Read Write Windows Registry C# VB.NET Reusable Code






4.82/5 (14 votes)
Read Write Windows Registry C# VB.NET Reusable Code
Introduction
I will show you how to create a base class (C#) which main purpose will be to write and read data to/from Window registry. Next it can be derived from more custom classes where you can put the logic for your application.
The main classes that you will need are placed in the mscorlib.dll - Microsoft.Win32 namespace. The DLL is referenced by default from all of yours .NET projects so you only need to add using to the namespace.
Windows Registry Read Write C# Code
Below you can see the default structure of the registry in Windows. The tree of folders(subKeys) contains keys with Name, Type and Value(Data). To be able to operate with them, you first need to open them. Once you do that you can perform read/write operations.Below you can see the default structure of the registry in Windows. The tree of folders(subKeys) contains keys with Name, Type and Value(Data). To be able to operate with them, you first need to open them. Once you do that you can perform read/write operations.
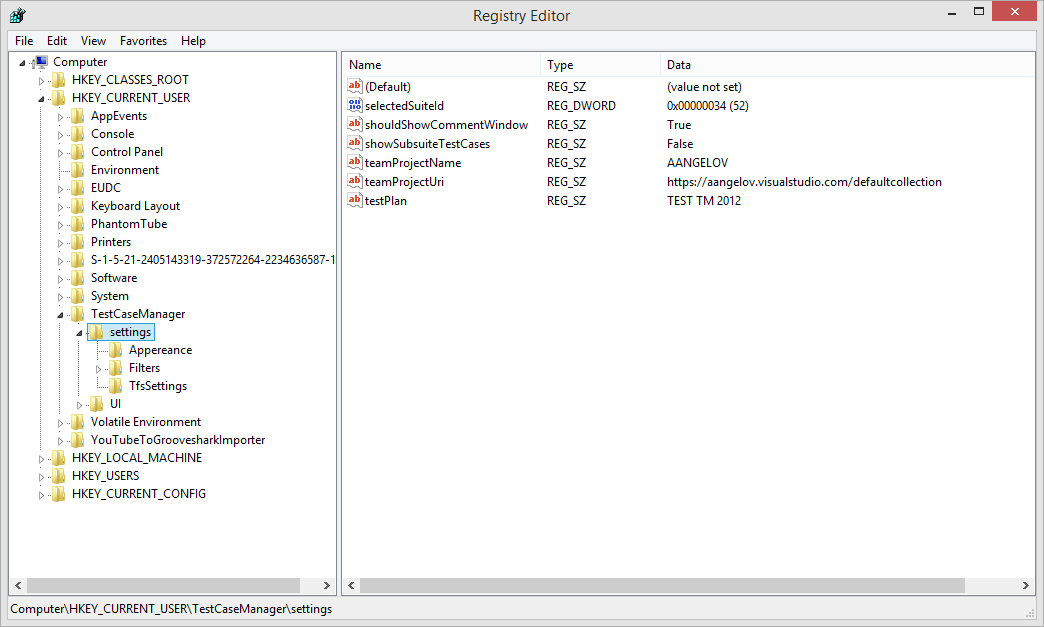
RegistryKey key;
key = Registry.CurrentUser.CreateSubKey("Names");
key.SetValue("Name", "Isabella");
key.Close();
The code above creates the "folder"(subKey) Names under HKEY_CURRENT_USER and creates a key with the name - "Name", the value "Isabella" and Type - string.
For read operations, you can use OpenSubKey and GetValue methods.
So far so good. However, you don't want to write these 3-4 lines of code for every read/write operation. You can use the following code to create a reusable service class for these actions.
using System;
using System.Collections.Generic;
using System.Linq;
using Microsoft.Win32;
public abstract class BaseRegistryService
{
private static readonly log4net.ILog log = log4net.LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
protected string MainRegistrySubKey;
protected void Write(string subKeys, Object value)
{
string[] subKeyNames = subKeys.Split('/');
List<RegistryKey> registryKeys = new List<RegistryKey>();
for (int i = 0; i < subKeyNames.Length; i++)
{
RegistryKey currentRegistryKey = default(RegistryKey);
if (i == 0)
{
currentRegistryKey = Registry.CurrentUser.CreateSubKey(subKeyNames[i]);
}
else if (i < subKeyNames.Length - 1)
{
currentRegistryKey = registryKeys[i - 1].CreateSubKey(subKeyNames[i]);
}
else
{
registryKeys.Last().SetValue(subKeyNames.Last(), value);
}
registryKeys.Add(currentRegistryKey);
}
this.CloseAllRegistryKeys(registryKeys);
}
protected Object Read(string subKeys)
{
Object result = default(Object);
try
{
string[] subKeyNames = subKeys.Split('/');
List<RegistryKey> registryKeys = new List<RegistryKey>();
for (int i = 0; i < subKeyNames.Length - 1; i++)
{
RegistryKey currentRegistryKey = default(RegistryKey);
if (i == 0)
{
currentRegistryKey = Registry.CurrentUser.OpenSubKey(subKeyNames[i]);
}
else
{
currentRegistryKey = registryKeys[i - 1].OpenSubKey(subKeyNames[i]);
}
registryKeys.Add(currentRegistryKey);
if (registryKeys.Last() != null && subKeyNames.Last() != null)
{
result = registryKeys.Last().GetValue(subKeyNames.Last());
}
}
}
catch (Exception ex)
{
log.Error(ex);
}
return result;
}
protected int ReadInt(string subKeys)
{
int result = (int)this.Read(subKeys);
return result;
}
protected double? ReadDouble(string subKeys)
{
object obj = this.Read(subKeys);
double? result = null;
if(obj != null)
{
result = double.Parse(obj.ToString());
}
return result;
}
protected bool ReadBool(string subKeys)
{
bool result = default(bool);
string resultStr = (string)this.Read(subKeys);
if (!string.IsNullOrEmpty(resultStr))
{
result = bool.Parse(resultStr);
}
return result;
}
protected string ReadStr(string subKeys)
{
string result = string.Empty;
string resultStr = (string)this.Read(subKeys);
if (!string.IsNullOrEmpty(resultStr))
{
result = resultStr;
}
return result;
}
protected string GenerateMergedKey(params string[] keys)
{
string result = this.MainRegistrySubKey;
foreach (var currentKey in keys)
{
result = string.Join("/", result, currentKey);
}
return result;
}
private void CloseAllRegistryKeys(List<RegistryKey> registryKeys)
{
for (int i = 0; i < registryKeys.Count - 1; i++)
{
registryKeys[i].Close();
}
}
}
The “MainRegistrySubKey” is the default subKey for you application- Test Case Manager.

Next if you want to add a new key with a particular value under TestCaseManager\Settings\Appearance- you need to create the three subKeys and then create the key and set the value. With the base service, it will be an easy task. You need to pass to the Write method the following parameters "\Settings\Appearance\color" and the value for the color - for example red.
The code is going to create the TestCaseManager, Settings, Appearance subKeys and color key under Appearance. After that, it is going to assign red to the color key. Most probably you are going to create private variables that are going to hold the different subKey names.
public class UIRegistryManager : BaseRegistryManager
{
private static UIRegistryManager instance;
private readonly string themeRegistrySubKeyName = "theme";
private readonly string shouldOpenDropDownOnHoverRegistrySubKeyName = "shouldOpenDropDrownOnHover";
private readonly string titlePromptDialogRegistrySubKeyName = "titlePromptDialog";
public UIRegistryManager(string mainRegistrySubKey)
{
this.MainRegistrySubKey = mainRegistrySubKey;
}
public static UIRegistryManager Instance
{
get
{
if (instance == null)
{
string mainRegistrySubKey = ConfigurationManager.AppSettings["mainUIRegistrySubKey"];
instance = new UIRegistryManager(mainRegistrySubKey);
}
return instance;
}
}
public void WriteCurrentTheme(string theme)
{
this.Write(this.GenerateMergedKey(this.themeRegistrySubKeyName), theme);
}
public void WriteTitleTitlePromtDialog(string title)
{
this.Write(this.GenerateMergedKey(this.titlePromptDialogRegistrySubKeyName, this.titleTitlePromptDialogIsCanceledRegistrySubKeyName), title);
}
public bool ReadIsCheckboxDialogSubmitted()
{
return this.ReadBool(this.GenerateMergedKey(this.checkboxPromptDialogIsSubmittedRegistrySubKeyName));
}
public string ReadTheme()
{
return this.ReadStr(this.GenerateMergedKey(this.themeRegistrySubKeyName));
}
}
You can use the GenerateMergedKey method to generate the subKeys chain parameter- "TestCaseManager\Settings\Appearance". The base class contains methods(ReadBool, ReadStr, ReadInt) which return the data parsed to the most common data types - bool, string, int. If you need, you can add your own easily.
So Far in the C# Series
1. Implement Copy Paste C# Code
2. MSBuild TCP IP Logger C# Code
3. Windows Registry Read Write C# Code
4. Change .config File at Runtime C# Code
5. Generic Properties Validator C# Code
6. Reduced AutoMapper- Auto-Map Objects 180% Faster
7. 7 New Cool Features in C# 6.0
8. Types Of Code Coverage- Examples In C#
9. MSTest Rerun Failed Tests Through MSTest.exe Wrapper Application
10. Hints For Arranging Usings in Visual Studio Efficiently
11. 19 Must-Know Visual Studio Keyboard Shortcuts – Part 1
12. 19 Must-Know Visual Studio Keyboard Shortcuts – Part 2
13. Specify Assembly References Based On Build Configuration in Visual Studio
14. Top 15 Underutilized Features of .NET
15. Top 15 Underutilized Features of .NET Part 2
16. Neat Tricks for Effortlessly Format Currency in C#
17. Assert DateTime the Right Way MSTest NUnit C# Code
18. Which Works Faster- Null Coalescing Operator or GetValueOrDefault or Conditional Operator
19. Specification-based Test Design Techniques for Enhancing Unit Tests
20. Get Property Names Using Lambda Expressions in C#
21. Top 9 Windows Event Log Tips Using C#
If you enjoy my publications, feel free to SUBSCRIBE
Also, hit these share buttons. Thank you!
Source Code
The post- Read Write Windows Registry C# VB.NET Reusable Code appeared first on Automate The Planet.
All images are purchased from DepositPhotos.com and cannot be downloaded and used for free. License Agreement