aCloud WebOS






3.60/5 (7 votes)
aCloud Web Operation System running on the cloud
Introduction
aCloud is a WebOS that provides fast and efficient Web Desktop Environment. It stands for Arabian Cloud, that mean it's especially for Arabian people.
Background
aCloud is the first Arabic WebOS on the cloud, it's based on Silverlight 4 and it's the second cloud system that uses that technology after SilveOS.
WebOS or WebTop is a virtual operating system that runs in your web browser. aCloud is a simple WebOS that run on the cloud. Through aCloud, anyone can edit their documents, compose and send mails, use calendar, address book, etc. WebOS is not an Operating System, it is an application. Using the browser, anyone can use this essential daily application over the internet.
The WebOS is classified into many categories:
- Self-Hosted WebOS like eyeOS
- Remote Desktops like Widgeop
- Remotely Hosted WebOS like ghost WebOS
Cloud Computing Technology
Cloud computing is an Internet-based computing, whereby shared resources, software and information are provided to computers and other devices on-demand like the electricity grid.
The cloud is a group of servers. A user interacts with the cloud without worrying about how it is implemented.

There are three types of the cloud:
- Infrastructure as a Service (IaaS)
- Platform as a Service (PaaS)
- Software as a Service (SaaS)
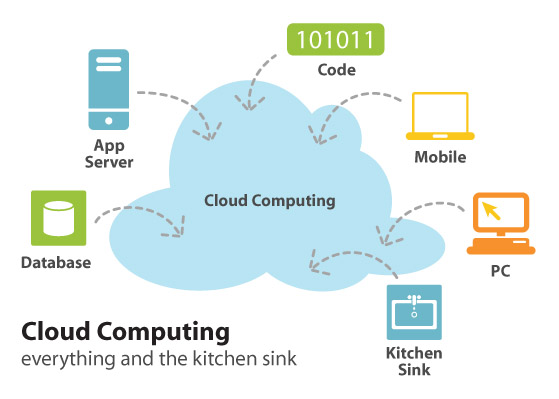
Layers of Cloud Computing:

Code Architecture
aCloud is based on a 3-tier architecture:
- Presentation Layer: It consists of Silverlight User Controls that made up the GUI for the whole Web Desktop.
- Business Layer: It's a simple Web Service which has a bunch of code that made the core functionality of the system.
- Data Layer: It' a simple XML file, which has information about current aClouders.
Using the Code
This is a sample user control in the presentation layer of aCloud named Card
which contains personal information about the current clouder.
Card.Xaml
<UserControl x:Class="aCloud.Card"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
d:DesignHeight="94" d:DesignWidth="226" FontSize="13">
<Grid x:Name="LayoutRoot">
<Border Height="75" Cursor="Hand" HorizontalAlignment="Left" Margin="9,10,0,0"
Name="PictureBorder" VerticalAlignment="Top" Width="68" CornerRadius="10">
<Border.Background>
<ImageBrush ImageSource="/aCloud;component/Images/Profile.png" />
</Border.Background>
</Border>
<TextBlock Height="21" HorizontalAlignment="Left" Margin="81,10,0,0"
Name="UsernameTextBlock" Text="إسم المستخدم" VerticalAlignment="Top"
Width="136" TextTrimming="WordEllipsis" TextAlignment="Right"
FontWeight="Bold" Foreground="WhiteSmoke">
<TextBlock.Effect>
<DropShadowEffect />
</TextBlock.Effect>
</TextBlock>
<TextBlock Height="21" HorizontalAlignment="Left" Margin="81,0,0,32"
Name="LastLoginDateTextBlock" Text="آخر دخول: 00/00/0000"
VerticalAlignment="Bottom" TextAlignment="Right" Foreground="WhiteSmoke" Width="136">
<TextBlock.Effect>
<DropShadowEffect />
</TextBlock.Effect>
</TextBlock>
<TextBlock HorizontalAlignment="Right" Margin="0,64,0,9"
Name="LoginsNoTextBlock" Text="عدد مرات الدخول: 00000" TextAlignment="Right"
Foreground="WhiteSmoke" Width="136">
<TextBlock.Effect>
<DropShadowEffect />
</TextBlock.Effect>
</TextBlock>
</Grid>
</UserControl>
Card.xaml.vb
Imports System.Windows.Media.Imaging
Imports System.IO
Imports aCloud.aCloudService
Partial Public Class Card
Inherits UserControl
Dim MyService As New aCloudServiceSoapClient()
Public Sub New()
InitializeComponent()
End Sub
Public Property Picture As String
Get
Return Nothing
End Get
Set(ByVal value As String)
Dim brush As New ImageBrush()
brush.ImageSource = New BitmapImage(New Uri(value))
PictureBorder.Background = brush
End Set
End Property
Public Property Username As String
Get
Return UsernameTextBlock.Text
End Get
Set(ByVal value As String)
UsernameTextBlock.Text = value
End Set
End Property
Public Property LastLoginDate As String
Get
Return LastLoginDateTextBlock.Text
End Get
Set(ByVal value As String)
LastLoginDateTextBlock.Text = String.Format("آخر دخول: {0}", value)
End Set
End Property
Public Property LoginsNo As String
Get
Return LoginsNoTextBlock.Text
End Get
Set(ByVal value As String)
LoginsNoTextBlock.Text = String.Format("عدد مرات الدخول: {0}", value)
End Set
End Property
Private Sub PictureBorder_MouseLeftButtonUp(ByVal sender As Object, _
ByVal e As System.Windows.Input.MouseButtonEventArgs) _
Handles PictureBorder.MouseLeftButtonUp
Dim ofd As New OpenFileDialog()
ofd.Filter = "Image Files |*.jpg;*.png;*.bmp;*.gif"
ofd.Multiselect = False
If ofd.ShowDialog() Then
Dim bi As New BitmapImage()
Dim data(ofd.File.Length) As Byte
ofd.File.OpenRead().Read(data, 0, data.Length)
MyService.UploadAsync(String.Format("aCloud Users/{0}/Picture.jpg", User.ID), data)
bi.SetSource(ofd.File.OpenRead())
Dim img As New ImageBrush()
img.ImageSource = bi
PictureBorder.Background = img
End If
End Sub
End Class
There're many functions in business layer that provide the basic functionality of aCloud. I'll mention some of them.
<WebMethod()> _
Public Function GetDirectories(ByVal FullName As String) As List(Of String)
Dim contents As New List(Of String)
Dim path As String = Server.MapPath(FullName)
For Each directory In New DirectoryInfo(path).GetDirectories()
contents.Add(<Directory ID=<%= directory.FullName.Substring_
(directory.FullName.IndexOf("aCloud Users")) %>>
<Name><%= directory.Name %></Name>
<Items><%= Convert.ToBoolean(directory.GetDirectories().Count + _
directory.GetFiles().Count) %></Items>
</Directory>.ToString())
Next
Return contents
End Function
<WebMethod()> _
Public Sub DeleteFile(ByVal FullName As String)
Dim path As String = Server.MapPath(FullName)
File.Delete(path)
End Sub
<WebMethod()> _
Public Sub CreateDirectory(ByVal FullName As String)
Dim path As String = Server.MapPath(FullName)
Directory.CreateDirectory(path)
End Sub
<WebMethod()> _
Public Sub Upload(ByVal FullName As String, ByVal data As Byte())
File.WriteAllBytes(HttpContext.Current.Server.MapPath(FullName), data)
End Sub
The essential part in the data layer is an XML file that contains information about clouders users.
<?xml version="1.0" encoding="utf-8"?>
<Users>
<User ID="6451be9f-8a63-409d-87c9-af6c14305fe3">
<Name>admin</Name>
<Password>123</Password>
<CreationDate>4/3/2011</CreationDate>
<LastLoginDate>4/3/2011</LastLoginDate>
<LoginsNo>1</LoginsNo>
<Status>Offline</Status>
</User>
<User ID="86612741-dd32-4d3a-bf16-d8fad6c3f2d0">
<Name>demo</Name>
<Password>demo</Password>
<CreationDate>4/3/2011</CreationDate>
<LastLoginDate>4/16/2011</LastLoginDate>
<LoginsNo>235</LoginsNo>
<Status>Offline</Status>
</User>
<User ID="83c5dff7-00dd-47b3-a63b-750677421220">
<Name>test</Name>
<Password>test</Password>
<CreationDate>4/16/2011</CreationDate>
<LastLoginDate>4/25/2011</LastLoginDate>
<LoginsNo>12</LoginsNo>
<Status>Offline</Status>
</User>
</Users>
(Screenshots from aCloud)
Points of Interest
- Silverlight is one of the RIA that makes the web applications look like a desktop applications
- Cloud Computing make is the future of operating system.
History
aCloud WebOS 1.0 beta version, which includes the basic functionalities for file management.
- Try it online: aCloud
- Install it Out of Browser, you can either register or login with
Username: admin, Password: 123