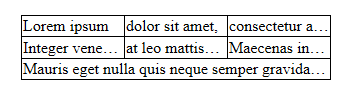
Introduction
This is a quick and dirty usage of tabular data and marquee
. You're not supposed to use marquee
tag because it is marked as deprecated but right now, all the major browsers are supporting it. Also, this code doesn't give a uniform experience across all browsers because each browser implements marquee
differently. The alternative is to use other JavaScript/jQuery libraries that mimic a marquee. However, it you're not interested in delving into yet another library, then this is going to be a fairly good, copy-paste quick, solution for you.
Let's say that you need a table
with columns that have fixed length. The data that is going into the table
can vary in length. If the data fits into the cell, it will be displayed as it is. If the data is longer than what the cell can fit in length, then the data will be truncated and a suffix of ellipsis will be added. If the user hovers with the mouse over the cell, the data will marquee inside the cell. when the user hovers out, the marquee will stop.
We start with a bare table
and a few Lorem ipsum sentences.
<table>
<tr>
<td>Lorem ipsum</td>
<td>dolor sit amet,</td>
<td>consectetur adipiscing elit.</td>
</tr>
<tr>
<td>Integer venenatis mauris</td>
<td>at leo mattis ullamcorper.</td>
<td>Maecenas in dui rutrum massa consequat convallis non in nibh.</td>
</tr>
<tr>
<td colspan="3">Mauris eget nulla quis neque semper gravida et non tortor.</td>
</tr>
</table>
The CSS code sets the columns at a fixed-length of 100px. The min-width
and max-width
are important to hammer the width in. The CSS overflow: hidden
tells the browser to hide any text that overflows out of the cell. Without this, the text will flow over to the adjacent cell. The CSS white-space: nowrap
tells the browser not to cramp the content into the cell. Without this, the cell will extend in height to accommodate the whole text. The CSS text-overflow: ellipsis
tells the browser to add an ellipsis suffix if the text does overflow. The ellipsis gives an indication to the user which cells are overflowing.
table
{
border-collapse: collapse;
}
td
{
border: 1px solid black;
width: 100px;
max-width: 100px;
min-width: 100px;
overflow: hidden;
white-space: nowrap;
text-overflow: ellipsis;
}
First, we need to detect which cells are overflowing. For each cell, we compare if its scrollWidth
is bigger or equal to jQuery $.outerWidth()
, which will properly calculate the outer width for various browsers and their quirks.
var overflowCells = $("td").filter(function (index, element) {
return (element.scrollWidth >= $(element).outerWidth());
});
Now, we bind mouse events. When the mouse enters the cell, the inner content of the cell is wrapped with marquee
. When the mouse leaves the cell, the marquee
is removed and the original HTML is restored.
overflowCells.mouseenter(function () {
$(this).wrapInner("<marquee>");
});
overflowCells.mouseleave(function () {
$(this).html($(this).find("marquee").html());
});
Finally, let's wrap it up in a little jQuery extension and expose some convenient marquee
properties.
$.fn.extend({
marquee: function (options) {
var marqueeProperties = $.extend({
behavior: "scroll",
direction: "left",
loop: -1
}, options);
return this.filter(function (index, element) {
return (element.scrollWidth >= $(element).outerWidth());
}).mouseenter(function () {
$(this).wrapInner($("<marquee>").prop(marqueeProperties));
}).mouseleave(function () {
$(this).html($(this).find("marquee").html());
}).end();
}
});