A few months ago I wrote a post on a component library built entirely in jQuery, but if you don’t like to write lots of javascript and prefer to write as much as possible in server-side code, today I’m writing about something I’ve been looking at lately: the new DevExpress DXv2 v2012 library, specifically the MVC extensions.
Installing and the First Impressions
Let’s start from the installation: it was pretty easy and not many steps to go through. It installed all the components and installed in all the versions of Visual Studio (including VS2012 RC). Was good to see a nice Metro touch on the UI of the various install screens: it seems like we’ll have Metro inspired UIs everywhere in the coming years.
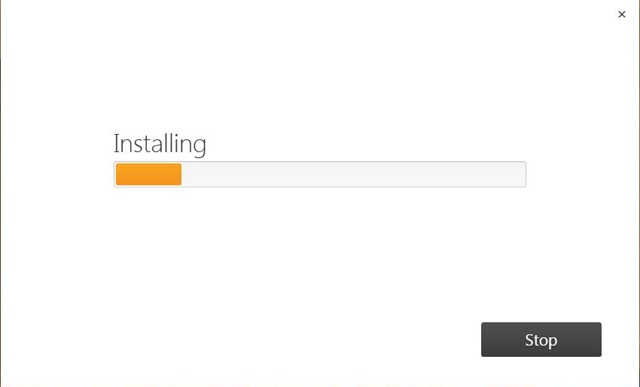

Above you see two screenshots: the first is the aforementioned install screen, while the second brings me to what happens when the install dialog closes: you get a very well thought getting started screen, which links to sample apps and to demo pages. The really nice thing is that, unlike other products I’ve used in the past, when you click on either a end-to-end sample app or on a demo page, a instance of the ASP.NET development server (aka Cassini) starts, and you can immediately start using the app and see the demos (where you can see both the working demo together with the related code).
To learn all about the new features introduced in the 2012 version of the library, you can read the extensive 60 pages of the What’s New in DXperience 12.1 and see the short overview videos.
The First Steps
When you open Visual Studio for the first time and try to create a new project, you’ll notice the new DevExpress project templates in the File-New dialog, and by selecting the Web projects’ group you get 7 project templates, 4 for web forms and 3 for MVC: the project templates names are self-explanatory and they give you a getting-started project wizard too. One of the DevExpress web project templates give you an empty ASP.NET MVC project with all the references to use the DevEx MVC extensions and the last one gives you a Outlook-like interface to build a feature-rich website. The interesting project template is the first one, "Web Application": instead of just creating the project, it opens a custom "wizard" dialog (Metro style of course) that helps you to setup your project, by allowing you to choose the layout of your page (standard, empty, outlook-style or customizable), the view engine (webforms or Razor), the theme and some other lower-level settings.



If you choose the "customizable" layout, you are basically getting the outlook-style layout with custom panes and controls. The Empty layout gives you exactly the same contents as the empty project template while the Standard layout gives you just enough to get you started with a project that runs immediately. It’s also nice that you can choose which version of ASP.NET MVC you want to target, 3 or 4, and it gives you all the same scripts and references as if you were using the official MVC project template. The only difference is that it doesn’t use Nuget to reference all the various referenced libraries (jQuery, jQuery UI, Knockout, Modernizer, etc.) so if you want to keep up to date with the newest releases you have to either download manually, or just download them via Nuget (as needed).
Let’s See Some Code
As I said just right at the beginning, the DXperience library uses ASP.NET MVC HTML Helper extensions methods to dynamically add JavaScript to your pages, so that you don’t have to mix JavaScript code to C# code. Personally I like writing JavaScript code, but I have to admit that sometimes, when you have to pass values coming from the page’s Model to JS constructors or methods, the mix of JS and C# is a bit too much, and I usually end up writing my own Html extension method.
Setting Up the References
Because JavaScript is necessary for the client-side interactions, you need to include the references to the JavaScript scripts and CSS files. To add them all you have to do is use one of the MVC extensions and list all the "MVC Extensions" you are going to add in your application:
@Html.DevExpress().GetStyleSheets(
new StyleSheet { ExtensionSuite = ExtensionSuite.NavigationAndLayout },
new StyleSheet { ExtensionSuite = ExtensionSuite.Editors },
new StyleSheet { ExtensionSuite = ExtensionSuite.HtmlEditor },
new StyleSheet { ExtensionSuite = ExtensionSuite.GridView },
new StyleSheet { ExtensionSuite = ExtensionSuite.PivotGrid },
new StyleSheet { ExtensionSuite = ExtensionSuite.Chart },
new StyleSheet { ExtensionSuite = ExtensionSuite.Report },
new StyleSheet { ExtensionSuite = ExtensionSuite.Scheduler }
)
@Html.DevExpress().GetScripts(
new Script { ExtensionSuite = ExtensionSuite.NavigationAndLayout },
new Script { ExtensionSuite = ExtensionSuite.HtmlEditor },
new Script { ExtensionSuite = ExtensionSuite.GridView },
new Script { ExtensionSuite = ExtensionSuite.PivotGrid },
new Script { ExtensionSuite = ExtensionSuite.Editors },
new Script { ExtensionSuite = ExtensionSuite.Chart },
new Script { ExtensionSuite = ExtensionSuite.Report },
new Script { ExtensionSuite = ExtensionSuite.Scheduler }
)
This generates a long URL that will be used to retrieve a minified and bundled script containing all the needed files:
<link rel="stylesheet" type="text/css"
href="http://www.codeproject.com/DXR.axd?r=[here a 100 char long hash]-PCKT4" />
<script id="dxis_1000344687" src="http://www.codeproject.com/DXR.axd?[here a 500 char long hash]-lDKT4"
type="text/javascript"></script>
<script id="dxss_1816643409" type="text/javascript">
This dynamic delivery is done using the DevExpress custom ASPxHttpHandlerModule
which provides mainly performance and server-side callback processing capabilities.
Adding Components
The library contains lots of interesting components, mostly "enterprise" components and elements, like the omnipresent editable/sortable/filterable grid, various charts, a HTML WYSIWYG editor, the scheduler component (a Outlook calendar-like component), various UI components (dockable panels, split panels, tabs, menus, accordions, trees, modal dialogs). It also has some more general purpose data editors, to beautify and add validations to the usual dropdown lists, textboxes, radio buttons and so on. In the next few paragraphs I’m going to show the general feeling of how it to use the MVC extensions.
For example, to setup a masked input textbox, here is what you would have to do:
@Html.DevExpress().TextBox(
settings => {
settings.Name = "PhoneNum";
settings.Width = 170;
settings.Properties.MaskSettings.Mask = "+00 (999) 000-0000";
settings.Properties.MaskSettings.IncludeLiterals = MaskIncludeLiteralsMode.None;
}
).GetHtml()
So you specify the name of the html field (remember that in ASP.NET MVC the name of the field is what is used by the model binding to get the value for the controller), and then the settings specific for that control (in that case the mask and other mask-specific options).
If you want to pre-fill the value of the textbox with value coming from the view model, all you need to do is "binding" the control to the Model itself.
@Html.DevExpress().TextBox(...).Bind(Model.PhoneNum).GetHtml()
But even easier, is to use the ready-made editor templates and just call the EditorFor and let all the configurations hidden away from your sight. If you use DevExpress project templates you get a few of these editor templates (Boolean, DateTime, Decimal, MultiLineText, String, Password), but if you want you can easily made your own specifying all the settings you need to.
If we wanted, for example, to make a reusable PhoneNum control all we needed would be to just create a Editor template and then specify in the definition of the view model that that particular string is of type PhoneNum.
First create a PhoneNum.cshtml file and place it into the Views\Shared\EditorTemplates folder
@model String
@Html.DevExpress().TextBox(s => {
s.Name = ViewContext.ViewData.TemplateInfo.GetFullHtmlFieldName("");
s.Width = 170;
s.Properties.MaskSettings.Mask = "+00 (999) 000-0000";
s.Properties.MaskSettings.IncludeLiterals = MaskIncludeLiteralsMode.None;
}).Bind(Model).GetHtml()
Than, in the view model class, decorate the property with the UIHint attribute
public class HomeViewModel
{
[UIHint("PhoneNum")]
public String PhoneNum { get; set; }
}
And finally in the view just call the EditorFor method
@model Models.HomeViewModel
<div class="editorContainer">
@Html.EditorFor(m=>m.PhoneNum)
</div>
This way you can easily reuse different editor controls without the need to write always all the configurations. That one was an easy example, but the same concepts and ideas can be applied to all the other MVC extensions.
Conclusions
The whole set of components and the overall experience is really well thought, starting from the first approach to the new project templates. Also the documentation available is really good at explaining all the various options and customization options.
From the technical standpoint the code has a bit too much a WebForms taste, but I have to agree that this approach might have its benefits: it will easily appeal to a WebForm developer, and also a "native" MVC developer, with a bit of work, could write the editor templates he needs, and then live a happy life without the need of writing all these lines of code each time he needs a control. And these native DevExpress MVC extensions are also feature-rich and provide some extensions not found in other libraries like the PivotGrid and Docking Suite.
If that complexity seems too much for the simpler controls, with the most complex ones (like the Scheduler or the Pivot Grid) the "verbosity" almost disappears compared to the amount of code you would need to write in order to implement the same functionalities directly in JavaScript. And the Scheduler component is really awesome: now I want to build something that needs that kind of UI just because I want to use it.
Disclosure of Material Connection: I received one or more of the products or services mentioned above for free in the hope that I would mention it on my blog. Regardless, I only recommend products or services I use personally and believe my readers will enjoy. I am disclosing this in accordance with the Federal Trade Commission’s 16 CFR, Part 255: "Guides Concerning the Use of Endorsements and Testimonials in Advertising."
Related Links
My name is Simone Chiaretta and I'm a software developer/architect his coding on the .NET platform both for business and for fun since 2001, when .NET was still in Beta version.
I worked for 8 years for Esperia, a web agency based in Milan (Italy), and then I decided to go the place which is the farthest from Milan: Wellington in New Zealand where I worked as Chief Software Architect for Calcium Software Ltd.
Back to Italy I decided to try the consulting world, and joined the ranks of Avanade, an international consulting company jointly owned by Accenture and Microsoft, where I'm now working as Senior Solution Developer.
But fed up with it I decided to move out of Italy, again, and now I'm technical lead and "web architect" (whatever that might mean) at the Council of European Union, the main decision making body of the European Union.
I worked on many opensource projects in the past, but now I'm mainly focusing on Subtext.
I'm an active community member, in Italy and worldwide: my other blog about .NET is in Italian and hosted on the UGIdotNET web site. I also I had a few talks at various usergroups and I wrote some technical articles both for online and paper magazines.
And when I'm not writing code, blog posts or taking part in the worldwide .NET community, I like to look for the shortest path up to a mountain, which usually is a vertical one: free-climbing, mountain climbing and ice climbing are my other way of throwing away my spare time.