Introduction
The GridView control has a variety of functionalities. In this article I will use it as a preview container.
Suppose you have an image folder in your web application and that image folder has pictures for a slideshow or picture gallery or slider, etcetera. You might need to add a new picture there or replace an existing picture. For that I have used a
GridView
control so that you can preview all the pictures in the images folder and replace them if required.
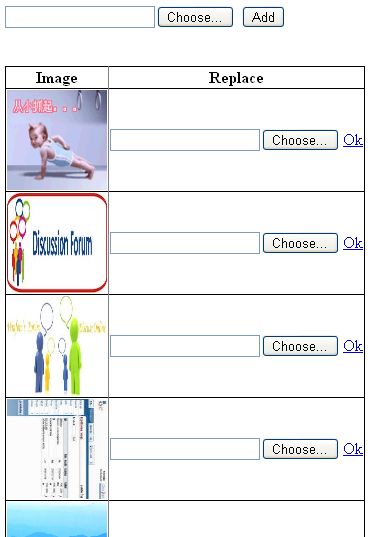
Of course you can also add a new image; for that there is a file upload control
ID. First of all, add an image folder to your web application as follows:
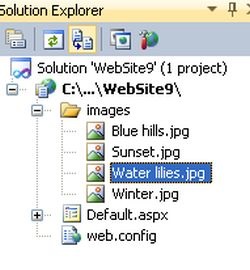
Controls on design
Now add a file-upload control and a button to add a new image. Add a GridView
control,
and your source code should look as follows in the source view:
<asp:FileUpload runat="server" ID="FupMain" />
<asp:Button ID="Button1" runat="server" onclick="Button1_Click"
Text="Add" />
<br />
<br />
<br />
<asp:GridView DataKeyNames="Name" AutoGenerateColumns="false" ID="GridView1"
DataSource='<%#dt %>' runat="server" onrowcommand="GridView1_RowCommand">
<Columns>
<asp:TemplateField HeaderText="Image">
<ItemTemplate>
<asp:Image ImageUrl='<%# "~/images/"+ Eval("Name") %>'
runat="server" ID="img2" Height="100"Width="100" />
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Replace">
<ItemTemplate>
<asp:FileUpload runat="server" ID="fup" />
<asp:LinkButton CommandName="replace" runat="server"Text="Ok" ID="btnRep" />
</ItemTemplate>
</asp:TemplateField>
</Columns>
</asp:GridView>
Note the following:
GridView
has a property, onrowcommand="GridView1_RowCommand"
.GridView
has two item-templates: one for the image control and another for the file-upload and link buttons.
Using Server Side Code
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data;
using System.IO;
public partial class _Default : System.Web.UI.Page
{
public string[] arr;
public DataTable dt;
public DataRow dr;
protected void Page_Load(object sender, EventArgs e)
{
arr = Directory.GetFiles(Server.MapPath("~\\") +
"images").Select(path => Path.GetFileName(path)).ToArray();
dt = new DataTable();
dt.Columns.Add("name");
for (int i = 0; i < arr.Length; i++)
{
dr = dt.NewRow();
dr["name"] = arr[i].ToString();
dt.Rows.Add(dr);
}
GridView1.DataBind();
}
protected void GridView1_RowCommand(object sender, GridViewCommandEventArgs e)
{
if (e.CommandName == "replace")
{
LinkButton btn = (LinkButton)e.CommandSource;
GridViewRow grow = (GridViewRow)btn.NamingContainer;
FileUpload fup1 = (FileUpload)grow.FindControl("fup");
string fileName = GridView1.DataKeys[grow.RowIndex].Value.ToString();
if (fup1.HasFile)
{
string path = Server.MapPath("~/images/") + fileName;
fup1.SaveAs(path);
}
}
}
protected void Button1_Click(object sender, EventArgs e)
{
if (FupMain.HasFile)
{
string path = Server.MapPath("~/images/")+FupMain.FileName;
FupMain.SaveAs(path);
}
}
}
History
- 20 Dec. 2013: Initial version.
He started programming in year 2005 and since he achieves MCTS, MCA and MCPD in .Net Platform.
He works as Team Leader in one MNC Company. He is also consultant software developer for both windows and web.
He always contribute technical belief on several technical websites.