Introduction
Looking back at the ASP.NET AJAX and the accompanying ASP.NET AJAX Control Toolkit, I decided to use the Rating control in one of my ASP.NET Web Forms project only to experience how misleading online bad documentation can prove to be.
This article demonstrates how to utilize the Rating control by properly accessing it both from code-behind and from JavaScript (using the Microsoft Ajax Library of the ASP.NET AJAX framework).
Background
A rating control allows the users to express their opinion about something by submitting a numerical value within a given range. Most often, values are presented as stars.
The Rating control is an Ajax-enabled server-side component that does exactly that. It is part of the AJAX Control Toolkit that needs to be downloaded and installed separately. Once installed, you can drag and drop the Rating control from the Visual Studio Toolbox on to your web page and have an assembly reference added to the project automatically, and the AJAX Control Toolkit assembly registered on the page, too. The installation procedure is nicely described in this step by step installation guide. At the time of this writing, the toolkit version is 19.1.
This is how the control may look like on a web page:

Getting Started
To get started, open Visual Studio and create a new ASP.NET Web Forms application project. The ASP.NET AJAX framework is bundled in your project, by default. If you've followed the installation procedure described above, you should be able to see the AJAX Control Toolkit controls in the Toolbox, too.
Open Default.aspx for editing and drag and drop the Rating control on to the page. The AjaxControlToolkit
assembly is automatically registered with the page:
<%@ Register Assembly="AjaxControlToolkit"
Namespace="AjaxControlToolkit" TagPrefix="ajaxToolkit" %>
and the following markup is added:
<ajaxToolkit:Rating ID="Rating1" runat="server"></ajaxToolkit:Rating>
Modify the control markup as follows:
<ajaxToolkit:Rating
ID="Rating1"
BehaviorID="RatingBehavior1"
runat="server"
CurrentRating="0"
StarCssClass="ratingStar"
WaitingStarCssClass="savedRatingStar"
FilledStarCssClass="filledRatingStar"
EmptyStarCssClass="emptyRatingStar"
OnChanged="Rating1_Changed">
</ajaxToolkit:Rating>
The Rating control depends on certain CSS classes for its rendering. This allows you to define how the stars will look like when visible, empty, filled or in waiting mode. Here are the CSS rules for this demo:
.ratingStar {
font-size: 0pt;
width: 64px;
height: 59px;
margin: 5px;
padding: 5px;
cursor: pointer;
display: block;
background-repeat: no-repeat;
}
.filledRatingStar {
background-image: url(../Images/FilledStar.png);
}
.emptyRatingStar {
background-image: url(../Images/EmptyStar.png);
}
.savedRatingStar {
background-image: url(../Images/SavedStar.png);
}
Make sure you place the above rules in the Site.css file. The star images are included in this article for your convenience. Copy them in the Images folder of your project.
Finally, to complete the demo UI, wrap the control markup so it looks like below:
<h2>What do you think?</h2>
<p>
How much do you love ASP.NET AJAX?
</p>
<p>
<ajaxToolkit:Rating
ID="Rating1"
BehaviorID="RatingBehavior1"
runat="server"
CurrentRating="0"
StarCssClass="ratingStar"
WaitingStarCssClass="savedRatingStar"
FilledStarCssClass="filledRatingStar"
EmptyStarCssClass="emptyRatingStar"
OnChanged="Rating1_Changed">
</ajaxToolkit:Rating>
</p>
<p style="clear:both">
<asp:Label ID="LabelRating" runat="server">Please rate. Your vote matters!</asp:Label>
</p>
The below screenshot shows the resulting output:
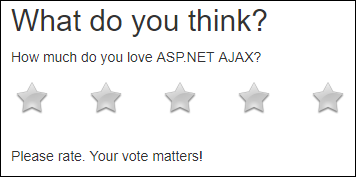
Using the Code
As already said, Rating is an Ajax-enabled server-side control. Every time the user clicks on a star thus changing its value, an asynchronous postback occurs and the event handler of the OnChanged
event is executed. During the execution of the event handler's code, the control is in wait mode and the stars are shown as defined by the WaitingStarCssClass
rule.
At this point, it is very important to understand that an asynchronous Ajax postback is always performed regardless of the value of the AutoPostBack
property and without the need of being wrapped in a UpdatePanel
control! (Now, go delete half your bookmarks about the Rating control).
Here's the Rating1_Changed
event handler as defined in the code-behind of the web page:
protected void Rating1_Changed(object sender, AjaxControlToolkit.RatingEventArgs e)
{
try
{
SetRatingToCookie(e.Value);
e.CallbackResult = "Got your " + e.Value + "! Thank you.";
}
catch (Exception ex)
{
e.CallbackResult = "The following error occurred: " + ex.Message + ". Please try again";
}
}
e.Value
holds the newly selected rating value as a string
(even though it is actually a numerical value). For demonstration purposes, the value is stored in a cookie, which, if present, will be used as the current rating value the next time the page is loaded. Here's the SetRatingToCookie
method:
protected void SetRatingToCookie(string value)
{
var cookie = new HttpCookie(CookieKey);
if (!string.IsNullOrEmpty(value) && !value.Equals("0"))
{
cookie.Values.Add(RatingKey, value);
cookie.Expires = DateTime.Now.AddYears(1);
Response.Cookies.Add(cookie);
}
}
Since this is an asynchronous postback, any results (or errors) that should be communicated back to the client must be passed as the string
value of e.CallbackResult
. On the client side, a callback function can be added to the Rating control through JavaScript to show the results using the LabelRating
control found in the page:
<script type="text/javascript">
function pageLoad() {
$find('RatingBehavior1').add_endClientCallback(function (sender, e) {
var labelRating = $get('<%= LabelRating.ClientID %>');
labelRating.innerHTML = e.get_CallbackResult();
});
}
</script>
The pageLoad
function is automatically called by the Microsoft Ajax Library when the page is fully loaded. It is equivalent to jQuery's $( document ).ready()
event.
Here's how the page looks like after the user gives a 5 star rating:

As mentioned earlier, you can check for the presence of the cookie and set the current value (CurrentRating
property) of the Rating control when the page get loaded:
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
var rating = GetRatingFromCookie();
if (rating > 0)
{
Rating1.CurrentRating = rating;
LabelRating.Text = string.Format
("We've already got your rate of {0} but feel free to change your mind.",
rating.ToString());
}
}
}
protected int GetRatingFromCookie()
{
var cookie = Request.Cookies[CookieKey];
if (cookie != null)
{
int rating = 0;
Int32.TryParse(cookie[RatingKey], out rating);
return rating;
}
else
{
return 0;
}
}
The page can then inform the user of any existing rating:

Rating is an Ajax-enabled server control that works with asynchronous Ajax postbacks right out-of-the-box. It can be accessed both from code-behind and JavaScript on the client side. It is easy to configure and work with as long as you spend some time playing with it and understanding its purpose and design. More than a handful of erroneous blog posts, misleading forum threads and bad documentation do no justice to the control.
History
- 15th August, 2019: Initial version
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.