogInformation("This should be logged during testing");
return Enumerable.Range(1, 5).Select(index => new WeatherForecast
{
Date = DateTime.Now.AddDays(index),
TemperatureC = Random.Shared.Next(-20, 55),
Summary = Summaries[Random.Shared.Next(Summaries.Length)]
})
.ToArray();
}
Here's a corresponding test for the method:
using ConnectingApps.Xunit.TestLogger;
using System.Net;
using Xunit.Abstractions;
public class ImprovedLoggingTest : IDisposable
{
private readonly TestLoggerWebApplicationFactory<Program> _factory;
private readonly HttpClient _client;
public ImprovedLoggingTest(ITestOutputHelper output)
{
_factory = new TestLoggerWebApplicationFactory<Program>(output);
_client = _factory.CreateClient();
}
[Fact]
public async Task ReadInTestOutputIfSomethingIsLogged()
{
var response = await _client.GetAsync("/WeatherForecast");
Assert.Equal(HttpStatusCode.OK, response.StatusCode);
}
public void Dispose()
{
_factory.Dispose();
_client.Dispose();
}
}
For a more comprehensive understanding and additional examples, the entire source code and related documentation can be found on GitHub.
Here's a visual representation of how the logging appears when using the ConnectingApps.Xunit.TestLogger:
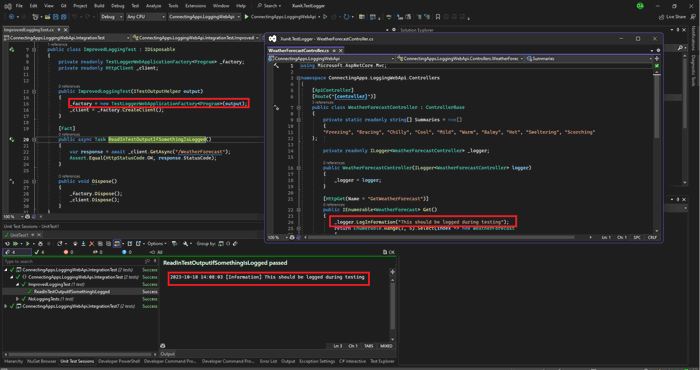
Points of Interest
The introduction of the ConnectingApps.Xunit.TestLogger package offers a straightforward solution to a long-standing problem. Its seamless integration with existing xUnit tests and the ability to provide comprehensive logging enhances the debugging experience for developers.
History
I am a self-employed software engineer working on .NET Core. I love TDD.