This article shows how to use PDFRasterizer.NET 4.0 to render a PDF page to a bitmap and to an Android canvas. PDFRasterizer.NET 4.0 supports various frameworks including Xamarin.Android. This article was written when PDFRasterizer.NET 4.0 was still in beta. You can view the latest changelog here.
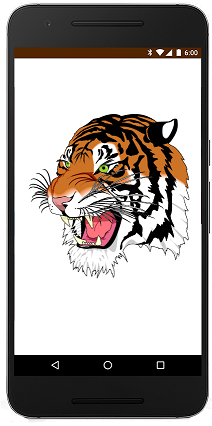
Render PDF page to PNG
The code discussed here draws a PDF page in a Xamarin.Android app by converting a PDF page to a bitmap and assigning that bitmap to an ImageView. To keep the code sample simple, the PDF document itself is embedded as a resource. In a more realistic code sample it would be selected from the device or from cloud storage.
We will show the relevant code snippets. You can download the full source code from github.
Layout
The main layout of the application only has an ImageView
and looks as follows:
="1.0"="utf-8"
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<ImageView
android:id="@+id/imageView"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
</LinearLayout>
Activity.OnCreateLayout
The main activity overrides the OnCreate
and performs the following tasks:
- create a PDF Page object from "tiger.pdf" that is embedded as a resource
- render the PDF Page object to a Bitmap
- assign the Bitmap to the
ImageView
that is part of the main layout
It looks as follows:
protected async override void OnCreate(Bundle savedInstanceState)
{
base.OnCreate(savedInstanceState);
SetContentView(Resource.Layout.Main);
var assembly = Assembly.GetExecutingAssembly();
var inputStream = new MemoryStream();
using (Stream resourceStream = assembly.GetManifestResourceStream("DrawPdf.Android.tiger.pdf"))
{
resourceStream.CopyTo(inputStream);
}
using (var outputStream = new MemoryStream())
{
await Task.Run(() =>
{
Document document = new Document(inputStream);
Page page = document.Pages[0];
page.SaveAsBitmap(outputStream, CompressFormat.Png, 72);
});
Bitmap bmp = global::Android.Graphics.BitmapFactory.DecodeByteArray(outputStream.GetBuffer(), 0, (int) outputStream.Length);
ImageView imageView = FindViewById<ImageView>(Resource.Id.imageView);
imageView.SetImageBitmap(bmp);
}
}
Render PDF to Android.Canvas
The code discussed here implements a custom View (PdfPageView) and draws a PDF page directly to an Android Canvas.
We will show the relevant code snippets. You can download the full source code from github.
Activity.OnCreate
Inside OnCreate
of the main activity, a Page instance is contructed and passed to the PdfPageView
ctor. The custom view is set as the content view:
protected override void OnCreate(Bundle savedInstanceState)
{
base.OnCreate(savedInstanceState);
var assembly = Assembly.GetExecutingAssembly();
var inputStream = new MemoryStream();
using (Stream resourceStream = assembly.GetManifestResourceStream("DrawPdf.Android.tiger.pdf"))
{
resourceStream.CopyTo(inputStream);
}
Document document = new Document(inputStream);
Page page = document.Pages[0];
SetContentView(new PdfPageView(this, page));
}
PdfPageView
The custom View uses the Page.Draw
method to draw directly to the canvas. It looks like this:
public class PdfPageView : View
{
Page _page;
public PdfPageView(Context context, Page page) :
base(context)
{
_page = page;
}
protected override void OnDraw(Canvas canvas)
{
_page.Draw(canvas, 1);
}
}
Worked for some years as a software engineer, architect and project leader for different software companies. Works at TallComponents, vendor of class libraries for creating, manipulating and rendering PDF documents.