In this post, we will take a look at objects-as-maps and show their drawbacks and how the Map collection saves the day. We will see the different methods and how to interact with them, and finally, we will see how to iterate over a Map collection with the use of for-of loops and spread operator.
Introduction
If you are familiar with other languages such as C#, Java, or C++, JavaScript’s map data-structure is similar to a dictionary (dictionary is the equivalent of JavaScript Map
in other programming languages). Knowing the concepts of key/value pair data structures in other languages will help you instantly to grasp the basic concepts here. However, if you haven’t touched any of these languages, it won’t hurt because we will still start from the basics.
Lastly, if you are one of those developers who remain comfortable with objects-as-maps and decided to see if the JavaScript Map
can save your day, you are reading the right article.
Ok, then let’s get started.
Prior to JavaScript Maps
Before Map
was introduced to the JavaScript language, object
was the primary way for creating a key/value pair data structures. However, the major drawback of using this technique is the inability to use a non-string value as the key. Let’s see an example.
var sqlServer = {};
var firstInstance = { id: 1 };
var secondInstance = { id: 2 };
sqlServer[firstInstance] = "SQLServer1";
sqlServer[secondInstance] = "SQLServer2";
The main idea here is that the two objects, firstInstance
and secondInstance
, both resulted into "[object Object]"
. Thus, only one key was set in sqlServer
. Let us see this in action below.
Output
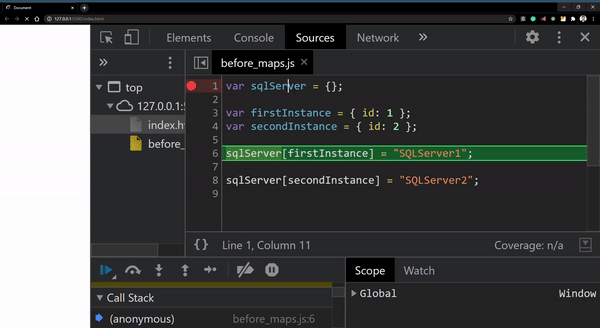
Of course, creating your own implementation of clone-maps or reinventing the wheel may cause you more time, especially when complexity enters the picture. However, today, we can use the JavaScript-Map object collection to help us deal with key/value pairs.
What is a JavaScript Map?
Fundamentally, a Map
is a collection of key/value pairs. These keys and values are of any data-type.
How to Create a Map?
It is easy to create a new Map
in JavaScript. Let’s see an example.
let myMap = new Map();
console.log(myMap);
Output

As you can see, we just created an empty Map
. That’s it! Just by using the Map
constructor, you can directly create a new Map
in JavaScript.
How to Initialize Map?
How about creating and initializing a new Map
with data? There are various ways to initialize it. Let’s see them one by one.
Using an Array
let topProgrammingLanguages = new Map([
[1, 'JavaScript'],
[2, 'Python'],
[3, 'Java'],
[4, 'C#'],
[5, 'C']
]);
console.log(topProgrammingLanguages);
Output

Using the set() Method
let myFavoriteBooks = new Map();
myFavoriteBooks.set(1, 'Rich Dad Poor Dad');
myFavoriteBooks.set(2, 'The Magic of Thinking Big');
myFavoriteBooks.set(3, 'Think and Grow Rich');
myFavoriteBooks.set(4, 'How to Win Friends & Influence People');
myFavoriteBooks.set(5, 'Shoe Dog');
console.log(myFavoriteBooks);
Output

Using the get, has, includes, clear and delete Methods
Using the get() Method
This method returns the associated value of the key
and if doesn’t exist, returns undefined
.
let sqlServerInstances = new Map();
sqlServerInstances.set('SQL_DEV_Instance', 'MS_SQLSERVER_1');
sqlServerInstances.set('SQL_UAT_Instance', 'MS_SQLSERVER_2');
sqlServerInstances.set('SQL_PROD_Instance', 'MS_SQLSERVER_3');
console.log(sqlServerInstances.get("SQL_DEV_Instance"));
console.log(sqlServerInstances.get('SQL_UAT_Instance'));
console.log(sqlServerInstances.get("SQL_PROD_Instance"));
Using the has() Method
This method checks whether the key
exists within the Map
.
let sqlServerInstances = new Map();
sqlServerInstances.set('SQL_DEV_Instance', 'MS_SQLSERVER_1');
sqlServerInstances.set('SQL_UAT_Instance', 'MS_SQLSERVER_2');
sqlServerInstances.set('SQL_PROD_Instance', 'MS_SQLSERVER_3');
console.log(sqlServerInstances.has("SQL_PROD_Instance"))
console.log(sqlServerInstances.has("SQL_PROD2_Instance"))
Using the clear() Method
This method clears the entire Map
collection.
let products = new Map();
products.set("PRODUCT_001", { name: "Product 1" });
products.set("PRODUCT_002", { name: "Product 2" });
products.set("PRODUCT_003", { name: "Product 3" });
console.log(products.size);
products.clear();
console.log(products.size);
Using the delete() Method
This method removes the key-value pair inside the Map
.
let sqlServerInstances = new Map();
sqlServerInstances.set('SQL_DEV_Instance', 'MS_SQLSERVER_1');
sqlServerInstances.set('SQL_UAT_Instance', 'MS_SQLSERVER_2');
sqlServerInstances.set('SQL_PROD_Instance', 'MS_SQLSERVER_3');
console.log(sqlServerInstances.get('SQL_UAT_Instance'));
console.log(sqlServerInstances.delete('SQL_UAT_Instance'));
console.log(sqlServerInstances.has('SQL_UAT_Instance'));
console.log(sqlServerInstances.get('SQL_UAT_Instance'));
Ways to Iterate Over a Map
In this section, we are going to see how to iterate over a Map. However, before that, we need to discuss the following methods: keys, values and entries. This will all make sense when we started to see how to use these methods when iterating the map using the for-of
loop.
Using the Keys, Values and Entries Methods
A reminder, the code sample below will be utilized as our source of data.
let myFavoriteBooks = new Map();
myFavoriteBooks.set(1, 'Rich Dad Poor Dad');
myFavoriteBooks.set(2, 'The Magic of Thinking Big');
myFavoriteBooks.set(3, 'Think and Grow Rich');
myFavoriteBooks.set(4, 'How to Win Friends & Influence People');
myFavoriteBooks.set(5, 'Shoe Dog');
Using the keys() Method
This method returns the keys for each element in the Map
object. Moreover, if you simply need the keys of the Map
collection, this a convenient method for that case, especially when iterating through the keys.
const myMap1 = new Map([[1, 'red'], [2, 'blue']]);
console.log(myMap1.keys());
Iterate Over keys
for (const key of myFavoriteBooks.keys()) {
console.log(key);
}
Using the values() Method
This method return the values for each element in the Map
object. Moreover, like the keys
method which is an exact opposite of this method, that is solely focus on getting the values of the Map
collection.
const myMap2 = new Map([['Electronic Gadget', 'Smart Phone'], ['Input Devices', 'Mouse']]);
console.log(myMap2.values());
Iterate Over values
for (const value of myFavoriteBooks.values()) {
console.log(value);
}
Using the entries() Method
This method return the object that contains the [key,value]
pairs for each element in the Map
collection.
const myMap3 = new Map([['Samsung', 'Smart Phone'],
['Colgate', 'Toothpaste'], ['Coke', 'Soda']]);
console.log(myMap3.entries());
Iterate Over entries
for (const [key, value] of myFavoriteBooks.entries()) {
console.log(key, value);
}
Spreading a Map
Now, at last, we are in the last section of our article. Using the spread operator (...
), we can easily spread our map like the example below:
let fastFoods = new Map([[1, 'McDO'], [2, 'Burger King'], [3, 'KFC'],
[4, 'Wendys'], [5, 'Pizza Hut']]);
console.log(...fastFoods.keys());
console.log(...fastFoods.values());
Hopefully, you can guess what are the outputs of the sample code above. If you have read and understood the previous sections of this article, you can guess it. By the way, if you are confident with your answer, you can comment below. Thanks.
Summary
In this post, we have tackled the JavaScript Map
object collection. We have started with objects-as-maps and have shown their drawbacks and how the Map
collection saves the day. Moreover, we have seen the different methods and how to interact with them. Lastly, we have seen how to iterate over a Map
collection with the use of for-of
loops and spread operator (...
).
I hope you have enjoyed this article, as I have enjoyed writing it. Stay tuned for more. Don’t forget to subscribe, if you haven’t subscribed yet. Many thanks, until next time, happy programming!
History
- 1st September, 2020: Initial version
Jin humbles himself as a C# programmer and a web developer, who loves backend and middleware development and still improving his skills at the front-end arena. He loves what he does, but far from perfect, here is a list of what he loves to do: read, write and code.