Revealed: How to Create Universal Dialog Handlers Testing Framework





5.00/5 (1 vote)
Create tests that handle different dialogs via Testing Framework. Provide universal utilities, so that you do not need to write boilerplate code.
Introduction
Not all tests play out directly inside a browser. Web pages can display popup dialog windows such as alerts, confirmations and others. Testing Framework allows you to track and respond to dialog windows and can handle HTML popups and Win32 dialogs. It is not a difficult task to write code that handles dialogs. However, I will show you how you can create universal dialog handlers so that you do not need to write boilerplate code.
Download File Dialog
The first case that we are going to cover is the one for downloading files.
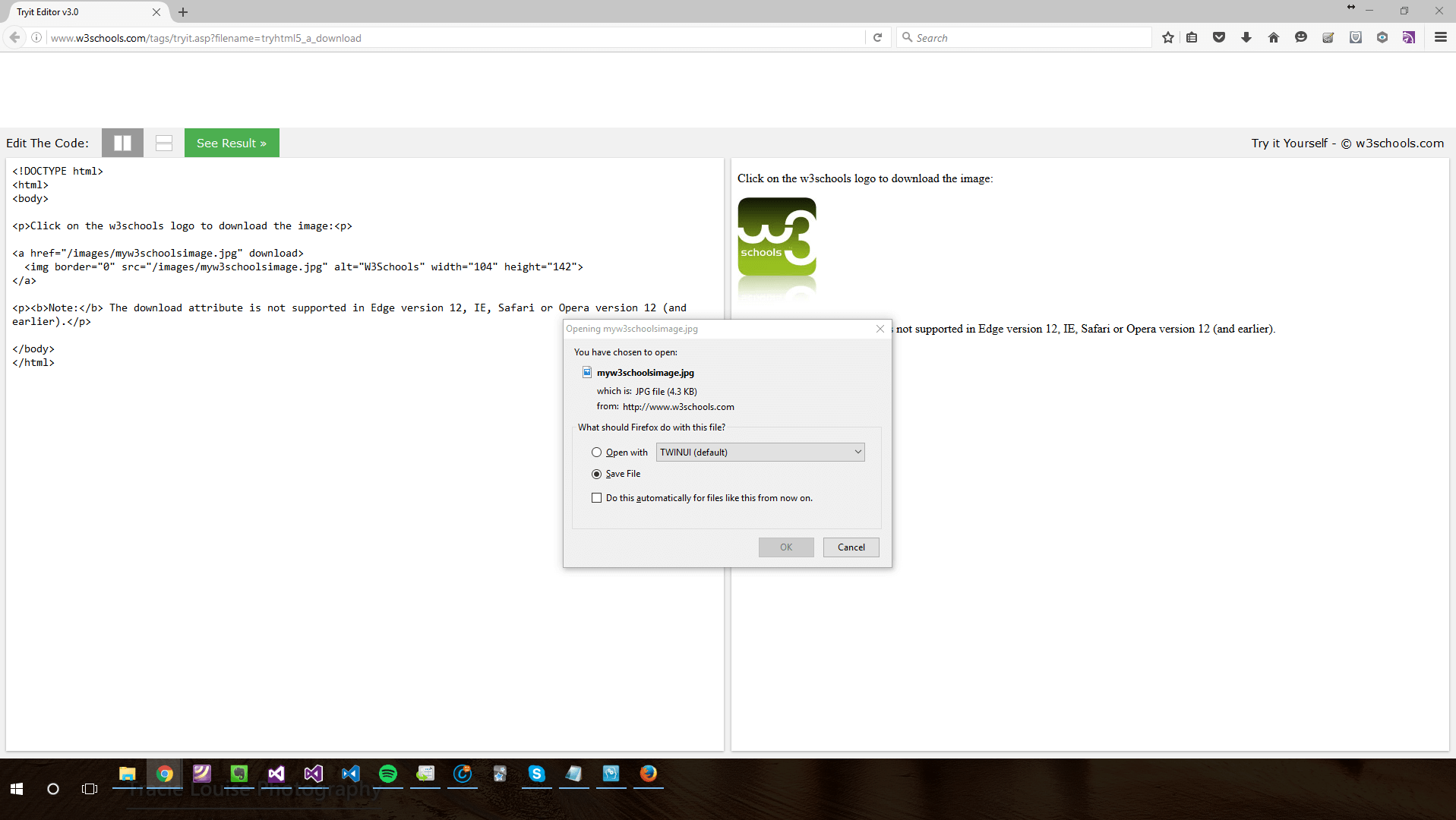
If you are just getting started with Testing Framework I recommend you to read my post- Getting Started with Telerik Testing Framework C# in 10 Minutes. Also, you can download the framework from the official site.
Download Dialog Handler Testing Framework
You should first create DownloadDialogsHandler with the appropriate parameters. After that, you start a dialog monitor and navigate to the desired page. After you click on the download button, the dialog is automatically handled by Testing Framework engine.
[TestMethod]
public void DownloadDialogNotUniversal()
{
string fileToDownload =
Path.Combine(
AppDomain.CurrentDomain.BaseDirectory,
@"myw3schoolsimage.jpg");
if (File.Exists(fileToDownload))
{
File.Delete(fileToDownload);
}
var dialog = new DownloadDialogsHandler(
manager.ActiveBrowser,
DialogButton.SAVE,
fileToDownload,
manager.Desktop);
manager.DialogMonitor.Start();
manager.ActiveBrowser.NavigateTo(
"http://www.w3schools.com/tags/tryit.asp?filename=tryhtml5_a_download");
ArtOfTest.WebAii.Core.Browser myFrame =
manager.ActiveBrowser.Frames.ById("iframeResult");
HtmlImage image =
myFrame.Find.AllByTagName<HtmlImage>("img")[0];
image.Click(false);
dialog.WaitUntilHandled();
}
If the file exists on the disk, the test deletes it. Through AppDomain.CurrentDomain.BaseDirectory we access the folder of the tests' assemblies.
Universal Download Dialog Handler
We can create a static class that hides the complexity of the Testing Framework dialog handler.
public static class DownloadDialogHandler
{
public static void DownloadFile(
Action action,
string saveLocation)
{
Manager.Current.DialogMonitor.Start();
Browser browser = Manager.Current.ActiveBrowser;
DownloadDialogsHandler handler =
new DownloadDialogsHandler(
browser,
DialogButton.SAVE,
saveLocation,
browser.Desktop);
action();
handler.WaitUntilHandled();
}
}
In general, the code does the same. We need to pass the clicking of the download button as an anonymous action.
[TestMethod]
public void DownloadDialogUniversal()
{
string fileToDownload =
Path.Combine(
AppDomain.CurrentDomain.BaseDirectory,
@"myw3schoolsimage.jpg");
if (File.Exists(fileToDownload))
{
File.Delete(fileToDownload);
}
manager.ActiveBrowser.NavigateTo(
"http://www.w3schools.com/tags/tryit.asp?filename=tryhtml5_a_download");
DownloadDialogHandler.DownloadFile(
() =>
{
Browser myFrame = manager.ActiveBrowser.Frames.ById("iframeResult");
HtmlImage image = myFrame.Find.AllByTagName<HtmlImage>("img")[0];
image.Click(false);
},
AppDomain.CurrentDomain.BaseDirectory);
}
Upload File Dialog
The next thing that we want to be able to do is to upload files.

Upload File Dialog Handler Testing Framework
The code is almost identical to the previous one except this time we create FileUploadDialog and pass different parameters.
[TestMethod]
public void UploadFileDialogNotUniversal()
{
string fileToBeUploadedPath =
Path.Combine(
AppDomain.CurrentDomain.BaseDirectory,
@"myw3schoolsimage.jpg");
var fileDialog = new FileUploadDialog(
manager.ActiveBrowser,
fileToBeUploadedPath,
DialogButton.OPEN);
manager.DialogMonitor.AddDialog(fileDialog);
manager.DialogMonitor.Start();
manager.ActiveBrowser.NavigateTo(
"http://nervgh.github.io/pages/angular-file-upload/examples/simple/");
HtmlInputFile fileInput =
manager.ActiveBrowser.Find.ByXPath<HtmlInputFile>(
"//*[@id='ng-app']/body/div/div[2]/div[1]/input[2]");
fileInput.Click(false);
}
Universal File Upload Dialog Handler
Again we create a static class that hides the file dialog handler code's complexity.
public static class FileUploader
{
public static void Upload(Action action, string filePath)
{
Manager.Current.ActiveBrowser.Window.Maximize();
var dialog = new FileUploadDialog(
Manager.Current.ActiveBrowser,
filePath,
DialogButton.OPEN,
"OPEN");
try
{
Manager.Current.DialogMonitor.AddDialog(dialog);
Manager.Current.ActiveBrowser.RefreshDomTree();
action();
dialog.WaitUntilHandled();
}
finally
{
Manager.Current.DialogMonitor.RemoveDialog(dialog);
}
}
}
The usage in tests is straightforward.
[TestMethod]
public void UploadFileDialogUniversal()
{
string fileToBeUploadedPath =
Path.Combine(
AppDomain.CurrentDomain.BaseDirectory,
@"myw3schoolsimage.jpg");
manager.ActiveBrowser.NavigateTo(
"http://nervgh.github.io/pages/angular-file-upload/examples/simple/");
FileUploader.Upload(
() =>
{
HtmlInputFile fileInput =
manager.ActiveBrowser.Find.ByXPath<HtmlInputFile>(
"//*[@id='ng-app']/body/div/div[2]/div[1]/input[2]");
fileInput.Click(false);
},
fileToBeUploadedPath);
}
Confirmation Dialog
The last scenario that I am going to cover is dedicated to the confirmation dialog.
Confirmation Dialog Handler Testing Framework
[TestMethod]
public void ConfirmDialogNotUniversal()
{
var dialog = new ConfirmDialog(manager.ActiveBrowser, DialogButton.OK);
manager.DialogMonitor.AddDialog(dialog);
manager.DialogMonitor.Start();
manager.ActiveBrowser.NavigateTo(
"http://www.w3schools.com/jsref/tryit.asp?filename=tryjsref_confirm");
Browser myFrame = manager.ActiveBrowser.Frames.ById("iframeResult");
HtmlButton alertButton = myFrame.Find.AllByTagName<HtmlButton>("button")[0];
alertButton.Click(false);
dialog.WaitUntilHandled();
manager.DialogMonitor.RemoveDialog(dialog);
}
Universal Confirmation Dialog Handler
We create a static ConfirmationDialogHandler class. You can even specify which dialog button to be clicked.
public static class ConfirmDialogHandler
{
public static void Handle(
System.Action action = null,
DialogButton dialogButton = DialogButton.OK)
{
ConfirmDialog confirmDialog =
new ConfirmDialog(Manager.Current.ActiveBrowser, dialogButton);
try
{
Manager.Current.DialogMonitor.AddDialog(confirmDialog);
Manager.Current.DialogMonitor.Start();
if (action != null)
{
action.Invoke();
}
confirmDialog.WaitUntilHandled();
confirmDialog.Handle();
}
finally
{
Manager.Current.DialogMonitor.RemoveDialog(confirmDialog);
Manager.Current.DialogMonitor.Stop();
}
}
}
The usage in tests is as simple as before.
[TestMethod]
public void ConfirmDialogUniversal()
{
manager.ActiveBrowser.NavigateTo(
"http://www.w3schools.com/jsref/tryit.asp?filename=tryjsref_confirm");
ConfirmDialogHandler.Handle(
() =>
{
Browser myFrame = manager.ActiveBrowser.Frames.ById("iframeResult");
HtmlButton alertButton =
myFrame.Find.AllByTagName<HtmlButton>("button")[0];
alertButton.Click(false);
});
}
You can find more code examples in the official framework's documentation.
Telerik Testing Framework Series
The post Revealed: How to Create Universal Dialog Handlers Testing Framework appeared first on Automate The Planet.
All images are purchased from DepositPhotos.com and cannot be downloaded and used for free.
License Agreement