Introduction
I have searched since many days but I did not find any proper guideline which would have been helpful for me when I was a beginner in SASS. I think SASS is the controlling language of CSS. If you are a CSS designer, then you have to learn SASS, otherwise you cannot manage CSS in the proper way. This will be unmanageable after a certain time. That’s why I have written this article for those want to manage CSS.
Background
At first, you have to download Visual Studio plugin for SASS. Please download the SASS extension from here and here. After completing installation, you will restart Visual Studio and will see the MINDSCAPE menu in Visual Studio toolbar like below:
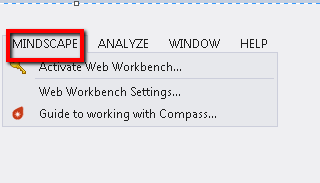
CSS is very difficult to maintain, more complex and larger. This is where SASS can help you to manage CSS in an easy way. SASS has some features like variables, nesting, mixins, and inheritance. Here, I will describe step by step. Before use of SASS, just create an SCSS file like below. Between SASS and SCSS, there is no difference without bracket. That’s why I use SCSS file for clarifying the coding.

Now rename Scss1.scss to style.scss and delete the default content of that file. Write sample CSS into this file. Here's a sample test example:
.test {
color: #f00;
}
When you will save style.scss file, then this file will be compiled and will generate CSS file like that below. This is your target file. You can now add this file into your pages. Here is the online compiler where you can test SASS code.

Variables
Variables store information and reuse for colors, font stacks, or any CSS. SASS uses the $
symbol to make a variable. Here's an example:
$black: #000;
h1{
background-color:$black;
}
Output: You can see the output in style.css.
h1 {
background-color: black;
}
Some Useful Variables
$audio-canvas-video: false;
$font-weight-bold: 700;
$font-weight-semibold: 600;
$font-weight-medium: 400;
$font-weight-regular: 400;
$font-weight-light: 300;
$font-weight-thin: 300;
$font-name: 'Segoe UI';
$font-path: '../fonts/segoeui';
$font-family-segoe-ui: $font-name, Arial, sans-serif !default;
$font-style-italic: italic;
$font-smoothing: always;
$line-height-base: 1.428571429;
$font-family-base: $font-family-segoe-ui !default;
$font-size-base: 11px;
$font-weight-base: $font-weight-regular;
$black: #000 !default;
$white: #fff !default;
$red: #f00 !default;
$light-color :#FFF !default;
$dark-color :#000 !default;
$accent-color :#f00 !default;
$gray-base: $gray !default;
$gray-dark: #5f5f5f !default;
$gray-light: #373a41 !default;
$black-base: $black !default;
$black-dark: darken($black, 80%);
$black-light: lighten($black, 80%);
$white-base: $white !default;
$white-dark: darken($white, 80%);
$white-light: lighten($white, 80%);
$red-base: $red !default;
$red-dark: darken($red, 80%);
$red-light: lighten($red, 80%);
$link-font-color: #444444 !default;
$link-color: #d04526 !default;
$link-hover-color: #c03d20 !default;
$link-background-color: $gray-light !default;
$nav-font-color: #444444 !default;
$nav-link-color: #d04526 !default;
$nav-link-hover-color: #c03d20 !default;
$nav-link-background-color: $gray-light !default;
$section-header-font-color: #444444 !default;
$section-header-link-color: #d04526 !default;
$section-header-link-hover-color: #c03d20 !default;
$body-font-color: #555555 !default;
$body-link-color: #d04526 !default;
$body-link-hover-color: #c03d20 !default;
$footer-font-color: #555555 !default;
$footer-link-color: #d04526 !default;
$footer-link-hover-color: #c03d20 !default;
$header-bg: $gray-dark !default;
$nav-bg: #adadad !default;
$body-bg: #efeff0 !default;
$footer-bg: $gray-dark !default;
$page-offset: 5px;
$screen-small: 768px;
$screen-Medium: 992px;
$screen-large: 1200px;
$element-height:22px;
Comments
SASS supports multiline CSS comments with /* */
, and single-line comments with //
. The multiline comments are preserved and single line comments are removed. For example:
.test{
color:$black;
}
Output: Comments have been removed.
.test{
color:black;
}
body { color: black; }
Output: Comments have not been removed.
body { color: black; }
Will .CSS File Generate or Not
I have placed all variables into _variables.scss file. Here, I have used underscore file name, this underscore file tells the SASS compiler which will be compile not generate CSS file like style.scss. This file is used only for import. I will tell you about import into this article letter. Here is the pictorial representation of _variables.scss. I have also downloaded twitter bootstrap and renamed file name bootstrap.css to _bootstrap.scss. I will use the twitter bootstrap class under the SASS. That’s why I have renamed the bootstrap file. You will get the _bootstrap.scss file when you will download my sample project.

Nesting
When you write HTML, you will see that HTML provides hierarchy and clear code but CSS does not do this. SASS will provide clear code and hierarchy like HTML. Here’s an example of some typical styles for a site's navigation:
footer {
.navbar-inverse {
background: $color_chicago_approx;
}
.navbar-nav {
float: left;
margin: 0;
> li > a {
padding-top: 15px;
padding-bottom: 15px;
}
}
.navbar-nav>li {
float: left;
}
}
Output of Nesting
footer .navbar-inverse {
background: #5f5f5f;
}
footer .navbar-nav {
float: left;
margin: 0;
}
footer .navbar-nav > li > a {
padding-top: 15px;
padding-bottom: 15px;
}
footer .navbar-nav > li {
float: left;
}
Use & For Link Up Parents Elements
Here is the example of &
in nesting below, where you use character &
, parents is placed there, here li
is placed into before :hover
.
.navbar-nav>li {
float: left;
&:hover{
color:red;
}
}
Output
.navbar-nav > li {
float: left;
}
.navbar-nav > li:hover {
color: red;
}
Partials
You can create partial something like _partial.scss. The underscore lets SASS know that the file is only a partial file and that it should not be generated into a CSS file. SASS partials are used with the @import
directive. I have used the two partial files into style.scss. Here is the picture below:

Imports
When you use @import
in CSS, the CSS creates another HTTP request. SASS builds on top of the current CSS @import
but instead of requiring an HTTP request, I have two files _bootstrap.scss and _variables.scss and imported into the previous example. Here is the example of @import
.
@import "variables";
@import "bootstrap";
Compass
Please see the details of compass here.
Mixins
Mixins is very important in SASS. A mixins is used for reuse. Here's an example for border-radius
.
@mixin make-property($property, $value) {
@each $prefix in -webkit-, -moz-, -ms-, -o-, '' {
#{$prefix}#{$property}: $value;
}
}
@mixin border-radius($radius) {
@include make-property(border-radius,$radius);
}
.box { @include border-radius(10px); }
Output
.box {
-webkit-border-radius: 10px;
-moz-border-radius: 10px;
-ms-border-radius: 10px;
-o-border-radius: 10px;
border-radius: 10px;
}
Some Useful Mixins
1. Font-Face:---------------------------------------------------------------------
Mixins
@mixin declare-font-face($font-family, $font-filename,
$font-weight : normal, $font-style :normal, $font-stretch : normal) {
@font-face {
font-family: '#{$font-family}';
url('../fonts/segoeui.woff') format('woff');
src: url('#{$font-filename}.woff') format('woff'),
url('#{$font-filename}.ttf') format('truetype');
font-weight: $font-weight;
font-style: $font-style;
font-stretch: $font-stretch;
}
}
Uses
$font-name: 'Segoe UI';
$font-path: '../fonts/segoeui';
@include declare-font-face($font-name,$font-path);
Output
@font-face {
font-family: "Segoe UI";
src: url("../fonts/segoeui.woff") format("woff"),
url("../fonts/segoeui.ttf") format("truetype");
font-weight: normal;
font-style: normal;
font-stretch: normal;
}
2. Responsive:---------------------------------------------------------------------
Mixins
@mixin breakpoint($point) {
@if $point == large {
@media only screen and (max-width: $screen-large) {
@content;
}
}
@else if $point == medium {
@media only screen and (max-width: $screen-Medium) {
@content;
}
}
@else if $point == small {
@media only screen and (max-width: $screen-small) {
@content;
}
}
}
Uses
$screen-small: 768px;
$screen-Medium: 992px;
$screen-large: 1200px;
.sidebar {
@include breakpoint(medium){
width: 60%;
}
}
Output
@media only screen and (max-width: 992px) {
.sidebar {
width: 60%;
}
}
3. More mixins:---------------------------------------------------------------------
@mixin make-property($property, $value) {
@each $prefix in -webkit-, -moz-, -ms-, -o-, '' {
#{$prefix}#{$property}: $value;
}
}
@mixin display ($disp: null, $padding: null, $margin: null) {
display: $disp;
padding: $padding;
margin: $margin;
}
@mixin center-block {
display: block;
margin-left: auto;
margin-right: auto;
}
@mixin set-padding ($t-padding: null,$r-padding: null,$b-padding: null ,$l-padding: null) {
padding-top: $t-padding;
padding-right: $r-padding;
padding-buttom: $b-padding;
padding-left: $l-padding;
}
@mixin set-margin ($t-margin: null,$r-margin: null,$b-margin: null, $l-margin: null) {
margin-top: $t-margin;
margin-right: $r-margin;
margin-buttom: $b-margin;
margin-left: $l-margin;
}
@mixin gradient-background ($startColor: $gray-dark, $endColor: $body-bg) {
background-color: $startColor;
background: -webkit-gradient(linear, left top, left bottom, from($startColor), to($endColor));
background: -webkit-linear-gradient(top, $startColor, $endColor);
background: -moz-linear-gradient(top, $startColor, $endColor);
background: -ms-linear-gradient(top, $startColor, $endColor);
background: -o-linear-gradient(top, $startColor, $endColor);
filter: progid:DXImageTransform.Microsoft.gradient( startColorstr='#{$startColor}',
endColorstr='#{$endColor}', GradientType=0 );
}
@mixin horizontal-gradient-background ($startColor: $gray-dark, $endColor: $body-bg) {
background-color: $startColor;
background-image: -webkit-gradient(linear, left top, right top, from($startColor), to($endColor));
background-image: -webkit-linear-gradient(left, $startColor, $endColor);
background-image: -moz-linear-gradient(left, $startColor, $endColor);
background-image: -ms-linear-gradient(left, $startColor, $endColor);
background-image: -o-linear-gradient(left, $startColor, $endColor);
}
@mixin gradient-background ($from,$to,$middle:null,$fpecnt:null,$tpecnt:null,$mpecnt:null) {
background: $to;
background: -moz-linear-gradient
(top, $to $tpecnt, $from $fpecnt, $middle $mpecnt);
background: -webkit-linear-gradient
(top, $to $tpecnt, $from $fpecnt, $middle $mpecnt);
background: -o-linear-gradient
(top, $to $tpecnt, $from $fpecnt, $middle $mpecnt);
background: -ms-linear-gradient
(top, $to $tpecnt, $from $fpecnt, $middle $mpecnt);
background: linear-gradient
(to bottom, $to $tpecnt, $from $fpecnt, $middle $mpecnt);
filter: progid:DXImageTransform.Microsoft.gradient
( startColorstr='#{$to}', endColorstr='#{$middle}', GradientType=0 );
}
@mixin text-shadow ($string: 1px 1px 1px rgba(200,200,200,0.9)) {
text-shadow: $string;
}
@mixin text-truncate {
overflow: hidden;
text-overflow: ellipsis;
white-space: nowrap;
}
@mixin box-shadow ($string) {
@include make-property(box-shadow, $string);
}
@mixin drop-shadow ($x: 0, $y: 1px, $blur: 2px, $spread: 0, $alpha: 0.25) {
-webkit-box-shadow: $x $y $blur $spread rgba(0, 0, 0, $alpha);
-moz-box-shadow: $x $y $blur $spread rgba(0, 0, 0, $alpha);
box-shadow: $x $y $blur $spread rgba(0, 0, 0, $alpha);
}
@mixin inner-shadow ($x: 0, $y: 1px, $blur: 2px, $spread: 0, $alpha: 0.25) {
-webkit-box-shadow: inset $x $y $blur $spread rgba(0, 0, 0, $alpha);
-moz-box-shadow: inset $x $y $blur $spread rgba(0, 0, 0, $alpha);
box-shadow: inset $x $y $blur $spread rgba(0, 0, 0, $alpha);
}
@mixin box-sizing ($type: border-box) {
@include make-property(box-sizing, $type);
}
@mixin border-radius ($radius: 4px) {
@include make-property(border-radius, $radius);
-moz-background-clip: padding;
-webkit-background-clip: padding-box;
background-clip: padding-box;
}
@mixin border-top-radius($radius) {
-webkit-border-top-right-radius: $radius;
border-top-right-radius: $radius;
-webkit-border-top-left-radius: $radius;
border-top-left-radius: $radius;
-moz-background-clip: padding;
-webkit-background-clip: padding-box;
background-clip: padding-box;
}
@mixin border-right-radius($radius) {
-webkit-border-bottom-right-radius: $radius;
border-bottom-right-radius: $radius;
-webkit-border-top-right-radius: $radius;
border-top-right-radius: $radius;
-moz-background-clip: padding;
-webkit-background-clip: padding-box;
background-clip: padding-box;
}
@mixin border-bottom-radius($radius) {
-webkit-border-bottom-right-radius: $radius;
border-bottom-right-radius: $radius;
-webkit-border-bottom-left-radius: $radius;
border-bottom-left-radius: $radius;
-moz-background-clip: padding;
-webkit-background-clip: padding-box;
background-clip: padding-box;
}
@mixin border-left-radius($radius) {
-webkit-border-bottom-left-radius: $radius;
border-bottom-left-radius: $radius;
-webkit-border-top-left-radius: $radius;
border-top-left-radius: $radius;
-moz-background-clip: padding;
-webkit-background-clip: padding-box;
background-clip: padding-box;
}
@mixin border-radiuses ($topright: 0, $bottomright: 0, $bottomleft: 0, $topleft: 0) {
-webkit-border-top-right-radius: $topright;
-webkit-border-bottom-right-radius: $bottomright;
-webkit-border-bottom-left-radius: $bottomleft;
-webkit-border-top-left-radius: $topleft;
-moz-border-radius-topright: $topright;
-moz-border-radius-bottomright: $bottomright;
-moz-border-radius-bottomleft: $bottomleft;
-moz-border-radius-topleft: $topleft;
border-top-right-radius: $topright;
border-bottom-right-radius: $bottomright;
border-bottom-left-radius: $bottomleft;
border-top-left-radius: $topleft;
-moz-background-clip: padding;
-webkit-background-clip: padding-box;
background-clip: padding-box;
}
@mixin set-border-radius
($tl-radius: null,$bl-radius: null,$tr-radius: null,$br-radius: null) {
border-top-left-radius: $tl-radius;
border-bottom-left-radius: $bl-radius;
border-top-right-radius: $tr-radius;
border-bottom-right-radius: $br-radius;
}
@mixin set-flexbox () {
display: -webkit-box;
display: -webkit-flex;
display: -moz-box;
display: -ms-flexbox;
display: flex;
flex: 1 auto;
}
@mixin link-hover {
background-color: $link-background-color;
color: $link-hover-color;
}
@mixin opacity ($opacity: 0.5) {
-webkit-opacity: $opacity;
-moz-opacity: $opacity;
opacity: $opacity;
}
@mixin clearfix() {
&:before,
&:after {
content: "";
display: table;
}
&:after {
clear: both;
}
}
@mixin set-position ($position,$top: auto, $right: auto, $bottom: auto, $left: auto) {
top: $top;
right: $right;
bottom: $bottom;
left: $left;
position: $position;
}
4. Generate Icons:---------------------------------------------------------------------
You can generate icon by passing index and size. Here, I have commented above coding line. This will be very helpful to you because over online, this method is not available for you.
Mixins
@mixin icon($index, $size) {
line-height: 1;
vertical-align: middle;
&:before {
// Manually define the icon set */
$columns: 5;
background-image: url(icons/large/sprite.png);
background-size: $columns * 100%;
width: #{$size}px;
height: #{$size}px;
background-position: #{(100/($columns - 1)*($index - 1))}% 0;
content: "";
margin-right: #{$size/4}px;
display: inline-block;
line-height: #{$size}px;
vertical-align: middle;
}
}
Functions
Functions is different from mixins. It has return type. Here's an example of functions.
Functions
@function pxtoem($target, $context){
@if $target == 0 { @return 0 }
@return ($target/$context)+0em;
}
Uses
$font-size-base: 11px;
.side-bar{
font-size: pxtoem(14px,$font-size-base);
}
Output
.side-bar {
font-size: 1.27273em;
}
Some Useful Functions
@function pxtoem($target, $context){
@if $target == 0 { @return 0 }
@return ($target/$context)+0em;
}
@function emtopx($target, $context){
@if $target == 0 { @return 0 }
@return ($target*$context)+0px;
}
@function font-size($sizeValue: $font-size-base) {
@return ($sizeValue / $font-size-base) * 100%;
}
@function line-height($heightValue: $line-height-base ){
@return $heightValue;
}
@function element-height($heightValue: $element-height ){
@return $heightValue;
}
Extend/Inheritance
This is one of the most useful features of SASS. Using @extend
to share a set of CSS properties from one selector to another. Here is simple example.
.message {
border: 1px solid #ccc;
padding: 10px;
color: #333;
}
.success {
@extend .message;
border-color: green;
}
Output
.message, .success {
border: 1px solid #ccc;
padding: 10px;
color: #333;
}
.success {
border-color: green;
}
Operators
+, -, *, /, and % is used for SASS. Here is the example for SASS.
.error{
width: 600px / 960px * 100%;
}
Colors
There is most important color converter methods in SASS like DARKEN
, LIGHTEN
, SATURAT
, DESATURATE
, ADJUST
, HUE
. Here is the example.
$red: #ff0000;
$red-dark: darken($red,10%);
$red-light: lighten($red,20%);
$red-desaturate: desaturate($red, 28);
$red-hue: adjust_hue(desaturate($red, 28), 189);
h1{
background-color:$red;
}
h2{
background-color:$red-light;
}
h3{
background-color:$red-dark;
}
h4{
background-color:$red-desaturate;
}
h5{
background-color:$red-hue;
}
Output
h1 {
background-color: #ff0000;
}
h2 {
background-color: #ff6666;
}
h3 {
background-color: #cc0000;
}
h4 {
background-color: #db2424;
}
h5 {
background-color: #24c0db;
}
There is a very nice online tools for color converter. you can use from here.
Not Familiar in SASS
If you are not familiar in SASS, you can use the online tools for creating SASS. You will just write CSS code, this tool will convert SASS code. This tool is for beginners. If you are an expert, then there is no need to write CSS code. It is only for SASS code practicing for beginners that have not used SASS before.
Tools
Conclusion
I think this article will be very helpful for beginners who want to learn SASS. I am not good at English, if I have made any mistakes, please forgive me. Thanks for having patience.
I am expert in AngularJs, KnockoutJs, Breezejs, NodeJS, ExpressJS, Javascript, jQuery, JSON, Html5, CSS3, Asp.Net (WebForm, MVC), WCF, Restful service, EF, C# .NET, XAML, XML, UML, SQL-SERVER, MongoDB