Introduction
In this article, you will learn how to search in a GridView
using custom controls from a DLL. We can use them as usercontols like a .ascx.
How to add a JavaScript file to a DLL
- Like in the previous article[^], first create a new project and select a Class Library project.
Figure 1. Add Class Library Project
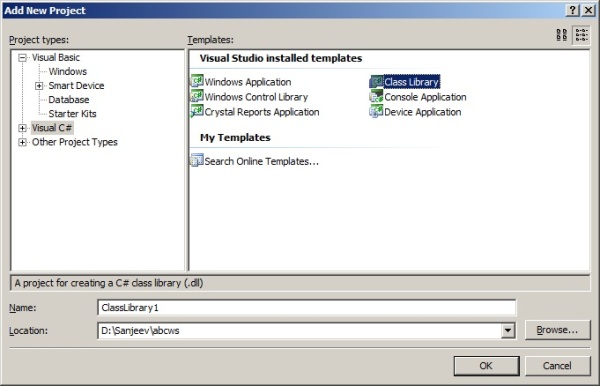
- Like in the previous article[^], add a JavaScript file and in the Properties window, set properties as in figure 2.

- Like in the previous article[^], now in AssemblyInfo.cs file, add the following lines. Add exactly the same name as the js file. Below is the code.
[assembly: System.Web.UI.WebResource("AddUserControl.JS_SearchInGrid.js",
"text/javascript", PerformSubstitution = true)]
- Now we write code for creating a custom control textbox with a
GridView
control.
We will create a TextBox
with a GridView
and when we type in the textbox our focus automatically goes to the GridView
matched item. And when we press Down arrow, the cursor moves to the next row. When we press the Up arrow, the cursor moves to the row above.
In Figure 1, set cursor on the Abrasives and emery stone row.

In Figure 2, when we press Down arrow key, the cursor moves on to the Acetals -whether or .. row.

We write [ToolboxData(@"<{0}:SearchInGrid_TextBox runat=""server"" \>")]
. By ToolboxData
we specify the tag which is added to the page when this control is dragged form the toolbox to the form. We use AutoCompleteType
which enables us to associate an AutoComplete class with the TextBox
control.
[ToolboxData(@"<{0}:SearchInGrid_TextBox runat=""server"" \>")]
public class SearchInGrid_ByTextBox : TextBox
{
private string _GridView_Id = "";
private string _SearchCol = "0";
private bool _ShowHeader = false;
public SearchInGrid_ByTextBox()
: base()
{
this.AutoCompleteType = AutoCompleteType.Disabled;
}
[Category("Behavior"),
DefaultValue(""),
Description("The GridView's Id In which Search Data on key press.")]
public string GridView_Id
{
get { return _GridView_Id; }
set { _GridView_Id = value.Trim(); }
}
[Category("Behavior"),
DefaultValue(""),
Description("The GridView's Column Number In which Search Data.")]
public string SearchCol
{
get { return _SearchCol; }
set { _SearchCol = value.Trim(); }
}
[Category("Behavior"),
DefaultValue(""),
Description("The GridView's Show Header Property by which " +
"we search data from 0 zero or 1 row.")]
public bool ShowHeader
{
get { return _ShowHeader; }
set { _ShowHeader = value; }
}
private void setCtrl_Attribute(string Attr_name, string value)
{
if (Attr_name != null && Attr_name != "" && value != null && value != "")
{
if (this.Attributes[Attr_name] != null && this.Attributes[Attr_name] != "")
{
if (this.Attributes[Attr_name].IndexOf(value, StringComparison.OrdinalIgnoreCase) == -1)
this.Attributes[Attr_name] = value + ";" + this.Attributes[Attr_name];
}
else
this.Attributes[Attr_name] = value + ";";
}
}
protected override void AddAttributesToRender(System.Web.UI.HtmlTextWriter writer)
{
base.AddAttributesToRender(writer);
}
protected override void OnPreRender(EventArgs e)
{
if (GridView_Id != "")
{
setCtrl_Attribute("onkeyup", "Search_InGrid_ByTextBox('" +
this.ClientID + "','" + Page.FindControl(GridView_Id).ClientID +
"','" + ShowHeader.ToString() + "','" +
SearchCol.ToString() + "')");
}
Page.RegisterRequiresPostBack(this);
Page.ClientScript.RegisterClientScriptInclude("SanSearch_ControlsJS",
Page.ClientScript.GetWebResourceUrl(this.GetType(), "AddUserControl.JS_SearchInGrid.js"));
base.OnPreRender(e);
}
}
GridView_Id
: By this we assign the GridView
's ID to this textbox in which we find the matched value and set focus on this item of the GridView
. SearchCol
: By this we set the column in which we search the textbox values. ShowHeader
: By this we check that showheader
of the GridView
property to specify whether it is true or not. If true then we search from row number 1, else we search from row zero.
We call the Page.RegisterRequiresPostBack()
method inside (or before) the control's PreRender()
event to tell page that our control is interested for receiving postback data. All the client side functionalities happen in an external JavaScript file. A JavaScript included is rendered by the control in its OnPreRender()
method. This line is included in the JavaScript file:
Page.ClientScript.RegisterClientScriptInclude("SanControlsJS",
Page.ClientScript.GetWebResourceUrl(this.GetType(), "AddUserControl.JS_SearchInGrid.js"));
setCtrl_Attribute("onkeyup", "Search_InGrid_ByTextBox('" + this.ClientID + "','" +
Page.FindControl(GridView_Id).ClientID + "','" + ShowHeader.ToString() + "','" + SearchCol.ToString() + "')");
By this we add an attribute to the textbox control. When we enter data in textbox then the Search_InGrid_ByTextBox
JavaScript function searches in the GridView
's particular column textbox's value. Now we will create a GridView
in which we find the value of the textbox.
[ToolboxData(@"<{0}:Search_GridView runat=""server"" \>")]
public class Search_GridView : GridView
{
bool isrendered = false;
public Search_GridView()
: base()
{ }
protected override void AddAttributesToRender(System.Web.UI.HtmlTextWriter writer)
{
isrendered = true;
base.AddAttributesToRender(writer);
}
protected override void OnPreRender(EventArgs e)
{
if (!isrendered)
{
Page.ClientScript.RegisterClientScriptInclude("SanSearch_ControlsJS",
Page.ClientScript.GetWebResourceUrl(this.GetType(),
"AddUserControl.JS_SearchInGrid.js"));
base.OnPreRender(e);
if (HttpContext.Current.Request.Form["SanSearch_Controls_HdnField"] == null)
Page.ClientScript.RegisterHiddenField("SanSearch_Controls_HdnField", "");
else
Page.ClientScript.RegisterHiddenField("SanSearch_Controls_HdnField",
HttpContext.Current.Request.Form["SanSearch_Controls_HdnField"]);
}
}
protected override void InitializeRow(GridViewRow row, DataControlField[] fields)
{
row.Style.Add(HtmlTextWriterStyle.Cursor, "hand");
row.Attributes.Add("onmouseover", "setMouseover(" + row.RowIndex +
"," + base.ClientID + ")");
row.Attributes.Add("onmouseout", "setMouseout(" + row.RowIndex +
"," + base.ClientID + ")");
row.Attributes.Add("onclick", "SetGridOnClick(" + base.ClientID +
"," + row.RowIndex + ")");
base.InitializeRow(row, fields);
}
}
The InitializeRow
method is overridden. This method is responsible for creating all the controls' row that the custom field contains. In this method we assign the GridView
's row JavaScript functions: row.Style.Add(HtmlTextWriterStyle.Cursor, "hand");
. By this when we move the cursor on a row, the cursor converts into a hand cursor. We assign the mouseover and mouseout events in which we change the color of the respective row.
See the mouseover effect

We build the DLL then add New Website and add a reference of this DLL. In our aspx page we register the assembly as we use user controls like same. Here is the .aspx page design:
<%@ Page Language="C#" AutoEventWireup="true"
CodeFile="Default.aspx.cs" Inherits="_Default" %>
<%@ Register Assembly ="AddUserControl" Namespace="AddUserControl"
TagPrefix ="SANCtrl" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" >
<head runat="server">
<title>Untitled Page</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<SANCtrl:SearchInGrid_ByTextBox runat ="server" ID="sanSearchText"
GridView_Id ="SanSearchGrid" ShowHeader ="true" SearchCol ="1"
></SANCtrl:SearchInGrid_ByTextBox>
<asp:Panel ID="PaneGrid" runat="server"
ScrollBars="auto" Width="99.8%" style="scrollbar-track-color:
whitesmoke;scrollbar-face-color:whitesmoke; scrollbar-shadow-color: gray; scrollbar-face-color:
whitesmoke;" Height ="250px">
<SANCtrl:Search_GridView runat ="server" ID="SanSearchGrid"
ShowHeader ="true" Width="100%" BackColor ="WhiteSmoke"
AutoGenerateColumns="False" CellPadding="0" BorderWidth="1px"
BorderStyle="Solid" BorderColor="Gray" RowStyle-Height ="20px"
>
<Columns>
<asp:TemplateField>
<ItemStyle Width="10%" BorderColor="DodgerBlue" />
<HeaderTemplate >Code</HeaderTemplate>
<ControlStyle Width="96%" />
<ItemTemplate>
<asp:Label ID="LblCode" Font-Size="8pt"
Font-Names="Verdana" runat="server"
Text='<%# Bind("Code") %>'
style="border: none 0px; text-align:center"/>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField>
<ItemStyle Width="90%" BorderColor="DodgerBlue" />
<HeaderTemplate >Commodity Name</HeaderTemplate>
<ControlStyle Width="96%" />
<ItemTemplate>
<asp:Label ID="LblCategoryName" Font-Size="8pt"
Font-Names="Verdana" runat="server"
Text='<%# Bind ("CmdName") %>'
style="border: none 0px; text-align:left" />
</ItemTemplate>
</asp:TemplateField>
</Columns>
<RowStyle Height="20px" />
</SANCtrl:Search_GridView>
</asp:Panel>
</div>
</form>
</body>
</html>
Now the .cs page code for filling the GridView
with data. When we view this page in browser, type in the textbox and our cursor goes to the matched row. We do not require any code for this.
public partial class _Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
FillGrid();
}
}
private void FillGrid()
{
SanSearchGrid.DataSource = GetCommodityList();
SanSearchGrid.DataBind();
}
private DataTable GetCommodityList()
Now see the figure showing the functionality of the textbox value searching in the GridView
.

See this figure of Solution Explorer in which I display the DLL project and a website page for testing the js functions are assigned to ASPX controls.

That's all!
History
i work in ASP.Net,C#,Sql,VB6.0 etc. I want to enhance my skills by learning new technologies.