The article describes how to implement Google.Apis.Drive.v3 in WinForm Project, which uses Microsoft Access Database 2007. The project simply manipulates user data from / within the database with simple actions, uses TreeView control to display Data from Database on the Form, and backs up the database locally and to Google Drive.
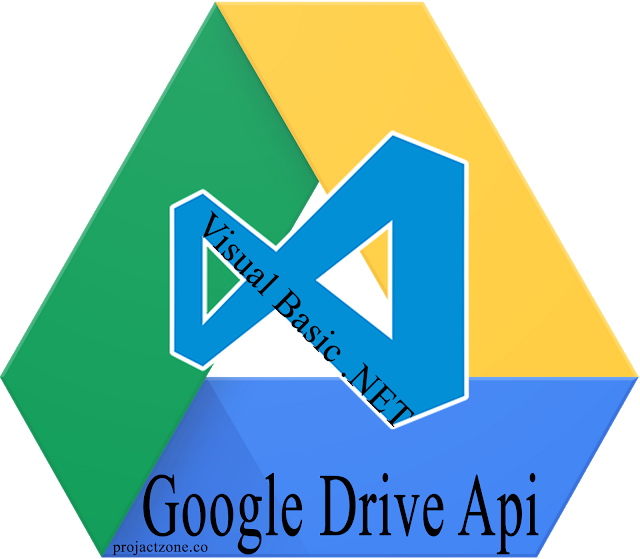
Requirements
- Visual Basic 2012 [recommended VS 2015] or above (Visual Studio 2019) from here
- Visual Basic previous example from here
- Google Account from here
- .NET Framework 4.5+ or above. "Async Programming"
- Google Drive Api [Google.Apis.Drive.v3] from NuGet here
Open VB 2015 Project in VB 2019
Can I open / migrate Visual Basic 2015 Project Source Code with Visual Basic 2019?
The answer is Yes, if you want to more details about VB 2015 to VB 2019 migration, please read this article.
VB.NET Google Drive Api v3 Post Key Points
Project Description
I will use the VB.NET example from the previous post [Backup Database to Google Drive] to add another functionality to it. I will add Backup Database to Google Drive [ToolStripMenuItem
].

VB.NET Google Drive Api Source Code Example
When a user press [ToolStripMenuItem
-> Backup to Google Drive]: The VB.NET application does the following:
- Checks for Internet Connection Status
- Connects to Google Drive project created in Google developer section [App Console]
- Uploads the Microsoft Access 2007 (*.accdb) database file from the previous post to remote folder [/Backups] created manually by me in my Google Drive account.
- Returns a string [File uploaded to Google Drive] to verify
[UploadFile]
method.
Installing Google Drive Api (Google.Apis.Drive.v3) from Visual Studio 2015
To install latest Google.Apis.Drive.v3 from Visual Basic 2015
- Navigate to (https://www.nuget.org/packages/Google.Apis.Drive.v3/1.41.1.1708) to obtain the latest Google Drive Api version 3.
- Copy the PM code (PM> Install-Package Google.Apis.Drive.v3 -Version 1.41.1.1708)
- Open Visual Basic 2015 as Administration
- Choose NuGet package manager -> Package manage console

VB .NET Google Drive API installation using NuGet in VS2015
- In the console manager in Visual Studio, paste the PM Code and press <Enter>.

VB .NET Google.Apis.Drive.v3 installation using NuGet in VS2015
- Wait for
Google.Apis.Drive.v3
download to 100% complete and restart Visual Studio 2015, to ensure that you successfully installed 'Google.Apis.Drive.v3
' to [HierarchicalTreeView] project. - Now, in your Visual Basic 2015 Solution Explorer panel [References] would looks something like this:

VB.NET Google.Apis.Drive.v3 installation using NuGet in VS2015 Reference
Create Google Console Project for .NET to Use Google Drive API

Google API Console Create new project

Google Drive API Project Overview
In the above picture, make sure your Google Drive API is present at the bottom of the page.
What Do We Need From Google Console API?

VB.NET Google Console API - Drive API
- In order to start coding your application, you need some data from Google Console API to use in your VB.NET project:
You need to create OAuth 2.0 client IDs from Credentials section. For installed applications (like desktop applications), you will not need to specify any information in the OAuth consent screen only the application name and your Google email address.

Your Google Console API Credentials Section
- Application Name (Backup-DB-Desktop) at the top of the page
- Client ID (something.apps.googleusercontent.com)
- Client Secret (Found when you click on OAuth Name)
VB.NET Google Drive API Desktop Project Code
- We create a Service using those credentials above.
- We upload the file.
- Check your Google Drive account associated with your original Google Account Email.
- I used a small file to upload.
- I will also include "Google Drive Resumable Upload"
ChunkedUpload()
method in my source code example for my 13MB Access database file.
VB.NET Google Drive API Desktop Project Run
When you run the application for the first time, you will go through some security issues like:
- Google will prompt you to verify access from another application.
- Refresh
AccessToken
is done via your browser, so you will notice that your browser will open for refreshing accesstoken. - Those would occur only once.
Try the QuickStart example first, this example is designed only for testing.

VB.NET Google drive API v3 OAuth 2.0 Quick Start Example Permission

VB.NET Google drive API v3 OAuth 2.0 allow access

VB.NET Google drive API v3 OAuth 2.0 received verification code received
VB.NET Google Drive Api v3 OAuth2 vs Service Account
Authentication is necessary if you want to access Google Drive API. There are 2 types of Authentications:
- OAuth2 : Will allow you to access another users data,
- Service Account : Can be set up to access your own data.
VB.NET Google Drive API v3 QuickStart .NET Example Source Code
Imports Google.Apis.Auth.OAuth2
Imports Google.Apis.Drive.v3
Imports Google.Apis.Drive.v3.Data
Imports Google.Apis.Services
Imports Google.Apis.Util.Store
Imports System.IO
Imports System.Threading
Module Module1
Dim Scopes() As String = {DriveService.Scope.DriveReadonly}
Dim ApplicationName As String = "Quickstart"
Private Service As DriveService = New DriveService
Public Sub Main()
Dim credential As UserCredential
Using Stream = New FileStream("credentials.json", FileMode.Open, FileAccess.Read)
Dim credPath As String = "token.json"
credential = GoogleWebAuthorizationBroker.AuthorizeAsync(
GoogleClientSecrets.Load(Stream).Secrets,
Scopes,
"user",
CancellationToken.None,
New FileDataStore(credPath, True)).Result
Console.WriteLine("Credential file saved to: " + credPath)
End Using
Dim Service = New DriveService(New BaseClientService.Initializer() With
{
.HttpClientInitializer = credential,
.ApplicationName = ApplicationName
})
Dim listRequest As FilesResource.ListRequest = Service.Files.List()
listRequest.PageSize = 10
listRequest.Fields = "nextPageToken, files(id, name)"
Dim files As IList(Of Data.File) = listRequest.Execute().Files
Console.WriteLine("Files:")
If (files IsNot Nothing And files.Count > 0) Then
For Each file In files
Console.WriteLine("{0} ({1})", file.Name, file.Id)
Next
Else
Console.WriteLine("No files found.")
End If
Console.Read()
End Sub
End Module
VB.NET Google Drive API TroubleShooting
Error
VB.NET Google Drive API Error (At least one client secrets (Installed or Web) should be set)
Diagnostic
You are getting this error because you are trying to use UserCredential instead of GoogleCredential. In the Quick Start Example for Google Drive API Dotnet:
Solution
Dim Credential As UserCredential
UserCredential credential;
Change the above code to:
Dim Credential as GoogleCredential
GoogleCredential Credential;
Update
- I have found and converted to VB.NET, the C# Resumable Upload for large files uploading.
- I've uploaded an example VB.NET source code on uploading large files to Google Drive using the v3 API.
Source Code Password
projectzone.co