A Simple Graph Control
A simple control to draw graphs of points as a function of time
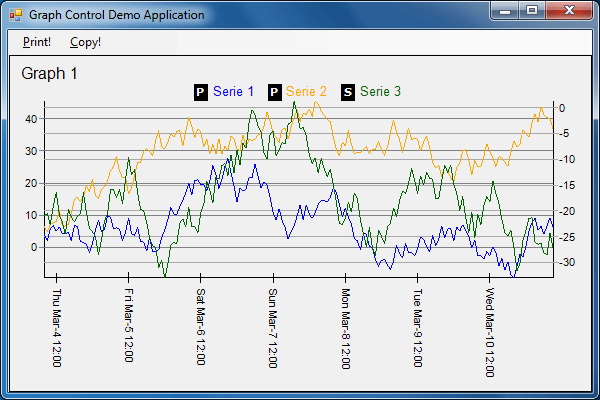
Introduction
Here's yet another graph control. I had to write it because I did not find any other easy to use Graph control which met my needs. This one may be the one you are looking for. I think the code is quite fast too.
This code is not too heavy, you can easily integrate it into your project. This code can also be used as a separate module that you can link to your own project.
Using the Code
First of all, add the using
clause:
using WindowsFormsControlLibrary;
Creating the Control
The first thing to do is to create an instance of the control.
There are three constructors:
- The default constructor
public GraphControl()
using Arial fonts, as follows:
_CurveFont = new Font("Arial", 15);
_CurveLegendFont = new Font("Arial", 10);
_AxeFont = new Font("Arial", 8);
public CurveControl(Font TitleFont, Font CurveLegendFont, Font AxeFont)
public GraphControl(Font TitleFont, Font GraphLegendFont,
Font AxeFont, string DTFormat)
The Graph control can then be created as follows:
//Create a Graph Control
GraphCtrl = new GraphControl(new Font("Arial", 12),
new Font("Arial", 10), new Font("Arial", 8));
GraphCtrl.TabIndex = 0;
GraphCtrl.Dock = DockStyle.Fill;
GraphCtrl.Name = "Graph 1";
//Add Graph Control to the form or a panel on the form
GraphPanel.Controls.Add(GraphCtrl);
Adding Series
The Graph control contains three main properties:
- A dictionary of a series of points
- The primary axis
- The secondary axis
It is needed to add (create) the series of points which will be empty at the beginning. To do so, call the AddPointsSerie
method:
//Add series
GraphCtrl.AddPointsSerie("Serie1", Axes.VerticalPrimary, "Serie 1", Color.Blue);
GraphCtrl.AddPointsSerie("Serie2", Axes.VerticalPrimary, "Serie 2", Color.Orange);
GraphCtrl.AddPointsSerie("Serie3", Axes.VerticalSecondary, "Serie 3", Color.DarkGreen);
The AddPointsSerie
method takes four parameters:
- A key used to identify the series
- The vertical axis used to display the series
- The displayed label of the series
- The color of the series
The axis is taken from the two vertical axes available in the Axes
enum.
Adding Points
It is now needed to populate the series with the points.
To retrieve the series, use the GetPointsSerie
method, then call one of the three AddPoint
methods:
GraphCtrl.GetPointsSerie("Serie1").AddPointD(dt.AddHours(i), y1);
The three AddPoint
methods are:
-
public void AddPointF(DateTime t, float Y)
-
public void AddPointD(DateTime t, double Y)
-
public void AddPointS(DateTime t, string Y)
Add a point whose Y value is a float
.
Add a point whose Y value is a double
.
Add a point whose Y value is a float provided as a string
. If an invalid string is given, the point is ignored.
Updating the Display
If anything is changed like adding points to series, for example, you need to Invalidate
the control:
CC.Invalidate();
Changing the Axis of a Series
It may be needed to change the axis a series is associated with. To do so, call the SetSerieAxe
method:
CC.SetSerieAxe("Serie2", Axes.VerticalPrimary);
Clearing a Series
CC.GetPointsSerie("Serie2").ClearPointsArray();
Removing a Series
CC.RemovePointsSerie("Serie2");
Printing and Copying to the Clipboard
It is possible to print the Graph or copy the Graph to the clipboard using the new method:
public void DrawGraph(Graphics g, Rectangle bounds)
The method takes 2 parameters:
- A Graphics object
- A Rectangle to draw the Graph onto
Using the Method to Print
private void PrintDocument1_PrintPage
(object sender, System.Drawing.Printing.PrintPageEventArgs e)
{
GraphCtrl.DrawGraph(e.Graphics, e.MarginBounds);
}
Using the Method to Copy to the Clipboard
private void CopyToolStripMenuItem_Click(object sender, EventArgs e)
{
Bitmap Bmp = new Bitmap(800, 400); Bmp.SetResolution(100, 100);
Graphics g = Graphics.FromImage(Bmp);
g.Clear(Color.White);
GraphCtrl.DrawGraph(g, new Rectangle(new Point(0, 0), Bmp.Size));
Clipboard.SetImage(Bmp);
}
Interactivity
Graph Displayed Name
The user has the ability to double click on the Graph displayed name to change it.
Serie Selection
Clicking on or close to a serie selects it.
History
- February 25, 2010 - Article first published
- March 3, 2010
- Added capability to the control to re-draw itself when resized
- Created a separated
Draw
function to allow its usage for Painting, Printing, Copying... - Changed default format to display dates on the horizontal axis and added a 3rd constructor to specify it if needed
- Added
MajorUnitDateTime
in theGraphAxe
class, not yet used - Renamed everything using Graph instead of Curve