Introduction
This is a continuation of this article (Part 1). iOS uses several terms which might be unfamiliar or have different meanings than what we are used to as a .NET developer. Like below. In this article, we will try to explore each of this in detail to strengthen our foundation in iOS concepts.
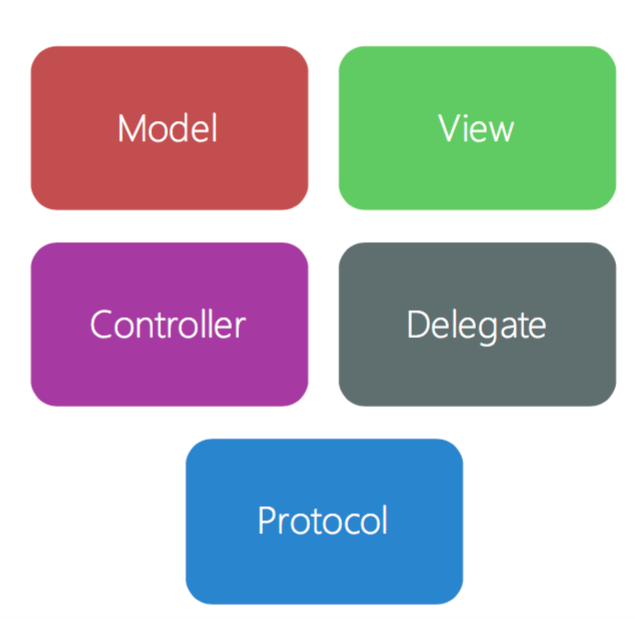
Background
As this is series of articles is coming through one by one, a good jump start would be to go and see the first article of our series: Let's build iOS app in Xamarin.iOS Part I.
What is MVC?
- Model-View-Controller (MVC) is an established architectural design pattern to logically separate the UI, data and behavior of an application. Not only in .NET or iOS, it is widely used in all forms of application development languages.
- This is the base design pattern for all iOS applications and its usage is enforced by the iOS API design.
Model
Model particularly holds all the data entities and basic data related logics. This may include Data entities, validation logic, processing logic, calculation functions but according to the MVC pattern, all the logic we write over here has to limit itself to only our base data. Again iOS doesn't provide any out of the box support to it - this Model layer is all developer created.
View
View layer is quite evident by its name itself. View simply represents every visual element user sees in the screen such as button, text, etc. All of them derive from a base class called UIView.
Views can be either defined in code or we can declaratively make it using a StoryBoard or Xib file.
Controller
The Controller is the moderator between the model and the view, in iOS these are classes that derive from UIViewController
. Take a good look at the following image. It will greatly explain what a controllers' role is. Remember there are cases where Model does merge into Controller, for simple application there might not even be models only controller. When your application does get complex, you might then realize the importance of Model and Controller for maintenance purposes.

How iOS App Starts
public class AppDelegate : UIApplicationDelegate {
public override UIWindow Window { get; set; }
public override bool FinishedLaunching(...) {
Window = new UIWindow(UIScreen.MainScreen.Bounds);
Window.RootViewController = new MyViewController();
Window.MakeKeyAndVisible();
return true;
}
As we discussed in Part I of our article, we have seen that AppDelegate.cs is the class which holds all the scenarios of our application start/restart/resume OS notifications. Particularly FinishedLaunching
method is the one which does set the Applications 1st screen View and Controller.
So we must remember that our app must identify a single view controller to be the starting controller. So let's imagine the name of the controller is MyViewController
. So in our case, our view is defined in the below line:
Window = new UIWindow(UIScreen.MainScreen.Bounds);
and for that particular Window, the controller is being set as below:
Window.RootViewController = new MyViewController();
What is a Delegate in iOS?
Coming from .NET background, we have to remember that delegation in iOS is different from delegation .NET. Although the idea is the same, but in iOS it is being used in a little broader sense.
In .NET, delegate is a method pointer whereas in iOS, it is more like an interface which provides methods.
- iOS uses a delegation pattern to provide behavior for classes without derivation.
- Most often used for notifications from iOS to parts of your app.
- Delegate methods often use the Should, Will, Did pattern to support customization + notification.
What is a Protocol in iOS?
- The operations (messages) a delegate supports are defined by a protocol
- Like interfaces in .NET – iOS objects can implement (conform) to multiple protocols to provide a standard contract
- Unlike interfaces, protocols support optional and
static
methods; to enable this, Xamarin.iOS
models protocols as abstract
classes with abstract
and virtual
methods
Points of Interest
So now, we have the idea of all the popular terminologies in iOS. In the next part of the article, we will straight away start with our application building.
History
.NET developer with close proximity to Mobile Technology(Xamarin/Windows Phone). Also passionate about ASP.NET MVC/WebApi development