Introduction
Many times, we manipulate HTML controls using JavaScript or JQuery code and don't know how strong ASP.NET MVC can be together with Razor and C# or don't know both ways to do it.
Below are these two ways to do it, the classic way with JQuery, that can also be used in PHP, JSP, etc.
Using the Code
This is a common multiple HTML select control:
@* Home\Index.cshtml *@
<select name='categories' id="selectCategories" multiple>
<option value="1">Meat</option>
<option value="2">Cereals</option>
<option value="3">Seafood</option>
<option value="4">Soda</option>
<option value="5">Coffee</option>
</select>
We need to select many options programmatically, for example: Meat
(1
), Cereals
(2
) and Soda
(4
).
Using JQuery
With JQuery, we need to create the JavaScript tag in the same HTML page or in JavaScript file and add the reference in the page, in this article, it has been used in the second way:
$(document).ready(function () {
selectItems();
});
function selectItems() {
var values = "1,2,4";
$.each(values.split(","), function (i, e) {
$("#selectCategories option[value='" + e + "']").prop("selected", true);
});
}
In this line, we have the options that need to be selected:
var values = "1,2,4";
Using Razor and C#
This is the Model class which we want load in HTML select control:
public class Category
{
public int Id { get; set; }
public string Name { get; set; }
}
The Controller
which allows to populate the select
control and select:
public ActionResult Index()
{
List<Category> categories = new List<Category>();
Category category = new Category();
category.Id = 1;
category.Name = "Meat";
categories.Add(category);
category = new Category();
category.Id = 2;
category.Name = "Cereals";
categories.Add(category);
category = new Category();
category.Id = 3;
category.Name = "Seafood";
categories.Add(category);
category = new Category();
category.Id = 4;
category.Name = "Soda";
categories.Add(category);
category = new Category();
category.Id = 5;
category.Name = "Coffee";
categories.Add(category);
ViewBag.Categories = new MultiSelectList(categories, "Id", "Name", new[] { 1, 2, 4 });
return View();
}
The MultiSelectList
object allows selected and pass the List
with all options to Home\Index.cshtml:
ViewBag.Categories = new MultiSelectList(categories, "Id", "Name", new[] { 1, 2, 4 });
In the Home\Index.cshtml, we can use an empty HTML select
control and populate with ViewBag.Categories
with loop sentence as for
or while
in Razor:
<select name='categories' id="selectCategories" multiple/>
Or use Razor HTML Helpers:
<h2>Multiselect with Razor (DropDownList)</h2>
@Html.DropDownList("Id", (MultiSelectList)ViewBag.Categories, new { multiple = "multiple" })
<h2>Multiselect with Razor (ListBox)</h2>
@Html.ListBox("Id", (MultiSelectList)ViewBag.Categories)
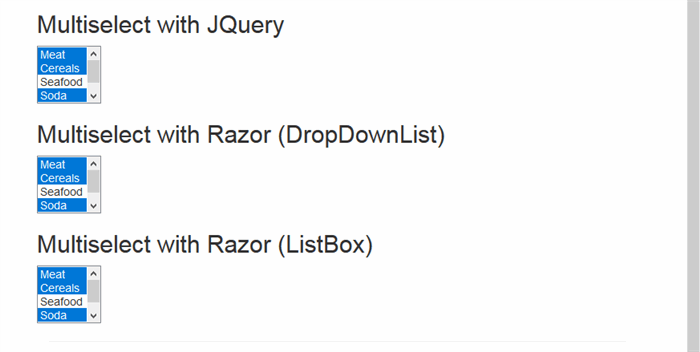
Points of Interest
Personally, I prefer Razor HTML Helpers, solve many HTML controls that lack functionalities and reduced code lines.
History
- 12th January, 2020: Initial submission
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.