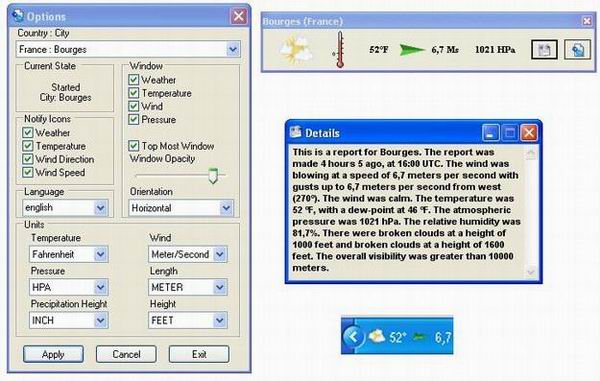
Introduction
This shows the weather in the status bar and/or in a window.
Details can be shown on another window. This is a multiple languages/units software (to add more languages, just put an XML file in the language directory). This is a C# translation of phpweather.
This shows a good example for XML serialization, the use of notifyicons, dynamic image loading, multiple language interface and regular expressions.
First we download information from weather.noaa.gov. Next we parse this information using regular expressions to retrieve full weather information: clouds, temperature, pressure, wind information, precipitation, visibility. This helps us to associate the correct image to the corresponding weather.
Content
This application just connects to weather.noaa.gov every hour to retrieve weather information. All the information is in a string like "TFFR 281000Z 00000KT 9999 FEW020 19/18 Q1011 NOSIG" called METAR. (Information on METAR decoding can be found here.)
So we just parse this string using regular expressions to find weather information (temperature, wind, pressure ...). After we retrieve all the required information, we just show the image associated with the weather conditions and make a report containing all this information.
Project contents:
- class
METAR
used for the parsing: only a big parsing of regular expressions.
ereg
: use of Microsoft regular expression functions to work like the PHP one's.
- class used to store decoded weather information: clouds, humidity, precipitation, runway, temp, visibility, weather, wind.
- class to easily convert values: wind, pressure, temperature, length, height, wind speed.
xml_access
: used for XML serialization for options and languages. It is used to save/restore object values in/from XML files.
tcp_socket
: class to retrieve data on the Internet (part of the network stuff project available on this website).
- class METAR download: launch download every hour or retry download 5 min after a failure.
- forms for user interface.
- other utility class (
file_access
, XP style).
Most important points:
The PHP ereg function emulation
We just try to make a similar function as that of PHP to avoid changing the parsing too much.
public class ClassEreg
{
public static bool ereg(string pattern,string input_string,
ref string[] strregs)
{
if (input_string=="")
return false;
if (!System.Text.RegularExpressions.Regex.IsMatch(input_string,
pattern,System.Text.RegularExpressions.RegexOptions.IgnoreCase))
return false;
System.Text.RegularExpressions.Match m
= System.Text.RegularExpressions.Regex.Match(input_string,
pattern,
System.Text.RegularExpressions.RegexOptions.IgnoreCase);
strregs=new string[m.Groups.Count];
for (int cpt=0;cpt<M.GROUPS.COUNT;CPT++)
{
if {m.Groups[cpt].Captures.Count >0)
strregs[cpt]=m.Groups[cpt].Captures[0].Value;
else
strregs[cpt]="";
}
return true;
}
public static bool ereg(string pattern,string input_string)
{
return System.Text.RegularExpressions.Regex.IsMatch(input_string,
pattern,
System.Text.RegularExpressions.RegexOptions.IgnoreCase);
}
}
These functions are called during all the parsing (the METAR
class is only a long boring parsing). Here's a sample usage:
string[] regs=null;
string part="010V090";
if (ClassEreg.ereg("^([0-9]{3})V([0-9]{3})$", part,ref regs))
{
this.wind.direction.var_beg = System.Convert.ToDouble(regs[1]);
this.wind.direction.var_end = System.Convert.ToDouble(regs[2]);
}
Dynamic image loading
We use a PictureBox
and its property PictureBox.Image
to show images in forms.
private System.Windows.Forms.PictureBox picturebox_weather;
public void set_img_weather(string fullpath)
{
Image img=get_image(fullpath);
if (img!=null)
this.picturebox_weather.Image=img;
}
private Image get_image(string fullpath)
{
try
{
return Image.FromFile(fullpath);
}
catch
{
MessageBox.Show( "File "+fullpath+" not found.","Error",
MessageBoxButtons.OK,MessageBoxIcon.Error);
return null;
}
}
Dynamic image loading for notify icon
private System.Windows.Forms.NotifyIcon notify_icon;
string img_path="image.png";
if (this.components==null)
this.components = new System.ComponentModel.Container();
notify_icon=new System.Windows.Forms.NotifyIcon(this.components);
notify_icon.Icon=System.Drawing.Icon.FromHandle(
this.GetThumb(new Bitmap(img_path),16,16).GetHicon());
Text for notify icon
private void drawstring_in_notify_icon(string text,
NotifyIcon notify_icon)
{
Bitmap bitmap = new Bitmap( 16, 16,
System.Drawing.Imaging.PixelFormat.Format32bppArgb);
Graphics graphics = Graphics.FromImage(bitmap);
System.Drawing.Font drawFont
= new System.Drawing.Font("Arial Narrow",8,
System.Drawing.FontStyle.Regular);
string drawString = text;
SizeF drawStringSize = new SizeF();
drawStringSize = graphics.MeasureString(drawString, drawFont);
System.Drawing.SolidBrush drawBrush
= new System.Drawing.SolidBrush(
Color.FromArgb( 255, 255, 255, 255));
graphics.DrawString(drawString, drawFont, drawBrush,
16/2-drawStringSize.Width/2,16/2-drawStringSize.Height/2);
notify_icon.Icon=System.Drawing.Icon.FromHandle(bitmap.GetHicon());
drawFont.Dispose();
drawBrush.Dispose();
graphics.Dispose();
}
XML loading/saving
The trick is the same for options, language and language interface XML files. We just use XML serialization. The following sample is for the options class:
public static ClassOptions load(string config_file_name)
{
try
{
return (ClassOptions)
XML_access.XMLDeserializeObject(config_file_name,
typeof(ClassOptions));
}
catch
{
return new ClassOptions();
}
}
public bool save(string config_file_name)
{
try
{
XML_access.XMLSerializeObject(config_file_name,this,
typeof(ClassOptions));
return true;
}
catch (Exception e)
{
System.Windows.Forms.MessageBox.Show(e.Message,"Error",
System.Windows.Forms.MessageBoxButtons.OK,
System.Windows.Forms.MessageBoxIcon.Error);
return false;
}
}
Where the XML serialization class is the following:
using System;
using System.IO;
using System.Text;
using System.Xml;
using System.Xml.Serialization;
public class XML_access
{
public static void XMLSerializeObject(string filename,object obj,
System.Type typeof_object)
{
Stream fs=null;
try
{
XmlSerializer serializer = new XmlSerializer(typeof_object);
fs = new FileStream(filename, FileMode.Create,
System.IO.FileAccess.ReadWrite );
XmlWriter writer = new XmlTextWriter(fs,
System.Text.Encoding.Unicode);
serializer.Serialize(writer, obj);
writer.Close();
fs.Close();
}
catch(Exception e)
{
if (fs!=null)
fs.Close();
System.Windows.Forms.MessageBox.Show( e.Message,
"Error",
System.Windows.Forms.MessageBoxButtons.OK,
System.Windows.Forms.MessageBoxIcon.Error);
}
}
public static object XMLDeserializeObject(string filename,
System.Type typeof_object)
{
XmlSerializer serializer = new XmlSerializer(typeof_object);
FileStream fs = new FileStream(filename, FileMode.Open);
XmlReader reader = new XmlTextReader(fs);
object obj;
obj = serializer.Deserialize(reader);
reader.Close();
fs.Close();
return obj;
}
}