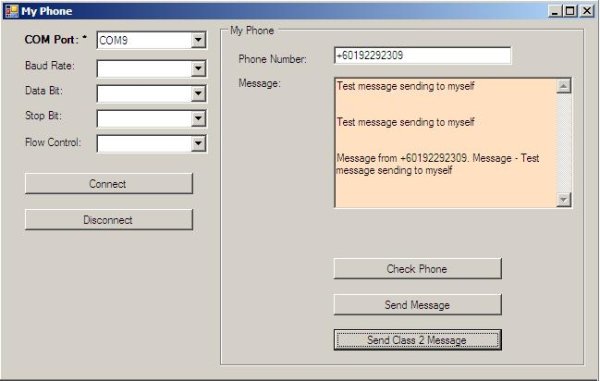
Introduction
In part II of the article, I showed you how to send SMS using a GSM modem which is built into your mobile phone. However, I did not show you how to detect incoming SMS. To detect new SMS received, it is a bit tricky as different mobile phones from different manufacturers may need different configurations. In this article, I am going to show you how to detect new SMS using the open source atSMS library.
How It Works
In order to detect incoming SMS, you must use the "AT+CNMI" command to set the new message indication to the terminal equipment (TE).
For the "+CNMI" command, the values are in the format of mode,mt,bm,ds,bfr
. In order to receive SMS, mt
must be 1. For other values, different handsets may require different values to be set. By default, the atSMS library sets mode
and mt
to 1 which should be sufficient for most handsets. However, you can always use the CheckATCommands
function to check your phone supported values.
Take note also that during my testing, it was noticed that for certain Nokia phones only, Class 2 SMS can be detected. For newer models of Nokia phones, e.g., N70, N80, the "+CNMI" is not supported, and you have no way of detecting incoming SMS.
The Code
By setting NewMessageIndication
to True
, and if your phone supports "+CNMI", the NewMessageReceived
event should be raised.
Imports ATSMS
Public Class MainForm
Private WithEvents oGsmModem As New GSMModem
Private Sub MainForm_Load(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles MyBase.Load
CheckForIllegalCrossThreadCalls = False
End Sub
Private Sub btnPhone_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles btnConnect.Click
If cboComPort.Text = String.Empty Then
MsgBox("COM Port must be selected", MsgBoxStyle.Information)
Return
End If
oGsmModem.Port = cboComPort.Text
If cboBaudRate.Text <> String.Empty Then
oGsmModem.BaudRate = Convert.ToInt32(cboBaudRate.Text)
End If
If cboDataBit.Text <> String.Empty Then
oGsmModem.DataBits = Convert.ToInt32(cboDataBit.Text)
End If
If cboStopBit.Text <> String.Empty Then
Select Case cboStopBit.Text
Case "1"
oGsmModem.StopBits = Common.EnumStopBits.One
Case "1.5"
oGsmModem.StopBits = Common.EnumStopBits.OnePointFive
Case "2"
oGsmModem.StopBits = Common.EnumStopBits.Two
End Select
End If
If cboFlowControl.Text <> String.Empty Then
Select Case cboFlowControl.Text
Case "None"
oGsmModem.FlowControl = Common.EnumFlowControl.None
Case "Hardware"
oGsmModem.FlowControl = Common.EnumFlowControl.RTS_CTS
Case "Xon/Xoff"
oGsmModem.FlowControl = Common.EnumFlowControl.Xon_Xoff
End Select
End If
Try
oGsmModem.Connect()
Catch ex As Exception
MsgBox(ex.Message, MsgBoxStyle.Critical)
Return
End Try
Try
oGsmModem.NewMessageIndication = True
Catch ex As Exception
End Try
btnSendMsg.Enabled = True
btnSendClass2Msg.Enabled = True
btnCheckPhone.Enabled = True
btnDisconnect.Enabled = True
MsgBox("Connected to phone successfully !", MsgBoxStyle.Information)
End Sub
Private Sub btnDisconnect_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles btnDisconnect.Click
Try
oGsmModem.Disconnect()
Catch ex As Exception
MsgBox(ex.Message, MsgBoxStyle.Critical)
End Try
btnSendMsg.Enabled = False
btnSendClass2Msg.Enabled = False
btnCheckPhone.Enabled = False
btnDisconnect.Enabled = False
btnConnect.Enabled = True
End Sub
Private Sub btnSendMsg_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles btnSendMsg.Click
If txtPhoneNumber.Text.Trim = String.Empty Then
MsgBox("Phone number must not be empty", MsgBoxStyle.Critical)
Return
End If
If txtMsg.Text.Trim = String.Empty Then
MsgBox("Phone number must not be empty", MsgBoxStyle.Critical)
Return
End If
Try
Dim msg As String = txtMsg.Text.Trim
Dim msgNo As String
If StringUtils.IsUnicode(msg) Then
msgNo = oGsmModem.SendSMS(txtPhoneNumber.Text, msg, _
Common.EnumEncoding.Unicode_16Bit)
Else
msgNo = oGsmModem.SendSMS(txtPhoneNumber.Text, msg, _
Common.EnumEncoding.GSM_Default_7Bit)
End If
MsgBox("Message is sent. Reference no is " & msgNo, _
MsgBoxStyle.Information)
Catch ex As Exception
MsgBox(ex.Message & ". Make sure your SIM memory" & _
" is not full.", MsgBoxStyle.Critical)
End Try
End Sub
Private Sub btnSendClass2Msg_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles btnSendClass2Msg.Click
If txtPhoneNumber.Text.Trim = String.Empty Then
MsgBox("Phone number must not be empty", MsgBoxStyle.Critical)
Return
End If
If txtMsg.Text.Trim = String.Empty Then
MsgBox("Phone number must not be empty", MsgBoxStyle.Critical)
Return
End If
Try
Dim msg As String = txtMsg.Text.Trim
Dim msgNo As String
If StringUtils.IsUnicode(msg) Then
msgNo = oGsmModem.SendSMS(txtPhoneNumber.Text, msg, _
Common.EnumEncoding.Unicode_16Bit)
Else
msgNo = oGsmModem.SendSMS(txtPhoneNumber.Text, msg, _
Common.EnumEncoding.Class2_7_Bit)
End If
MsgBox("Message is sent. Reference no is " & msgNo, _
MsgBoxStyle.Information)
Catch ex As Exception
MsgBox(ex.Message & ". Make sure your SIM memory is not full.", _
MsgBoxStyle.Critical)
End Try
End Sub
Private Sub oGsmModem_NewMessageReceived(ByVal e As _
ATSMS.NewMessageReceivedEventArgs) Handles _
oGsmModem.NewMessageReceived
txtMsg.Text = "Message from " & e.MSISDN & ". Message - " & _
e.TextMessage & ControlChars.CrLf
End Sub
Private Sub btnCheckPhone_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles btnCheckPhone.Click
MsgBox("Going to analyze your phone. It may take a while", _
MsgBoxStyle.Information)
oGsmModem.CheckATCommands()
If oGsmModem.ATCommandHandler.Is_SMS_Received_Supported Then
MsgBox("Your phone is able to receive SMS. Message " & _
"indication command is " & _
oGsmModem.ATCommandHandler.MsgIndication, _
MsgBoxStyle.Information)
oGsmModem.NewMessageIndication = True
Else
MsgBox("Sorry. Your phone cannot receive SMS", _
MsgBoxStyle.Information)
End If
End Sub
End Class
In the next article, I will show you how to send vCard, vCalendar, and WAP Push messages using the atSMS library.
A programmer for a long time, and still learning everyday.
A supporter for open source solutions, and have written quite a few open source software both in .NET and Java.
https://mengwangk.github.io/