Adding a Status Bar Pane to an Internet Explorer Window and Unmasking the Web Page Passwords in Place






4.63/5 (12 votes)
This article demonstrates how to add a Status Bar pane to Internet Explorer Window, and then how to use it for managing Web page password fields.

Introduction
While surfing the Web, Internet Explorer status bar indicators can be very useful and allow access to several built-in applications such as Popup blocker, Phishing Filter, or Security applet. Unlike IE Toolbar buttons, Microsoft has said nothing to developers about how to add a status bar pane to an Internet Explorer Window.
This article shows how to add a status bar pane using subclassing tricks.
First, find and subclass Status Bar window and create a pane window (as a STATIC control). Next, choose any existing pane, resize it for inserting a new one, and place the pane window over the existing pane where desired.
A new status pane should be drawn and themed (for XP or Vista) exactly as any typical pane, and mouse clicks should be handled to prevent notifications from the native pane behind.
General Steps
- Create a Browser Helper Object that is started from within Internet Explorer:
extern "C" BOOL WINAPI DllMain(HINSTANCE hInstance, DWORD dwReason, LPVOID /*lpReserved*/) { if (dwReason == DLL_PROCESS_ATTACH) { TCHAR szLoader[MAX_PATH]; memset(szLoader,0,MAX_PATH); GetModuleFileName(NULL, szLoader, MAX_PATH); _tcslwr(szLoader); if (_tcsstr(szLoader,_T("iexplore.exe")) == 0 && _tcsstr(szLoader,_T("regsvr32.exe")) == 0) return FALSE;
- Find the Status bar window and subclass it:
LONG nBrowser = NULL; m_pWebBrowser2->get_HWND(&nBrowser); if(nBrowser) { g_hIcon = LoadIcon(_Module.m_hInst, MAKEINTRESOURCE(IDI_ICON_START)); TCHAR szClassName[MAX_PATH]; HWND hWndStatusBar = NULL; // looking for a TabWindowClass window in IE7 // the last one should be parent for statusbar HWND hTabWnd = GetWindow((HWND)nBrowser, GW_CHILD); if(hTabWnd) { while(hTabWnd) { memset(szClassName,0,MAX_PATH); GetClassName(hTabWnd, szClassName, MAX_PATH); if(_tcscmp(szClassName, _T("TabWindowClass")) == 0) nBrowser = (LONG)hTabWnd; hTabWnd = GetWindow(hTabWnd, GW_HWNDNEXT); } } HWND hWnd = GetWindow((HWND)nBrowser, GW_CHILD); if(hWnd) { while(hWnd) { memset(szClassName,0,MAX_PATH); GetClassName(hWnd, szClassName, MAX_PATH); if(_tcscmp(szClassName,_T("msctls_statusbar32")) == 0) { if(hWnd) hWndStatusBar = hWnd; break; } hWnd = GetWindow(hWnd, GW_HWNDNEXT); } } if(hWndStatusBar) { g_pWndProcStatus = (WNDPROC)SetWindowLong(hWndStatusBar, GWL_WNDPROC, (LPARAM)(WNDPROC)NewStatusProc); ...
- Create a pane window as a STATIC control and subclass it:
HWND hWndNewPane = CreateWindowEx(NULL, _T("static"),_T(""),WS_CHILD | WS_VISIBLE,0,0,0,0,hWndStatusBar,(HMENU)3333,_Module.m_hInst,NULL); if(hWndNewPane && IsWindow(hWndNewPane)) { HFONT hFont = (HFONT)SendMessage(hWndStatusBar, WM_GETFONT, 0, 0); SendMessage(hWndNewPane, WM_SETFONT, (WPARAM)hFont, MAKELPARAM(TRUE, 0)); GetUxtheme(hWndNewPane); g_pWndProcPane = (WNDPROC)SetWindowLong(hWndNewPane, GWL_WNDPROC, (LPARAM)(WNDPROC)PaneWindowProc); ...
- Process important messages of status bar:
static LRESULT CALLBACK NewStatusProc (HWND hWnd, UINT message, WPARAM wParam, LPARAM lParam) { ... switch (message) { case SB_SIMPLE: // hide the pane when the user scrolls the menu ::ShowWindow(g_hWndNewPane, !(BOOL)wParam); break; case WM_SYSCOLORCHANGE: // query updated XP theme GetUxtheme(g_hWndNewPane); break; ... case SB_SETPARTS: // resize the existing pane and move the new pane over { if(lParam && wParam) { unsigned int nParts = wParam; HLOCAL hLocal = LocalAlloc(LHND, sizeof(int) * (nParts+1)); LPINT lpParts = (LPINT)LocalLock(hLocal); memcpy(lpParts, (void*)lParam, wParam*sizeof(int)); g_nNewPaneWidth = 32; unsigned nCurWidth = 0; for(unsigned j=0;j<nParts-1;j++) { nCurWidth = lpParts[j+1]-lpParts[j]; if(g_nNewPaneWidth > nCurWidth && nCurWidth>1) g_nNewPaneWidth = nCurWidth; } g_nNewPaneWidth += 2; if(g_nNewPanePosition<nParts) { for(unsigned i=0;i<g_nNewPanePosition;i++) lpParts[i] -= g_nNewPaneWidth; } LRESULT hRet = CallWindowProc(g_pWndProcStatus, hWnd, message, wParam, (LPARAM)lpParts); CRect rcPane; SendMessage(hWnd, SB_GETRECT, g_nNewPanePosition, (LPARAM)&rcPane); if(IsWindow(g_hWndNewPane)) MoveWindow(g_hWndNewPane, lpParts[g_nNewPanePosition] - g_nNewPaneWidth, rcPane.top, g_nNewPaneWidth, rcPane.Height(), TRUE); LocalFree(hLocal); return hRet; } } break; default: break; }
- Process
WM_PAINT
message for a new pane:
static LRESULT CALLBACK PaneWindowProc(HWND hWnd, UINT message, WPARAM wParam, LPARAM lParam) { switch (message) { case WM_PAINT: { PAINTSTRUCT ps; HDC hDC = BeginPaint(hWnd, &ps); CRect rcClient; GetClientRect(hWnd, &rcClient); int nDrawEdge = 0; // not themed if(g_hTheme == NULL) { FillRect(hDC, &rcClient, (HBRUSH)(COLOR_BTNFACE+1)); DrawEdge(hDC,&rcClient,BDR_RAISEDINNER,BF_LEFT); rcClient.left += 3; nDrawEdge = 3; DrawEdge(hDC,&rcClient,BDR_SUNKENOUTER,BF_RECT); } else { DRAWTHEMEBACKGROUND pfnDrawThemeBackground = (DRAWTHEMEBACKGROUND)::GetProcAddress (g_hUxtheme, "DrawThemeBackground"); if(pfnDrawThemeBackground) pfnDrawThemeBackground(g_hTheme, hDC, SP_PANE, NULL, &rcClient, NULL); // copy separator picture from right to left int nHeight = rcClient.Height(); int nWidth = rcClient.Width() - 2; for(int i=0;i<2;i++) { for(int j=0;j<nHeight;j++) SetPixel(hDC,i,j,GetPixel(hDC,i+nWidth,j)); } } if(g_hIcon) DrawIconEx(hDC,(rcClient.Width() - 16)/2 + nDrawEdge, (rcClient.Height() - 16)/2, g_hIcon,16,16,NULL,NULL,DI_NORMAL); EndPaint(hWnd, &ps); return 0; } ...
By default, the Internet Explorer Status bar contains several panes. The first pane (left) is a separator and can display menu prompts and visited Web page notifications. The second pane is normally invisible and contains a progress control. When the Web navigation starts, this pane becomes visible and can show download progress. The next panes can be occupied by built-in tools of Internet Explorer, such as Popup Blocker or Phishing Filter.
For example, I decide to place a new pane at the third position and reserve enough space to display a small icon:
UINT g_nNewPaneWidth = 20;
UINT g_nNewPanePosition = 2;
So, now the pane is complete and ready for use.
In the sample code attached to this article, there is a newly created pane used for access to the password revealing application named Asterisk Master and published on my web site.
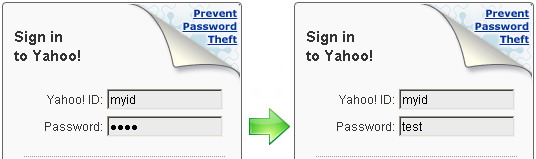
When Internet Explorer has finished opening any web page, Asterisk Master starts searching for a password input box and substitutes it with a usual text input box, so any hidden characters become readable.
The password input box can be defined in HTML by type=password
attribute.
<INPUT id=inputPassword type=password value="" name=passwd>
If the attribute type=password
is substituted with type=text
on the fly, all the asterisk masked characters become readable.
So, subscribe to IWebBrowser2
events and process the visited web page when DISPID_DOWNLOADCOMPLETE
is fired:
STDMETHODIMP CFieldManager::Invoke(DISPID dispidMember, REFIID riid, LCID lcid,
WORD wFlags, DISPPARAMS* pDispParams, VARIANT* pvarResult,
EXCEPINFO* pExcepInfo, UINT* puArgErr)
{
if (!pDispParams)
return E_INVALIDARG;
switch (dispidMember)
{
case DISPID_DOWNLOADBEGIN:
g_hIcon = LoadIcon(_Module.m_hInst, MAKEINTRESOURCE(IDI_ICON_START));
RedrawWindow(g_hWndNewPane,NULL,NULL,RDW_INVALIDATE|RDW_UPDATENOW);
break;
case DISPID_DOWNLOADCOMPLETE:
m_bPasswordsFound = FALSE;
ShowPasswords();
if(m_bPasswordsFound)
{
if(g_bUnmaskNeeded)
g_hIcon = LoadIcon(_Module.m_hInst, MAKEINTRESOURCE(IDI_ICON_UNLOCK));
else
g_hIcon = LoadIcon(_Module.m_hInst, MAKEINTRESOURCE(IDI_ICON_LOCK));
}
else
g_hIcon = LoadIcon(_Module.m_hInst, MAKEINTRESOURCE(IDI_ICON_START));
RedrawWindow(g_hWndNewPane,NULL,NULL,RDW_INVALIDATE|RDW_UPDATENOW);
break;
default:
break;
}
Next, the ShowPassword()
function scans through the web page for password fields and substitutes type
attributes if needed.
Please feel free to post errors, issues, or requests.